
Sample web camera program for GR-PEACH
Dependencies: GR-PEACH_video GraphicsFramework HttpServer_snapshot_mbed-os LWIPBP3595Interface_STA_for_mbed-os R_BSP RomRamBlockDevice mbed-rpc
main.cpp
00001 #include "mbed.h" 00002 #include "DisplayBace.h" 00003 #include "rtos.h" 00004 #include "JPEG_Converter.h" 00005 #include "HTTPServer.h" 00006 #include "mbed_rpc.h" 00007 #include "FATFileSystem.h" 00008 #include "RomRamBlockDevice.h" 00009 #include "file_table.h" //Binary data of web pages 00010 #include "i2c_setting.h" 00011 00012 #define VIDEO_CVBS (0) /* Analog Video Signal */ 00013 #define VIDEO_CMOS_CAMERA (1) /* Digital Video Signal */ 00014 #define VIDEO_YCBCR422 (0) 00015 #define VIDEO_RGB888 (1) 00016 #define VIDEO_RGB565 (2) 00017 00018 /**** User Selection *********/ 00019 /** Network setting **/ 00020 #define USE_DHCP (0) /* Select 0(static configuration) or 1(use DHCP) */ 00021 #if (USE_DHCP == 0) 00022 #define IP_ADDRESS ("192.168.0.2") /* IP address */ 00023 #define SUBNET_MASK ("255.255.255.0") /* Subnet mask */ 00024 #define DEFAULT_GATEWAY ("192.168.0.3") /* Default gateway */ 00025 #endif 00026 #define NETWORK_TYPE (0) /* Select 0(EthernetInterface) or 1(GR_PEACH_WlanBP3595) */ 00027 #if (NETWORK_TYPE == 1) 00028 #define SCAN_NETWORK (1) /* Select 0(Use WLAN_SSID, WLAN_PSK, WLAN_SECURITY) or 1(To select a network using the terminal.) */ 00029 #define WLAN_SSID ("SSIDofYourAP") /* SSID */ 00030 #define WLAN_PSK ("PSKofYourAP") /* PSK(Pre-Shared Key) */ 00031 #define WLAN_SECURITY NSAPI_SECURITY_WPA_WPA2 /* NSAPI_SECURITY_NONE, NSAPI_SECURITY_WEP, NSAPI_SECURITY_WPA, NSAPI_SECURITY_WPA2 or NSAPI_SECURITY_WPA_WPA2 */ 00032 #endif 00033 /** Camera setting **/ 00034 #define VIDEO_INPUT_METHOD (VIDEO_CMOS_CAMERA) /* Select VIDEO_CVBS or VIDEO_CMOS_CAMERA */ 00035 #define VIDEO_INPUT_FORMAT (VIDEO_YCBCR422) /* Select VIDEO_YCBCR422 or VIDEO_RGB888 or VIDEO_RGB565 */ 00036 #define USE_VIDEO_CH (0) /* Select 0 or 1 If selecting VIDEO_CMOS_CAMERA, should be 0.) */ 00037 #define VIDEO_PAL (0) /* Select 0(NTSC) or 1(PAL) If selecting VIDEO_CVBS, this parameter is not referenced.) */ 00038 /*****************************/ 00039 00040 #if USE_VIDEO_CH == (0) 00041 #define VIDEO_INPUT_CH (DisplayBase::VIDEO_INPUT_CHANNEL_0) 00042 #define VIDEO_INT_TYPE (DisplayBase::INT_TYPE_S0_VFIELD) 00043 #else 00044 #define VIDEO_INPUT_CH (DisplayBase::VIDEO_INPUT_CHANNEL_1) 00045 #define VIDEO_INT_TYPE (DisplayBase::INT_TYPE_S1_VFIELD) 00046 #endif 00047 00048 #if ( VIDEO_INPUT_FORMAT == VIDEO_YCBCR422 || VIDEO_INPUT_FORMAT == VIDEO_RGB565 ) 00049 #define DATA_SIZE_PER_PIC (2u) 00050 #else 00051 #define DATA_SIZE_PER_PIC (4u) 00052 #endif 00053 00054 /*! Frame buffer stride: Frame buffer stride should be set to a multiple of 32 or 128 00055 in accordance with the frame buffer burst transfer mode. */ 00056 #define PIXEL_HW (320u) /* QVGA */ 00057 #define PIXEL_VW (240u) /* QVGA */ 00058 00059 #define VIDEO_BUFFER_STRIDE (((PIXEL_HW * DATA_SIZE_PER_PIC) + 31u) & ~31u) 00060 #define VIDEO_BUFFER_HEIGHT (PIXEL_VW) 00061 00062 #if (NETWORK_TYPE == 0) 00063 #include "EthernetInterface.h" 00064 EthernetInterface network; 00065 #elif (NETWORK_TYPE == 1) 00066 #include "LWIPBP3595Interface.h" 00067 LWIPBP3595Interface