
Modified Bomberman Game in mbed
Dependencies: 4DGL-uLCD-SE USBDevice mbed
main.cpp
00001 // uLCD-144-G2 demo program for uLCD-4GL LCD driver library 00002 // 00003 #include "mbed.h" 00004 #include "uLCD_4DGL.h" 00005 #include "rtos.h" 00006 00007 Ticker dirSet; 00008 Ticker XorY; 00009 Serial pc (USBTX,USBRX); 00010 Mutex mutex; 00011 00012 uLCD_4DGL uLCD(p9,p10,p11); // serial tx, serial rx, reset pin; 00013 float fx1=rand()%100+1,fy1=rand()%100+1,fx2=rand()%100+1,fy2=rand()%100+1,fx3=rand()%100+1,fy3=rand()%100+1, vx=1, vy=1; 00014 int x1=(int)fx1,y1=(int)fy1,x2=(int)fx2,y2=(int)fy2,x3=(int)fx3,y3=(int)fy3, radius=3; 00015 int xory1; 00016 int xory2; 00017 int xdir1; 00018 int xdir2; 00019 int ydir1; 00020 int ydir2; 00021 int hit1=0; 00022 int hit2=0; 00023 int timer=0; 00024 int x4; 00025 int y4; 00026 char move; 00027 00028 void drawWalls() { 00029 //draw walls 00030 uLCD.line(0, 0, 127, 0, WHITE); 00031 uLCD.line(127, 0, 127, 127, WHITE); 00032 uLCD.line(127, 127, 0, 127, WHITE); 00033 uLCD.line(0, 127, 0, 0, WHITE); 00034 } 00035 00036 void setxory(){ 00037 xory1 = rand()%2; 00038 xory2 = rand()%2; 00039 } 00040 00041 void setDir(){ 00042 //set value of movement left/right and up/down 00043 xdir1 = rand()%2; 00044 ydir1 = rand()%2; 00045 xdir2 = rand()%2; 00046 ydir2 = rand()%2; 00047 } 00048 00049 void drawBaddie1() { 00050 //draw baddie1 00051 uLCD.filled_circle(x1, y1, radius, RED); 00052 } 00053 00054 void drawBaddie2() { 00055 //draw baddie2 00056 uLCD.filled_circle(x2, y2, radius, RED); 00057 } 00058 void eraseBaddie1() { 00059 //erase old baddie1's locations 00060 uLCD.filled_circle(x1, y1, radius, BLACK); 00061 } 00062 void eraseBaddie2() { 00063 //erase old baddie2's locations 00064 uLCD.filled_circle(x2, y2, radius, BLACK); 00065 } 00066 void moveBaddie1() { 00067 //move baddie1 00068 if (xory1==0){ 00069 if ((xdir1==0)||(x1<=radius+1)) fx1=fx1+vx; 00070 if ((xdir1==1)||(x1>=126-radius)) fx1=fx1-vx; 00071 } else if (xory1==1){ 00072 if ((ydir1==0)||(y1<=radius+1)) fy1=fy1+vy; 00073 if ((ydir1==1)||(y1>=126-radius)) fy1=fy1-vy; 00074 } 00075 x1=(int)fx1; 00076 y1=(int)fy1; 00077 } 00078 00079 void moveBaddie2() { 00080 //move baddie2 00081 if (xory2==0){ 00082 if ((xdir2==0)||(x2<=radius+1)) fx2=fx2+vx; 00083 if ((xdir2==1)||(x2>=126-radius)) fx2=fx2-vx; 00084 } else if (xory1==1){ 00085 if ((ydir2==0)||(y2<=radius+1)) fy2=fy2+vy; 00086 if ((ydir2==1)||(y2>=126-radius)) fy2=fy2-vy; 00087 } 00088 x2=(int)fx2; 00089 y2=(int)fy2; 00090 } 00091 00092 void drawHero() { 00093 //draw Hero 00094 uLCD.filled_circle(x3, y3, radius, GREEN); 00095 } 00096 00097 void eraseHero() { 00098 //erase old hero's location 00099 uLCD.filled_circle(x3, y3, radius, BLACK); 