huffmancode to decode in real-time for motion-jpeg
Dependents: BaseJpegDecode_example SimpleJpegDecode_example Dumb_box_rev2
inverseDCT.cpp
00001 #include "mbed.h" 00002 #include "inverseDCT.h" 00003 00004 #ifdef USE_IDCT_AAN 00005 const int zigzag[64] = 00006 {0, 00007 1, 8, 00008 16, 9, 2, 00009 3,10,17,24, 00010 32,25,18,11, 4, 00011 5,12,19,26,33,40, 00012 48,41,34,27,20,13,6, 00013 7,14,21,28,35,42,49,56, 00014 57,50,43,36,29,22,15, 00015 23,30,37,44,51,58, 00016 59,52,45,38,31, 00017 39,46,53,60, 00018 61,54,47, 00019 55,62, 00020 63}; 00021 00022 void inverseDCT::inputBLOCK(int mcu, int block, int scan, int value) { 00023 if (scan == 0) { 00024 for(int i = 0; i < 64; i++) { 00025 m_s[i] = 0; 00026 } 00027 } 00028 m_s[zigzag[scan]] = value; 00029 if (scan == 63) { // last 00030 idct.conv(reinterpret_cast<int8_t*>(m_s), m_s); 00031 outputBLOCK(mcu, block, reinterpret_cast<int8_t*>(m_s)); 00032 } 00033 }; 00034 #endif //USE_IDCT_AAN 00035 00036 #ifdef USE_IDCT_TABLE 00037 #include "inverseDCT_table.h" 00038 void inverseDCT::inputBLOCK(int mcu, int block, int scan, int value) 00039 { 00040 if (scan == 0) { 00041 int t = value * 32 / 8; 00042 for(int i = 0; i < 64; i++) { 00043 m_sum[i] = t; 00044 } 00045 return; 00046 } 00047 00048 if (value != 0) { 00049 for(int xy = 0; xy < 64; xy++) { 00050 m_sum[xy] += idct_table[scan*64+xy] * value / 16; 00051 } 00052 } 00053 00054 if (scan == 63) { 00055 int8_t* result = reinterpret_cast<int8_t*>(m_sum); 00056 for(int i = 0; i < 64; i++) { 00057 int t = m_sum[i] / 32; 00058 if (t > 127) { 00059 t = 127; 00060 } else if (t < -128) { 00061 t = -128; 00062 } 00063 result[i] = t; 00064 } 00065 outputBLOCK(mcu, block, result); 00066 } 00067 } 00068 #endif //USE_IDCT_TABLE
Generated on Thu Jul 14 2022 18:10:57 by
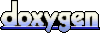