AK8963
Embed:
(wiki syntax)
Show/hide line numbers
AK8963.h
00001 // I2Cdev library collection - AK8963 I2C device class header file 00002 // Based on AKM AK8963/B datasheet, 12/2009 00003 // 8/27/2011 by Jeff Rowberg <jeff@rowberg.net> modified by Merlin 6-14-14 for 8963 00004 // Updates should (hopefully) always be available at https://github.com/jrowberg/i2cdevlib 00005 // 00006 // Changelog: 00007 // 2011-08-27 - initial release 00008 // 2014-06-14 - modified for AK8963 based on Kris Wieners MPU-9250 Sketch 00009 /* ============================================ 00010 I2Cdev device library code is placed under the MIT license 00011 Copyright (c) 2011 Jeff Rowberg 00012 00013 Permission is hereby granted, free of charge, to any person obtaining a copy 00014 of this software and associated documentation files (the "Software"), to deal 00015 in the Software without restriction, including without limitation the rights 00016 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00017 copies of the Software, and to permit persons to whom the Software is 00018 furnished to do so, subject to the following conditions: 00019 00020 The above copyright notice and this permission notice shall be included in 00021 all copies or substantial portions of the Software. 00022 00023 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00024 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00025 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00026 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00027 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00028 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00029 THE SOFTWARE. 00030 =============================================== 00031 */ 00032 00033 #ifndef _AK8963_H_ 00034 #define _AK8963_H_ 00035 00036 #include "mbed.h" 00037 00038 #ifndef I2C_SDA 00039 #define I2C_SDA p9 00040 #define I2C_SCL p10 00041 #endif 00042 00043 #include "I2CdevAK8963.h" 00044 00045 #define AK8963_ADDRESS_00 0x0C 00046 #define AK8963_ADDRESS_01 0x0D 00047 #define AK8963_ADDRESS_10 0x0E // default for InvenSense MPU-6050 evaluation board 00048 #define AK8963_ADDRESS_11 0x0F 00049 #define AK8963_DEFAULT_ADDRESS AK8963_ADDRESS_00 00050 00051 #define AK8963_RA_WIA 0x00 00052 #define AK8963_RA_INFO 0x01 00053 #define AK8963_RA_ST1 0x02 00054 #define AK8963_RA_HXL 0x03 00055 #define AK8963_RA_HXH 0x04 00056 #define AK8963_RA_HYL 0x05 00057 #define AK8963_RA_HYH 0x06 00058 #define AK8963_RA_HZL 0x07 00059 #define AK8963_RA_HZH 0x08 00060 #define AK8963_RA_ST2 0x09 00061 #define AK8963_RA_CNTL1 0x0A 00062 #define AK8963_RA_CNTL2 0x0B 00063 #define AK8963_RA_ASTC 0x0C 00064 #define AK8963_RA_TS1 0x0D // SHIPMENT TEST, DO NOT USE 00065 #define AK8963_RA_TS2 0x0E // SHIPMENT TEST, DO NOT USE 00066 #define AK8963_RA_I2CDIS 0x0F 00067 #define AK8963_RA_ASAX 0x10 00068 #define AK8963_RA_ASAY 0x11 00069 #define AK8963_RA_ASAZ 0x12 00070 00071 #define AK8963_ST1_DRDY_BIT 0 //Data ready bit 00072 #define AK8963_ST1_DOR_BIT 1 //Data overrun bit 00073 #define AK8963_ST2_HOFL_BIT 3 //Mag sensor overflow 00074 //#define AK8963_ST2_DERR_BIT 2 00075 #define AK8963_ST2_BITM 3 //Output bit, 0 = 14 bit, 1 = 16 bit 00076 00077 #define AK8963_CNTL1_MODE_BIT 3 00078 #define AK8963_CNTL1_MODE_LENGTH 4 00079 #define AK8963_MODE_POWERDOWN 0x0 00080 #define AK8963_MODE_SINGLE 0x1 00081 #define AK8963_MODE_CONT1 0x2 //Continuous mode 1 00082 #define AK8963_MODE_CONT2 0x6 //Continuous mode 2 00083 #define AK8963_MODE_EXT 0x4 //External Trigger mode 1 00084 #define AK8963_MODE_SELFTEST 0x8 00085 #define AK8963_MODE_FUSEROM 0xF 00086 00087 #define AK8963_CNTL1_OUT_BIT 4 00088 #define AK8963_CNTL1_OUT_LENGTH 1 //Output bit, 0 = 14 bit, 1 = 16 bit 00089 00090 #define AK8963_CNTL2_RESET 0x01 00091 00092 #define AK8963_ASTC_SELF_BIT 6 00093 00094 #define AK8963_I2CDIS_BIT 0 00095 00096 class AK8963 { 00097 public: 00098 AK8963(); 00099 AK8963(bool useSPI, uint8_t address); 00100 00101 void initialize(); 00102 bool testConnection(); 00103 00104 // WIA register 00105 uint8_t getDeviceID(); 00106 00107 // INFO register 00108 uint8_t getInfo(); 00109 00110 // ST1 register 00111 bool getDataReady(); 00112 bool getDataOverRun(); 00113 00114 // H* registers 00115 void getHeading(int16_t *x, int16_t *y, int16_t *z); 00116 00117 // ST2 register 00118 bool getOverflowStatus(); 00119 bool getOutBitST(); 00120 00121 // CNTL register 00122 uint8_t getMode(); 00123 void setModeRes(uint8_t mode, uint8_t Mscale); 00124 void reset(); 00125 00126 // ASTC register 00127 void setSelfTest(bool enabled); 00128 void getSelfTest(int16_t *x, int16_t *y, int16_t *z); 00129 00130 // I2CDIS 00131 void disableI2C(); // um, why...? 00132 00133 // ASA* registers 00134 void getAdjustment(uint8_t *x, uint8_t *y, uint8_t *z); 00135 00136 00137 private: 00138 bool bSPI; 00139 uint8_t devAddr; 00140 uint8_t buffer[14]; 00141 uint8_t mode; 00142 uint8_t Mscale; 00143 00144 AK8963I2C::I2Cdev i2Cdev; 00145 }; 00146 00147 #endif /* _AK8963_H_ */
Generated on Wed Jul 13 2022 20:54:49 by
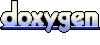