00100 } 00101 00102 void moveHero() { 00103 //move hero's location 00104 if (pc.readable()){ 00105 move = pc.getc(); 00106 if ((move=='d')||(x3<=radius+1)) fx3=fx3+2; 00107 else if ((move=='a')||(x3>=126-radius)) fx3=fx3-2; 00108 else if ((move=='s')||(y3<=radius+1)) fy3=fy3+2; 00109 else if ((move=='w')||(y3>=126-radius)) fy3=fy3-2; 00110 } 00111 x3=(int)fx3; 00112 y3=(int)fy3; 00113 } 00114 void bomb() { 00115 if (move=='m'){ 00116 pc.printf("Boooooom!\n\r"); 00117 x4=x3; 00118 y4=y3; 00119 while(timer<=50){ 00120 uLCD.filled_circle(x4,y4,radius,BLUE); 00121 drawHero(); 00122 eraseHero(); 00123 moveHero(); 00124 drawHero(); 00125 drawBaddie1(); 00126 eraseBaddie1(); 00127 moveBaddie1(); 00128 drawBaddie1(); 00129 drawBaddie2(); 00130 eraseBaddie2(); 00131 moveBaddie2(); 00132 drawBaddie2(); 00133 timer++; 00134 } 00135 timer=0; 00136 uLCD.filled_rectangle(x4+3, y4+20, x4-3, y4-20, BLUE); 00137 uLCD.filled_rectangle(x4+20, y4+3, x4-20, y4-3, BLUE); 00138 wait(1); 00139 uLCD.filled_rectangle(x4+3, y4+20, x4-3, y4-20, BLACK); 00140 uLCD.filled_rectangle(x4+20, y4+3, x4-20, y4-3, BLACK); 00141 if ((((x1-radius)<=(x4+20))&&((y1>=y4-5)&&(y1<=y4+5)))&&(((x1+radius)>=(x4-20))&&((y1>=y4-5)&&(y1<=y4+5)))){ 00142 hit1=1; 00143 eraseBaddie1(); 00144 } 00145 else if((((y1-radius)<=(y4+20))&&((x1>=x4-5)&&(x1<=x4+5)))&&(((y1-radius)>=(y4-20))&&((x1>=x4-5)&&(x1<=x4+5)))){ 00146 hit1=1; 00147 eraseBaddie1(); 00148 } 00149 else if ((((x2-radius)<=(x4+20))&&((y2>=y4-5)&&(y2<=y4+5)))&&(((x2+radius)>=(x4-20))&&((y2>=y4-5)&&(y2<=y4+5)))){ 00150 hit2=1; 00151 eraseBaddie2(); 00152 } 00153 else if ((((y2-radius)<=(y4+20))&&((x2>=x4-5)&&(x2<=x4+5)))&&(((y2-radius)>=(y4-20))&&((x2>=x4-5)&&(x2<=x4+5)))){ 00154 hit2=1; 00155 eraseBaddie1(); 00156 } 00157 } 00158 } 00159 00160 int main(){ 00161 uLCD.baudrate(300000); 00162 uLCD.background_color(BLACK); 00163 uLCD.cls(); 00164 uLCD.text_width(1); 00165 uLCD.text_height(1); 00166 uLCD.printf("Modified Bomberman\n\r\n\rPress s\n\rto start Game"); 00167 if(pc.getc()=='s'){ 00168 uLCD.background_color(BLACK); 00169 uLCD.cls(); 00170 } 00171 drawWalls(); 00172 XorY.attach(&setxory, 0.5); 00173 dirSet.attach(&setDir,0.5); 00174 while(1){ 00175 drawHero(); 00176 eraseHero(); 00177 moveHero(); 00178 bomb(); 00179 drawHero(); 00180 if(hit1!=1){ 00181 drawBaddie1(); 00182 eraseBaddie1(); 00183 moveBaddie1(); 00184 drawBaddie1(); 00185 } 00186 if(hit2!=1){ 00187 drawBaddie2(); 00188 eraseBaddie2(); 00189 moveBaddie2(); 00190 drawBaddie2(); 00191 } 00192 if(hit1==1&&hit2==1) 00193 { 00194 uLCD.cls(); 00195 uLCD.printf("YOU WIN!"); 00196 break; 00197 } 00198 } 00199 }
Generated on Mon Jul 18 2022 19:58:21 by
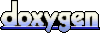