A simple 128x32 graphical LCD program to quickstart with LCD on ARM mbed IoT Starter Kit. This requires mbed Applciation Shield with FRDM-K64F platform.
Timer Class Reference
A general purpose timer. More...
#include <Timer.h>
Public Member Functions | |
void | start () |
Start the timer. | |
void | stop () |
Stop the timer. | |
void | reset () |
Reset the timer to 0. | |
float | read () |
Get the time passed in seconds. | |
int | read_ms () |
Get the time passed in mili-seconds. | |
int | read_us () |
Get the time passed in micro-seconds. |
Detailed Description
A general purpose timer.
Example:
// Count the time to toggle a LED #include "mbed.h" Timer timer; DigitalOut led(LED1); int begin, end; int main() { timer.start(); begin = timer.read_us(); led = !led; end = timer.read_us(); printf("Toggle the led takes %d us", end - begin); }
Definition at line 44 of file Timer.h.
Member Function Documentation
float read | ( | ) |
Get the time passed in seconds.
int read_ms | ( | ) |
Get the time passed in mili-seconds.
int read_us | ( | ) |
Get the time passed in micro-seconds.
void reset | ( | ) |
Reset the timer to 0.
If it was already counting, it will continue
void start | ( | ) |
Start the timer.
void stop | ( | ) |
Stop the timer.
Generated on Tue Jul 12 2022 18:19:34 by
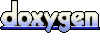