
ICRS Eurobot 2013
Dependencies: mbed mbed-rtos Servo QEI
Identity.h
00001 /* 00002 * $Id: Identity.h,v 1.4 2006-11-21 18:43:09 opetzold Exp $ 00003 */ 00004 00005 #ifndef TVMET_XPR_IDENTITY_H 00006 #define TVMET_XPR_IDENTITY_H 00007 00008 00009 namespace tvmet { 00010 00011 00012 /** 00013 * \class XprIdentity Identity.h "tvmet/xpr/Identity.h" 00014 * \brief Expression for the identity matrix. 00015 * 00016 * This expression doesn't hold any other expression, it 00017 * simply returns 1 or 0 depends where the row and column 00018 * element excess is done. 00019 * 00020 * \since release 1.6.0 00021 * \sa identity 00022 */ 00023 template<class T, std::size_t Rows, std::size_t Cols> 00024 struct XprIdentity 00025 : public TvmetBase< XprIdentity<T, Rows, Cols> > 00026 { 00027 XprIdentity& operator=(const XprIdentity&); 00028 00029 public: 00030 typedef T value_type; 00031 00032 public: 00033 /** Complexity counter. */ 00034 enum { 00035 ops_assign = Rows * Cols, 00036 ops = ops_assign 00037 }; 00038 00039 public: 00040 /** access by index. */ 00041 value_type operator()(std::size_t i, std::size_t j) const { 00042 return i==j ? 1 : 0; 00043 } 00044 00045 public: // debugging Xpr parse tree 00046 void print_xpr(std::ostream& os, std::size_t l=0) const { 00047 os << IndentLevel(l++) 00048 << "XprIdentity[O="<< ops << ")]<" 00049 << std::endl; 00050 os << IndentLevel(l) 00051 << typeid(T).name() << "," 00052 << "R=" << Rows << ", C=" << Cols << std::endl; 00053 os << IndentLevel(--l) << ">" 00054 << ((l != 0) ? "," : "") << std::endl; 00055 } 00056 }; 00057 00058 00059 } // namespace tvmet 00060 00061 00062 #endif // TVMET_XPR_IDENTITY_H 00063 00064 00065 // Local Variables: 00066 // mode:C++ 00067 // tab-width:8 00068 // End:
Generated on Wed Jul 13 2022 18:28:36 by
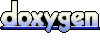