
CMSIS-DAP debug adapter
Dependencies: BaseDAP SWD USBDAP USBDevice mbed
Fork of Simple-CMSIS-DAP by
main.cpp
00001 #include "mbed.h" 00002 #include "USBDAP.h" 00003 #include "BaseDAP.h" 00004 00005 #if defined(TARGET_MCU_LPC11U35_501) 00006 SWD swd(p6, p8, p30); // SWDIO,SWCLK,nRESET 00007 DigitalOut connected(LED1); 00008 DigitalOut running(LED2); 00009 #else 00010 SWD swd(D12, D10, D6); // SWDIO,SWCLK,nRESET 00011 DigitalOut connected(LED_GREEN); 00012 DigitalOut running(LED_RED); 00013 #endif 00014 00015 class myDAP : public BaseDAP { 00016 public: 00017 myDAP(SWD* swd):BaseDAP(swd){}; 00018 virtual void infoLED(int select, int value) { 00019 switch(select) { 00020 case 0: 00021 connected = value^1; 00022 running = 1; 00023 break; 00024 case 1: 00025 running = value^1; 00026 connected = 1; 00027 break; 00028 } 00029 } 00030 }; 00031 00032 int main() { 00033 USBDAP* hid = new USBDAP("CMSIS-DAP"); 00034 myDAP* dap = new myDAP(&swd); 00035 while(1) { 00036 HID_REPORT recv_report; 00037 if(hid->readNB(&recv_report)) { 00038 HID_REPORT send_report; 00039 dap->Command(recv_report.data, send_report.data); 00040 send_report.length = 64; 00041 hid->send(&send_report); 00042 } 00043 } 00044 } 00045
Generated on Sun Jul 24 2022 02:29:22 by
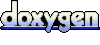