network; 00068 DigitalOut usb1en(P3_8); 00069 #else 00070 #error NETWORK_TYPE error 00071 #endif /* NETWORK_TYPE */ 00072 FATFileSystem fs("storage"); 00073 RomRamBlockDevice romram_bd(512000, 512); 00074 Serial pc(USBTX, USBRX); 00075 00076 #if defined(__ICCARM__) 00077 #pragma data_alignment=16 00078 static uint8_t FrameBuffer_Video[VIDEO_BUFFER_STRIDE * VIDEO_BUFFER_HEIGHT]@ ".mirrorram"; //16 bytes aligned!; 00079 #pragma data_alignment=4 00080 #else 00081 static uint8_t FrameBuffer_Video[VIDEO_BUFFER_STRIDE * VIDEO_BUFFER_HEIGHT]__attribute((section("NC_BSS"),aligned(16))); //16 bytes aligned!; 00082 #endif 00083 static volatile int32_t vsync_count = 0; 00084 #if VIDEO_INPUT_METHOD == VIDEO_CVBS 00085 static volatile int32_t vfield_count = 1; 00086 #endif 00087 #if defined(__ICCARM__) 00088 #pragma data_alignment=8 00089 static uint8_t JpegBuffer[2][1024 * 50]@ ".mirrorram"; //8 bytes aligned!; 00090 #pragma data_alignment=4 00091 #else 00092 static uint8_t JpegBuffer[2][1024 * 50]__attribute((section("NC_BSS"),aligned(8))); //8 bytes aligned!; 00093 #endif 00094 static size_t jcu_encode_size[2]; 00095 static int image_change = 0; 00096 JPEG_Converter Jcu; 00097 static int jcu_buf_index_write = 0; 00098 static int jcu_buf_index_write_done = 0; 00099 static int jcu_buf_index_read = 0; 00100 static int jcu_encoding = 0; 00101 static char i2c_setting_str_buf[I2C_SETTING_STR_BUF_SIZE]; 00102 00103 static void JcuEncodeCallBackFunc(JPEG_Converter::jpeg_conv_error_t err_code) { 00104 jcu_buf_index_write_done = jcu_buf_index_write; 00105 image_change = 1; 00106 jcu_encoding = 0; 00107 } 00108 00109 static void IntCallbackFunc_Vfield(DisplayBase::int_type_t int_type) { 00110 //Interrupt callback function 00111 #if VIDEO_INPUT_METHOD == VIDEO_CVBS 00112 if (vfield_count != 0) { 00113 vfield_count = 0; 00114 } else { 00115 vfield_count = 1; 00116 #else 00117 { 00118 #endif 00119 JPEG_Converter::bitmap_buff_info_t bitmap_buff_info; 00120 JPEG_Converter::encode_options_t encode_options; 00121 00122 bitmap_buff_info.width = PIXEL_HW; 00123 bitmap_buff_info.height = PIXEL_VW; 00124 bitmap_buff_info.format = JPEG_Converter::WR_RD_YCbCr422; 00125 bitmap_buff_info.buffer_address = (void *)FrameBuffer_Video; 00126 00127 encode_options.encode_buff_size = sizeof(JpegBuffer[0]); 00128 encode_options.p_EncodeCallBackFunc = &JcuEncodeCallBackFunc; 00129 00130 jcu_encoding = 1; 00131 if (jcu_buf_index_read == jcu_buf_index_write) { 00132 if (jcu_buf_index_write != 0) { 00133 jcu_buf_index_write = 0; 00134 } else { 00135 jcu_buf_index_write = 1; 00136 } 00137 } 00138 jcu_encode_size[jcu_buf_index_write] = 0; 00139 if (Jcu.encode(&bitmap_buff_info, JpegBuffer[jcu_buf_index_write], &jcu_encode_size[jcu_buf_index_write], &encode_options) != JPEG_Converter::JPEG_CONV_OK) { 00140 jcu_encode_size[jcu_buf_index_write] = 0; 00141 jcu_encoding = 0; 00142 } 00143 } 00144 } 00145 00146 static void IntCallbackFunc_Vsync(DisplayBase::int_type_t int_type) { 00147 //Interrupt callback function for Vsync interruption 00148 if (vsync_count > 0) { 00149 vsync_count--; 00150 } 00151 } 00152 00153 static void WaitVsync(const int32_t wait_count) { 00154 //Wait for the specified number of times Vsync occurs 00155 vsync_count = wait_count; 