Pressure, Temperature, Altitude Sensor on breakout from sparkfun (I2C)
Embed:
(wiki syntax)
Show/hide line numbers
BMP085.h
00001 /* 00002 * @file BMP085.h 00003 * @author Tyler Weaver 00004 * @author Kory Hill 00005 * 00006 * @section LICENSE 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00009 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00010 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00011 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in all copies or 00015 * substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00018 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00020 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00022 * 00023 * @section DESCRIPTION 00024 * 00025 * BMP085 I2C Temperature/Pressure/Altitude Sensor 00026 * 00027 * Max sample rate: 128 samples/second (temperature at 1/second) 00028 * 00029 * Datasheet: 00030 * 00031 * http://dlnmh9ip6v2uc.cloudfront.net/datasheets/Sensors/Pressure/BST-BMP085-DS000-06.pdf 00032 */ 00033 00034 #ifndef BMP085_H 00035 #define BMP085_H 00036 00037 #include "mbed.h" 00038 00039 class BMP085 00040 { 00041 public: 00042 /** 00043 * The I2C address that can be passed directly to i2c object (it's already shifted 1 bit left). 00044 */ 00045 static const int16_t I2C_ADDRESS = 0xEE; //address of bmp085 00046 00047 /** 00048 * Constructor. 00049 * 00050 * Calls init function 00051 * 00052 * @param sda - mbed pin to use for the SDA I2C line. 00053 * @param scl - mbed pin to use for the SCL I2C line. 00054 */ 00055 BMP085(PinName sda, PinName scl); 00056 00057 /** 00058 * Constructor that accepts external i2c interface object. 00059 * 00060 * Calls init function 00061 * 00062 * @param i2c The I2C interface object to use. 00063 */ 00064 BMP085(I2C &i2c) : i2c_(i2c) { 00065 init(); 00066 } 00067 00068 ~BMP085(); 00069 00070 /** 00071 * Sets the oss rate variables 00072 * Acceptable values = 1,2,4,8 00073 * 00074 *@param oss the number of over sampling 00075 */ 00076 void set_oss(int oss); 00077 00078 int32_t get_temperature(); 00079 int32_t get_pressure(); 00080 double get_altitude_m(); 00081 double get_altitude_ft(); 00082 00083 /** 00084 * Initialize sensor and get calibration values 00085 */ 00086 void init(); 00087 00088 void display_cal_param(Serial *pc); 00089 00090 protected: 00091 00092 00093 private: 00094 00095 I2C &i2c_; 00096 00097 /** 00098 * The raw buffer for allocating I2C object in its own without heap memory. 00099 */ 00100 char i2cRaw[sizeof(I2C)]; 00101 00102 // calculation variables 00103 int16_t AC1, AC2, AC3, B1, B2, MB, MC, MD; 00104 uint16_t AC4, AC5, AC6; 00105 00106 int32_t UT,UP; // uncompressed temperature and pressure value 00107 00108 int32_t X1, X2, B5, temperature; // get_temperature variables 00109 00110 int32_t B6, B3, X3, pressure; // get_pressure variables 00111 uint32_t B4, B7; 00112 00113 double altitude; 00114 00115 // setting variables 00116 char oss_bit_; 00117 char oversampling_setting_; 00118 float conversion_time_; 00119 00120 void get_cal_param(); // get calibration parameters 00121 void get_ut(); // get uncompressed temperature 00122 void get_up(); // get uncompressed pressure 00123 void write_char(char,char); 00124 int16_t read_int16(char); 00125 uint16_t read_uint16(char); 00126 void read_multiple(char, char*, int); 00127 }; 00128 00129 #endif
Generated on Wed Jul 13 2022 10:31:14 by
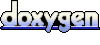