
Simple USBHost Mouse for FRDM-KL46Z test program
Dependencies: KL46Z-USBHost mbed
main.cpp
00001 // Simple USBHost Mouse for FRDM-KL46Z test program 00002 00003 #include "USBHostMouse.h" 00004 00005 DigitalOut led1(LED_GREEN); 00006 DigitalOut led2(LED_RED); 00007 #define LED_OFF 1 00008 #define LED_ON 0 00009 00010 void callback(uint8_t buttons) { 00011 led1 = (buttons&1) ? LED_ON : LED_OFF; // button on/off 00012 led2 = (buttons&2) ? LED_ON : LED_OFF; 00013 printf("%02x\n", buttons); 00014 } 00015 00016 int main() { 00017 USBHostMouse mouse; 00018 if (!mouse.connect()) { 00019 error("USB mouse not found.\n"); 00020 } 00021 mouse.attachButtonEvent(callback); 00022 while(1) { 00023 USBHost::poll(); 00024 } 00025 }
Generated on Mon Aug 1 2022 00:49:01 by
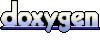