00156 while (vsync_count > 0) { 00157 /* Do nothing */ 00158 } 00159 } 00160 00161 static void camera_start(void) { 00162 DisplayBase::graphics_error_t error; 00163 00164 #if VIDEO_INPUT_METHOD == VIDEO_CMOS_CAMERA 00165 DisplayBase::video_ext_in_config_t ext_in_config; 00166 PinName cmos_camera_pin[11] = { 00167 /* data pin */ 00168 P2_7, P2_6, P2_5, P2_4, P2_3, P2_2, P2_1, P2_0, 00169 /* control pin */ 00170 P10_0, /* DV0_CLK */ 00171 P1_0, /* DV0_Vsync */ 00172 P1_1 /* DV0_Hsync */ 00173 }; 00174 #endif 00175 00176 /* Create DisplayBase object */ 00177 DisplayBase Display; 00178 00179 /* Graphics initialization process */ 00180 error = Display.Graphics_init(NULL); 00181 if (error != DisplayBase::GRAPHICS_OK) { 00182 printf("Line %d, error %d\n", __LINE__, error); 00183 while (1); 00184 } 00185 00186 #if VIDEO_INPUT_METHOD == VIDEO_CVBS 00187 error = Display.Graphics_Video_init( DisplayBase::INPUT_SEL_VDEC, NULL); 00188 if( error != DisplayBase::GRAPHICS_OK ) { 00189 printf("Line %d, error %d\n", __LINE__, error); 00190 while(1); 00191 } 00192 00193 #elif VIDEO_INPUT_METHOD == VIDEO_CMOS_CAMERA 00194 /* MT9V111 camera input config */ 00195 ext_in_config.inp_format = DisplayBase::VIDEO_EXTIN_FORMAT_BT601; /* BT601 8bit YCbCr format */ 00196 ext_in_config.inp_pxd_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing data */ 00197 ext_in_config.inp_vs_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing Vsync signals */ 00198 ext_in_config.inp_hs_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing Hsync signals */ 00199 ext_in_config.inp_endian_on = DisplayBase::OFF; /* External input bit endian change on/off */ 00200 ext_in_config.inp_swap_on = DisplayBase::OFF; /* External input B/R signal swap on/off */ 00201 ext_in_config.inp_vs_inv = DisplayBase::SIG_POL_NOT_INVERTED; /* External input DV_VSYNC inversion control */ 00202 ext_in_config.inp_hs_inv = DisplayBase::SIG_POL_INVERTED; /* External input DV_HSYNC inversion control */ 00203 ext_in_config.inp_f525_625 = DisplayBase::EXTIN_LINE_525; /* Number of lines for BT.656 external input */ 00204 ext_in_config.inp_h_pos = DisplayBase::EXTIN_H_POS_CRYCBY; /* Y/Cb/Y/Cr data string start timing to Hsync reference */ 00205 ext_in_config.cap_vs_pos = 6; /* Capture start position from Vsync */ 00206 ext_in_config.cap_hs_pos = 150; /* Capture start position form Hsync */ 00207 ext_in_config.cap_width = 640; /* Capture width */ 00208 ext_in_config.cap_height = 468u; /* Capture height Max 468[line] 00209 Due to CMOS(MT9V111) output signal timing and VDC5 specification */ 00210 error = Display.Graphics_Video_init( DisplayBase::INPUT_SEL_EXT, &ext_in_config); 00211 if( error != DisplayBase::GRAPHICS_OK ) { 00212 printf("Line %d, error %d\n", __LINE__, error); 00213 while(1); 00214 } 00215 00216 /* MT9V111 camera input port setting */ 00217 error = Display.Graphics_Dvinput_Port_Init(cmos_camera_pin, 11); 00218 if( error != DisplayBase::GRAPHICS_OK ) { 00219 printf("Line %d, error %d\n", __LINE__, error); 00220 while (1); 00221 } 00222 #endif 00223 00224 /* Interrupt callback function setting (Vsync signal input to scaler 0) */ 00225 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_VI_VSYNC, 0, IntCallbackFunc_Vsync); 00226 if (error != DisplayBase::GRAPHICS_OK) { 00227 printf("Line %d, error %d\n", __LINE__, error); 00228 while (1); 00229 } 00230 /* Video capture setting (progressive form fixed) */ 00231 error = Display.Video_Write_Setting( 00232 VIDEO_INPUT_CH, 00233 #if VIDEO_PAL == 0 00234 DisplayBase::COL_SYS_NTSC_358, 00235 #else 00236 DisplayBase::COL_SYS_PAL_443, 00237 #endif 00238 FrameBuffer_Video, 00239 VIDEO_BUFFER_STRIDE, 00240 #if VIDEO_INPUT_FORMAT == VIDEO_YCBCR422 00241 DisplayBase::VIDEO_FORMAT_YCBCR422, 00242 DisplayBase::WR_RD_WRSWA_NON, 00243 #elif VIDEO_INPUT_FORMAT == VIDEO_RGB565 00244 DisplayBase::VIDEO_FORMAT_RGB565, 00245 DisplayBase::WR_RD_WRSWA_32_16BIT, 00246 #else 00247 DisplayBase::VIDEO_FORMAT_RGB888, 00248 DisplayBase::WR_RD_WRSWA_32BIT, 00249 #endif 00250 PIXEL_VW, 00251 PIXEL_HW 00252 ); 00253 if (error != DisplayBase::GRAPHICS_OK) { 00254 printf("Line %d, error %d\n", __LINE__, error); 00255 while (1); 00256 } 00257 00258 /* Interrupt callback function setting (Field end signal for recording function in scaler 0) */ 00259 error = Display.Graphics_Irq_Handler_Set(VIDEO_INT_TYPE, 0, IntCallbackFunc_Vfield); 00260 if (error != DisplayBase::GRAPHICS_OK) { 00261 printf("Line %d, error %d\n", __LINE__, error); 00262 while (1); 00263 } 00264 00265 /* Video write process start */ 00266 error = Display.Video_Start (VIDEO_INPUT_CH); 00267 if (error != DisplayBase::GRAPHICS_OK) { 00268 printf("Line %d, error %d\n", __LINE__, error); 00269 while (1); 00270 } 00271 00272 /* Video write process stop */ 00273 error = Display.Video_Stop (VIDEO_INPUT_CH); 00274 if (error != DisplayBase::GRAPHICS_OK) { 00275 printf("Line %d, error %d\n", __LINE__, error); 00276 while (1); 00277 } 00278 00279 /* Video write process start */ 00280 error = Display.Video_Start (VIDEO_INPUT_CH); 00281 if (error != DisplayBase::GRAPHICS_OK) { 00282 printf("Line %d, error %d\n", __LINE__, error); 00283 while (1); 00284 } 00285 00286 /* Wait vsync to update resister */ 00287 WaitVsync(1); 00288 } 00289 00290 static int snapshot_req(const char ** pp_data) { 00291 int encode_size; 00292 00293 while ((jcu_encoding == 1) || (image_change == 0)) { 00294 Thread::wait(1); 00295 } 00296 jcu_buf_index_read = jcu_buf_index_write_done; 00297 image_change = 0; 00298 00299 *pp_data = (const char *)JpegBuffer[jcu_buf_index_read]; 00300 encode_size = (int)jcu_encode_size[jcu_buf_index_read]; 00301 00302 return encode_size; 00303 } 00304 00305 static void TerminalWrite(Arguments* arg, Reply* r) { 00306 if ((arg != NULL) && (r != NULL)) { 00307 for (int i = 0; i < arg->argc; i++) { 00308 if (arg->argv[i] != NULL) { 00309 printf("%s", arg->argv[i]); 00310 } 00311 } 00312 printf("\n"); 00313 r->putData<const char*>("ok"); 00314 } 00315 } 00316 00317 static void mount_romramfs(void) { 00318 FILE * fp; 00319 00320 romram_bd.SetRomAddr(0x18000000, 0x1FFFFFFF); 00321 fs.format(&romram_bd, 512); 00322 fs.mount(&romram_bd); 00323 00324 //index.htm 00325 fp = fopen("/storage/index.htm", "w"); 00326 fwrite(index_htm_tbl, sizeof(char), sizeof(index_htm_tbl), fp); 00327 fclose(fp); 00328 00329 //camera.js 00330 fp = fopen("/storage/camera.js", "w"); 00331 fwrite(camaera_js_tbl, sizeof(char), sizeof(camaera_js_tbl), fp); 00332 fclose(fp); 00333 00334 //camera.htm 00335 