Simple USBHost library for LPC4088. Backward compatibility of official-USBHost.
Dependencies: FATFileSystem mbed-rtos
USBHost Class Reference
USBHost class This class is a singleton. More...
#include <USBHost.h>
Inherits USBHALHost.
Public Member Functions | |
USB_TYPE | controlRead (USBDeviceConnected *dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t *buf, uint32_t len) |
Control read: setup stage, data stage and status stage. | |
USB_TYPE | controlWrite (USBDeviceConnected *dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t *buf, uint32_t len) |
Control write: setup stage, data stage and status stage. | |
USB_TYPE | bulkRead (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Bulk read. | |
USB_TYPE | bulkWrite (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Bulk write. | |
USB_TYPE | interruptRead (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Interrupt read. | |
USB_TYPE | interruptWrite (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Interrupt write. | |
USB_TYPE | enumerate (USBDeviceConnected *dev, IUSBEnumerator *pEnumerator) |
Enumerate a device. | |
USBDeviceConnected * | getDevice (uint8_t index) |
Get a device. | |
template<typename T > | |
void | registerDriver (USBDeviceConnected *dev, uint8_t intf, T *tptr, void(T::*mptr)(void)) |
register a driver into the host associated with a callback function called when the device is disconnected | |
Static Public Member Functions | |
static USBHost * | getHostInst () |
Static method to create or retrieve the single USBHost instance. |
Detailed Description
USBHost class This class is a singleton.
All drivers have a reference on the static USBHost instance
Definition at line 42 of file USBHost.h.
Member Function Documentation
USB_TYPE bulkRead | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Bulk read.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to read a packet buf pointer on a buffer where will be store the data received len length of the transfer blocking if true, the read is blocking (wait for completion)
- Returns:
- status of the bulk read
Definition at line 251 of file USBHost.cpp.
USB_TYPE bulkWrite | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Bulk write.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the write is blocking (wait for completion)
- Returns:
- status of the bulk write
Definition at line 266 of file USBHost.cpp.
USB_TYPE controlRead | ( | USBDeviceConnected * | dev, |
uint8_t | requestType, | ||
uint8_t | request, | ||
uint32_t | value, | ||
uint32_t | index, | ||
uint8_t * | buf, | ||
uint32_t | len | ||
) |
Control read: setup stage, data stage and status stage.
- Parameters:
-
dev the control read will be done for this device requestType request type request request value value index index buf pointer on a buffer where will be store the data received len length of the transfer
- Returns:
- status of the control read
Definition at line 194 of file USBHost.cpp.
USB_TYPE controlWrite | ( | USBDeviceConnected * | dev, |
uint8_t | requestType, | ||
uint8_t | request, | ||
uint32_t | value, | ||
uint32_t | index, | ||
uint8_t * | buf, | ||
uint32_t | len | ||
) |
Control write: setup stage, data stage and status stage.
- Parameters:
-
dev the control write will be done for this device requestType request type request request value value index index buf pointer on a buffer which will be written len length of the transfer
- Returns:
- status of the control write
Definition at line 199 of file USBHost.cpp.
USB_TYPE enumerate | ( | USBDeviceConnected * | dev, |
IUSBEnumerator * | pEnumerator | ||
) |
Enumerate a device.
- Parameters:
-
dev device which will be enumerated
- Returns:
- status of the enumeration
Definition at line 91 of file USBHost.cpp.
USBDeviceConnected* getDevice | ( | uint8_t | index ) |
USBHost * getHostInst | ( | ) | [static] |
Static method to create or retrieve the single USBHost instance.
Definition at line 25 of file USBHost.cpp.
USB_TYPE interruptRead | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Interrupt read.
- Parameters:
-
dev the interrupt transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the read is blocking (wait for completion)
- Returns:
- status of the interrupt read
Definition at line 276 of file USBHost.cpp.
USB_TYPE interruptWrite | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Interrupt write.
- Parameters:
-
dev the interrupt transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the write is blocking (wait for completion)
- Returns:
- status of the interrupt write
void registerDriver | ( | USBDeviceConnected * | dev, |
uint8_t | intf, | ||
T * | tptr, | ||
void(T::*)(void) | mptr | ||
) |
Generated on Tue Jul 12 2022 22:44:17 by
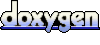