Simple USBHost library for LPC4088. Backward compatibility of official-USBHost.
Dependencies: FATFileSystem mbed-rtos
USBEndpoint.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #pragma once 00018 #include "rtos.h" 00019 #include "FunctionPointer.h" 00020 #include "USBHostTypes.h" 00021 #include "USBDeviceConnected.h" 00022 00023 class USBDeviceConnected; 00024 00025 #define HCTD_QUEUE_SIZE 3 00026 00027 struct HCTD; 00028 struct HCED; 00029 struct HCITD; 00030 class USBHost; 00031 00032 /** 00033 * USBEndpoint class 00034 */ 00035 class USBEndpoint { 00036 public: 00037 /** 00038 * Constructor 00039 */ 00040 USBEndpoint(USBDeviceConnected* _dev) { 00041 init(CONTROL_ENDPOINT, IN, 8, 0); 00042 dev = _dev; 00043 } 00044 00045 /** 00046 * Initialize an endpoint 00047 * 00048 * @param type endpoint type 00049 * @param dir endpoint direction 00050 * @param size endpoint size 00051 * @param ep_number endpoint number 00052 */ 00053 void init(ENDPOINT_TYPE _type, ENDPOINT_DIRECTION _dir, uint32_t size, uint8_t ep_number) { 00054 setState(USB_TYPE_FREE); 00055 setType(_type); 00056 dir = _dir; 00057 MaxPacketSize = size; 00058 address = ep_number; 00059 m_pED = NULL; 00060 //data01_toggle = DATA0; // for KL46Z 00061 } 00062 00063 /** 00064 * Attach a member function to call when a transfer is finished 00065 * 00066 * @param tptr pointer to the object to call the member function on 00067 * @param mptr pointer to the member function to be called 00068 */ 00069 template<typename T> 00070 void attach(T* tptr, void (T::*mptr)(void)) { 00071 if((mptr != NULL) && (tptr != NULL)) { 00072 rx.attach(tptr, mptr); 00073 } 00074 } 00075 00076 /** 00077 * Attach a callback called when a transfer is finished 00078 * 00079 * @param fptr function pointer 00080 */ 00081 void attach(void (*fptr)(void)) { 00082 if(fptr != NULL) { 00083 rx.attach(fptr); 00084 } 00085 } 00086 00087 /** 00088 * Call the handler associted to the end of a transfer 00089 */ 00090 void call() { 00091 rx.call(); 00092 }; 00093 00094 void irqWdhHandler(HCTD* td) {m_queue.put(td);} // WDH 00095 HCTD* get_queue_HCTD(uint32_t millisec=osWaitForever); 00096 HCED* m_pED; 00097 // report 00098 uint8_t m_ConditionCode; 00099 int m_report_queue_error; 00100 00101 void setType(ENDPOINT_TYPE _type) { type = _type; }; 00102 void setState(USB_TYPE st){ state = st; }; 00103 void setLengthTransferred(int len) { transferred = len; }; 00104 void setBuffer(uint8_t* buf, int size) { buf_start = buf, buf_size = size; } 00105 void setSize(int size) { MaxPacketSize = size; } 00106 void setNextEndpoint(USBEndpoint* ep) { nextEp = ep; }; 00107 00108 USBDeviceConnected* getDevice() { return dev; } 00109 ENDPOINT_TYPE getType() { return type; }; 00110 USB_TYPE getState() { return state; } 00111 int getLengthTransferred() { return transferred; } 00112 uint8_t *getBufStart() { return buf_start; } 00113 int getBufSize() { return buf_size; } 00114 uint8_t getAddress(){ return address; }; 00115 int getSize() { return MaxPacketSize; } 00116 ENDPOINT_DIRECTION getDir() { return dir; } 00117 USBEndpoint* nextEndpoint() { return nextEp; }; 00118 00119 private: 00120 ENDPOINT_TYPE type; 00121 USB_TYPE state; 00122 ENDPOINT_DIRECTION dir; 00123 USBDeviceConnected* dev; 00124 uint8_t address; 00125 int transferred; 00126 uint8_t * buf_start; 00127 int buf_size; 00128 FunctionPointer rx; 00129 int MaxPacketSize; 00130 USBEndpoint* nextEp; 00131 00132 protected: 00133 Queue<HCTD, HCTD_QUEUE_SIZE> m_queue; // TD done queue 00134 int m_td_queue_count; 00135 }; 00136 00137 class EndpointQueue { 00138 public: 00139 EndpointQueue():head(NULL),tail(NULL) {} 00140 void push(USBEndpoint* ep) { 00141 if (head) { 00142 tail->setNextEndpoint(ep); 00143 } else { 00144 head = ep; 00145 } 00146 tail = ep; 00147 ep->setNextEndpoint(NULL); 00148 } 00149 USBEndpoint* pop() { 00150 USBEndpoint* ep = head; 00151 if (ep) { 00152 head = ep->nextEndpoint(); 00153 } 00154 return ep; 00155 } 00156 bool empty() { return head == NULL; } 00157 00158 private: 00159 USBEndpoint* head; 00160 USBEndpoint* tail; 00161 }; 00162 00163
Generated on Tue Jul 12 2022 22:44:17 by
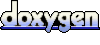