fp = fopen("/storage/camera.htm", "w"); 00336 fwrite(camera_htm_tbl, sizeof(char), sizeof(camera_htm_tbl), fp); 00337 fclose(fp); 00338 00339 //mbedrpc.js 00340 fp = fopen("/storage/mbedrpc.js", "w"); 00341 fwrite(mbedrpc_js_tbl, sizeof(char), sizeof(mbedrpc_js_tbl), fp); 00342 fclose(fp); 00343 00344 //led.htm 00345 fp = fopen("/storage/led.htm", "w"); 00346 fwrite(led_htm_tbl, sizeof(char), sizeof(led_htm_tbl), fp); 00347 fclose(fp); 00348 00349 //i2c_set.htm 00350 fp = fopen("/storage/i2c_set.htm", "w"); 00351 fwrite(i2c_set_htm_tbl, sizeof(char), sizeof(i2c_set_htm_tbl), fp); 00352 fclose(fp); 00353 00354 //web_top.htm 00355 fp = fopen("/storage/web_top.htm", "w"); 00356 fwrite(web_top_htm_tbl, sizeof(char), sizeof(web_top_htm_tbl), fp); 00357 fclose(fp); 00358 00359 //menu.htm 00360 fp = fopen("/storage/menu.htm", "w"); 00361 fwrite(menu_htm_tbl, sizeof(char), sizeof(menu_htm_tbl), fp); 00362 fclose(fp); 00363 00364 //window.htm 00365 fp = fopen("/storage/window.htm", "w"); 00366 fwrite(window_htm_tbl, sizeof(char), sizeof(window_htm_tbl), fp); 00367 fclose(fp); 00368 } 00369 00370 static void SetI2CfromWeb(Arguments* arg, Reply* r) { 00371 int result = 0; 00372 00373 if (arg != NULL) { 00374 if (arg->argc >= 2) { 00375 if ((arg->argv[0] != NULL) && (arg->argv[1] != NULL)) { 00376 sprintf(i2c_setting_str_buf, "%s,%s", arg->argv[0], arg->argv[1]); 00377 result = 1; 00378 } 00379 } else if (arg->argc == 1) { 00380 if (arg->argv[0] != NULL) { 00381 sprintf(i2c_setting_str_buf, "%s", arg->argv[0]); 00382 result = 1; 00383 } 00384 } else { 00385 /* Do nothing */ 00386 } 00387 /* command analysis and execute */ 00388 if (result != 0) { 00389 if (i2c_setting_exe(i2c_setting_str_buf) != false) { 00390 r->putData<const char*>(i2c_setting_str_buf); 00391 } 00392 } 00393 } 00394 } 00395 00396 #if (SCAN_NETWORK == 1) 00397 static const char *sec2str(nsapi_security_t sec) { 00398 switch (sec) { 00399 case NSAPI_SECURITY_NONE: 00400 return "None"; 00401 case NSAPI_SECURITY_WEP: 00402 return "WEP"; 00403 case NSAPI_SECURITY_WPA: 00404 return "WPA"; 00405 case NSAPI_SECURITY_WPA2: 00406 return "WPA2"; 00407 case NSAPI_SECURITY_WPA_WPA2: 00408 return "WPA/WPA2"; 00409 case NSAPI_SECURITY_UNKNOWN: 00410 default: 00411 return "Unknown"; 00412 } 00413 } 00414 00415 static bool scan_network(WiFiInterface *wifi) { 00416 WiFiAccessPoint *ap; 00417 bool ret = false; 00418 int i; 00419 int count = 8; /* Limit number of network arbitrary to 8 */ 00420 00421 printf("Scan:\r\n"); 00422 ap = new WiFiAccessPoint[count]; 00423 count = wifi->scan(ap, count); 00424 for (i = 0; i < count; i++) { 00425 printf("No.%d Network: %s secured: %s BSSID: %hhX:%hhX:%hhX:%hhx:%hhx:%hhx RSSI: %hhd Ch: %hhd\r\n", i, ap[i].get_ssid(), 00426 sec2str(ap[i].get_security()), ap[i].get_bssid()[0], ap[i].get_bssid()[1], ap[i].get_bssid()[2], 00427 ap[i].get_bssid()[3], ap[i].get_bssid()[4], ap[i].get_bssid()[5], ap[i].get_rssi(), ap[i].get_channel()); 00428 } 00429 printf("%d networks available.\r\n", count); 00430 00431 if (count > 0) { 00432 char c; 00433 char pass[64]; 00434 int select_no; 00435 bool loop_break = false;; 00436 00437 printf("\nPlease enter the number of the network you want to connect.