
Sistema de control de un cooler a lazo abierto y cerrado
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "Dht11.h" 00003 00004 //TICKERS CON FUNCIONES Y VARIABLES QUE SE USAN CON EL 00005 Ticker sec; 00006 void segundo(); 00007 int mandar = 0; 00008 int contador = 0; 00009 00010 //SENSOR DE TEMPERATURA 00011 Dht11 sensor(D2); 00012 int temperatura = 0; 00013 00014 //ENTRADA DEL SENSOR DEL MOTOR 00015 DigitalIn rpm(D3); 00016 00017 //SALIDA PWM PARA LA INTERFAZ DEL MOTOR 00018 PwmOut motor(D4); 00019 00020 //ENTRADA ANALOGICA PARA EL PRESET 00021 AnalogIn preset(A0); 00022 00023 //BOTON DE RESTART 00024 Ticker boton; 00025 DigitalIn restartButton(PTB8); 00026 void button_in(); 00027 unsigned char state1 = 0x00; 00028 int button_output = 0; 00029 00030 //MAQUINA DE ESTADOS CUENTA DE VUELTAS 00031 void ME_RPM(); 00032 enum ME_RPM_ESTADOS { ESPERO_FA, ESPERO_FD }; 00033 ME_RPM_ESTADOS ME_RPM_ESTADO; 00034 int cuenta_vueltas = 0; 00035 00036 //MAQUINA DE ESTADOS GENERAL 00037 void ME_GENERAL(); 00038 enum ME_GENERAL_ESTADOS { LAZO_A, LAZO_C }; 00039 ME_GENERAL_ESTADOS ME_GENERAL_ESTADO; 00040 00041 //VARIABLE QUE GUARDA EL NIVEL DE DUTY QUE CALCULA EL PROGRAMA 00042 float duty = 0; 00043 00044 int main() 00045 { 00046 //INICIO EL MOTOR PRENDIDO AL MAXIMO PARA QUE SE LE PUEDA BAJAR EL DUTY POSTERIORMENTE 00047 00048 motor = 1.0; 00049 wait(2); 00050 00051 //PULLUP PARA LA ENTRADA DEL BOTON DE CAMBIO DE MODO 00052 restartButton.mode(PullUp); 00053 00054 //TICKERS 00055 sec.attach(&segundo, 1); 00056 boton.attach(&button_in, 0.01); 00057 00058 //ESTADO INICIAL MAQUINAS DE ESTADO 00059 ME_RPM_ESTADO = ESPERO_FA; 00060 ME_GENERAL_ESTADO = LAZO_A; 00061 00062 while(1) { 00063 ME_RPM(); 00064 ME_GENERAL(); 00065 00066 //CALCULO DE DUTY PARA CADA LAZO 00067 if(ME_GENERAL_ESTADO == LAZO_A) { 00068 if(preset < 0.035) { 00069 duty = 0.035; // 200 RPM - 0.035 00070 } else { 00071 duty = preset; 00072 } 00073 } else { 00074 duty = temperatura < 20 ? 0.035 : temperatura / 70.0; 00075 } 00076 00077 //ENVIO DE DATOS A LA PC 00078 if(mandar) { 00079 printf("T: %d, Modo: %d\r\n", sensor.getCelsius(), ME_GENERAL_ESTADO); 00080 printf("RPM: %d Duty: %f\r\n", cuenta_vueltas * 60, duty * 100); 00081 printf("Boton: %d\n\r", button_output); 00082 cuenta_vueltas = 0; 00083 mandar = 0; 00084 motor = duty; 00085 } 00086 } 00087 } 00088 00089 //TICKER 1 seg 00090 void segundo() 00091 { 00092 //FLAG DE ENVIO DE DATOS A LA PC 00093 mandar = 1; 00094 00095 //LECTURA DE LA TEMEPERATURA DEL SENSOR 00096 sensor.read(); 00097 temperatura = sensor.getCelsius(); 00098 00099 //CONTADOR 1 seg 00100 contador++; 00101 } 00102 00103 void ME_RPM() 00104 { 00105 switch(ME_RPM_ESTADO) { 00106 case ESPERO_FA: 00107 //SI SE DETECTA UN FLANCO ASCENDENTE CAMBIO DE MODO 00108 if(rpm) { 00109 ME_RPM_ESTADO = ESPERO_FD; 00110 cuenta_vueltas++; 00111 } 00112 break; 00113 case ESPERO_FD: 00114 //SI SE DETECTA UN FLANCO DESCENDENTE CAMBIO DE MODO 00115 if(!rpm) { 00116 ME_RPM_ESTADO = ESPERO_FA; 00117 } 00118 break; 00119 } 00120 00121 } 00122 00123 void ME_GENERAL() 00124 { 00125 switch(ME_GENERAL_ESTADO) { 00126 case LAZO_A: 00127 //SI SE APRIETA EL BOTON Y YA PASO UN SEGUNDO DESDE EL ULTIMO CAMBIO DE MODO SE PRODUCE UN CAMBIO 00128 if(button_output == 1 && contador > 1) { 00129 ME_GENERAL_ESTADO = LAZO_C; 00130 contador = 0; 00131 } 00132 break; 00133 case LAZO_C: 00134 //SI SE APRIETA EL BOTON Y YA PASO UN SEGUNDO DESDE EL ULTIMO CAMBIO DE MODO SE PRODUCE UN CAMBIO 00135 if(button_output == 1 && contador > 1) { 00136 ME_GENERAL_ESTADO = LAZO_A; 00137 contador = 0; 00138 } 00139 break; 00140 } 00141 } 00142 00143 //MUESTREO DEL BOTON PARA ELIMINACION DEL REBOTE 00144 void button_in() 00145 { 00146 state1 = state1 >> 1; 00147 00148 if(!restartButton) { 00149 state1 |= 0x80; 00150 } 00151 if(state1 == 0xFF) 00152 button_output = 1; 00153 else if(state1 == 0) 00154 button_output = 0; 00155 }
Generated on Wed Jul 20 2022 10:42:22 by
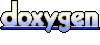