Graphic OLED 100x16 pixels interface
Dependents: mbed_nicovideo_search_api mbed_recent_nicovideo_display_pub
GraphicOLED.h
00001 // GraphicOLED.h 00002 #pragma once 00003 #include "mbed.h" 00004 00005 /** GraphicOLED interface for WS0010 00006 * 00007 * Currently support UTF-8 KANJI(misaki font) 00008 * 00009 * @code 00010 * #include "mbed.h" 00011 * #include "GraphicOLED.h" 00012 * 00013 * GraphicOLED oled(p24, p26, p27, p28, p29, p30); // rs, e, d4-d7 00014 * 00015 * int main() { 00016 * oled.printf("Hello World!\n"); 00017 * } 00018 * @endcode 00019 */ 00020 class GraphicOLED : public Stream { 00021 public: 00022 /** Create a GraphicOLED interface 00023 * 00024 * @param rs Instruction/data control line 00025 * @param e Enable line (clock) 00026 * @param d4-d7 Data lines for using as a 4-bit interface 00027 */ 00028 GraphicOLED(PinName rs, PinName e, PinName d4, PinName d5, PinName d6, PinName d7); 00029 00030 #if DOXYGEN_ONLY 00031 /** Write a character to the OLED 00032 * 00033 * @param c The character to write to the display 00034 */ 00035 int putc(int c); 00036 00037 /** Write a formated string to the OLED 00038 * 00039 * @param format A printf-style format string, followed by the 00040 * variables to use in formating the string. 00041 */ 00042 int printf(const char* format, ...); 00043 #endif 00044 00045 /** Locate to a screen column and row 00046 * 00047 * @param column The horizontal position from the left, indexed from 0 00048 * @param row The vertical position from the top, indexed from 0 00049 */ 00050 void locate(int column, int row); 00051 00052 /** Clear the screen and locate to 0,0 */ 00053 void cls(); 00054 00055 int rows(); 00056 int columns(); 00057 00058 void g_write(uint8_t pat, int x, int y); 00059 void g_write(uint8_t *buf, int len, int x, int y); 00060 00061 private: 00062 int _uni_len; 00063 uint32_t _unicode; 00064 00065 // Stream implementation functions 00066 virtual int _putc(int value); 00067 virtual int _getc(); 00068 00069 int character(int column, int row, int c); 00070 void writeByte(uint8_t value); 00071 void writeCommand(uint8_t command); 00072 void writeData(uint8_t data); 00073 00074 DigitalOut _rs, _e; 00075 BusOut _d; 00076 00077 int _column; 00078 int _row; 00079 }; 00080
Generated on Wed Jul 13 2022 09:52:33 by
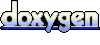