\r\n"); 00438 printf("Enter key:[0]-[%d], (If inputting the other key, it's scanned again.)\r\n", count - 1); 00439 c = (uint8_t)pc.getc(); 00440 select_no = c - 0x30; 00441 if ((select_no >= 0) && (select_no < count)) { 00442 printf("[%s] is selected.\r\n", ap[select_no].get_ssid()); 00443 printf("Please enter the PSK.\r\n"); 00444 i = 0; 00445 while (loop_break == false) { 00446 c = (uint8_t)pc.getc(); 00447 switch (c) { 00448 case 0x0D: 00449 pass[i] = '\0'; 00450 pc.puts("\r\n"); 00451 loop_break = true; 00452 break; 00453 case 0x08: 00454 if (i > 0) { 00455 pc.puts("\b \b"); 00456 i--; 00457 } 00458 break; 00459 case 0x0A: 00460 break; 00461 default: 00462 if ((i + 1) < sizeof(pass)) { 00463 pass[i] = c; 00464 i++; 00465 pc.putc(c); 00466 } 00467 break; 00468 } 00469 } 00470 printf("connecting...\r\n"); 00471 wifi->set_credentials(ap[select_no].get_ssid(), pass, ap[select_no].get_security()); 00472 ret = true; 00473 } 00474 } 00475 00476 delete[] ap; 00477 00478 return ret; 00479 } 00480 #endif 00481 00482 int main(void) { 00483 printf("********* PROGRAM START ***********\r\n"); 00484 00485 /* Please enable this line when performing the setting from the Terminal side. */ 00486 // Thread thread(SetI2CfromTerm, NULL, osPriorityBelowNormal, DEFAULT_STACK_SIZE); 00487 00488 mount_romramfs(); //RomRamFileSystem Mount 00489 camera_start(); //Camera Start 00490 00491 RPC::add_rpc_class<RpcDigitalOut>(); 00492 RPC::construct<RpcDigitalOut, PinName, const char*>(LED1, "led1"); 00493 RPC::construct<RpcDigitalOut, PinName, const char*>(LED2, "led2"); 00494 RPC::construct<RpcDigitalOut, PinName, const char*>(LED3, "led3"); 00495 RPCFunction rpcFunc(TerminalWrite, "TerminalWrite"); 00496 RPCFunction rpcSetI2C(SetI2CfromWeb, "SetI2CfromWeb"); 00497 00498 #if (NETWORK_TYPE == 1) 00499 //Audio Camera Shield USB1 enable for WlanBP3595 00500 usb1en = 1; //Outputs high level 00501 Thread::wait(5); 00502 usb1en = 0; //Outputs low level 00503 Thread::wait(5); 00504 #endif 00505 00506 printf("Network Setting up...\r\n"); 00507 #if (USE_DHCP == 0) 00508 network.set_dhcp(false); 00509 if (network.set_network(IP_ADDRESS, SUBNET_MASK, DEFAULT_GATEWAY) != 0) { //for Static IP Address (IPAddress, NetMasks, Gateway) 00510 printf("Network Set Network Error \r\n"); 00511 return -1; 00512 } 00513 #endif 00514 #if (NETWORK_TYPE == 1) 00515 #if (SCAN_NETWORK == 1) 00516 while (!scan_network(&network)); 00517 #else 00518 network.set_credentials(WLAN_SSID, WLAN_PSK, WLAN_SECURITY); 00519 #endif 00520 #endif 00521 if (network.connect() != 0) { 00522 printf("Network Connect Error \r\n"); 00523 return -1; 00524 } 00525 printf("MAC Address is %s\r\n", network.get_mac_address()); 00526 printf("IP Address is %s\r\n", network.get_ip_address()); 00527 printf("NetMask is %s\r\n", network.get_netmask()); 00528 printf("Gateway Address is %s\r\n", network.get_gateway()); 00529 printf("Network Setup OK\r\n"); 00530 00531 SnapshotHandler::attach_req(&snapshot_req); 00532 HTTPServerAddHandler<SnapshotHandler>("/camera"); //Camera 00533 FSHandler::mount("/storage", "/"); 00534 HTTPServerAddHandler<FSHandler>("/"); 00535 HTTPServerAddHandler<RPCHandler>("/rpc"); 00536 HTTPServerStart(&network, 80); 00537 }
Generated on Sat Jul 23 2022 10:27:07 by
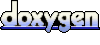