An eddied version of http://mbed.org/users/crazystick/code/DHT22/ for LPC11U24. All printf statements are removed and features requiring the real time clock are removed.
Dependents: RHT03_HelloWorld IOT_sensor_nfc CanSat_Alex cansat_alex_v1 ... more
RHT03.cpp
00001 /* 00002 RHT22.cpp - RHT22 sensor library 00003 Developed by HO WING KIT 00004 Modifications by Paul Adams 00005 More modifications by Tristan Hughes 00006 00007 This library is free software; you can redistribute it and / or 00008 modify it under the terms of the GNU Leser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRENTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PATRICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 00017 You should have received a copy of the GNU Lesser General Public 00018 License along with this library; if not, write to the Free Software 00019 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00020 00021 00022 Humidity and Temperature Sensor RHT22 info found at 00023 http://www.sparkfun.com/products/10167 00024 same as RHT03 http://www.humiditycn.com 00025 00026 Version 0.1: 8-Jan-2011 by Ho Wing Kit 00027 Version 0.2: 29-Mar-2012 by Paul Adams 00028 00029 Additional Credit: All the commenters on http://www.sparkfun.com/products/10167 00030 00031 */ 00032 00033 #include "RHT03.h" 00034 00035 00036 // This should be 40, but the sensor is adding an extra bit at the start 00037 #define RHT03_DATA_BIT_COUNT 41 00038 00039 RHT03::RHT03(PinName Data) { 00040 00041 _data = Data; // Set Data Pin 00042 _lastReadTime = time(NULL); 00043 _lastHumidity = 0; 00044 _lastTemperature = RHT03_ERROR_VALUE; 00045 } 00046 00047 RHT03::~RHT03() { 00048 } 00049 00050 RHT03_ERROR RHT03::readData() { 00051 int i, j, retryCount; 00052 int currentTemperature=0; 00053 int currentHumidity=0; 00054 unsigned int checkSum = 0, csPart1, csPart2, csPart3, csPart4; 00055 unsigned int bitTimes[RHT03_DATA_BIT_COUNT]; 00056 RHT03_ERROR err = RHT_ERROR_NONE; 00057 time_t currentTime = time(NULL); 00058 00059 DigitalInOut DATA(_data); 00060 00061 for (i = 0; i < RHT03_DATA_BIT_COUNT; i++) { 00062 bitTimes[i] = 0; 00063 } 00064 00065 // if (int(currentTime - _lastReadTime) < 2) { 00066 // printf("RHT22 Error Access Time < 2s"); 00067 // err = RHT_ERROR_TOO_QUICK; 00068 // } 00069 retryCount = 0; 00070 // Pin needs to start HIGH, wait unit it is HIGH with a timeout 00071 do { 00072 if (retryCount > 125) { 00073 //pc1.printf("RHT22 Bus busy!"); 00074 err = RHT_BUS_HUNG; 00075 return err; 00076 } 00077 retryCount ++; 00078 wait_us(2); 00079 } while (DATA==0); // exit on RHT22 return 'High' Signal within 250us 00080 00081 // Send the activate pulse 00082 // Step 1: MCU send out start signal to RHT22 and RHT22 send 00083 // response signal to MCU. 00084 // If always signal high-voltage-level, it means RHT22 is not 00085 // working properly, please check the electrical connection status. 00086 // 00087 DATA.output(); // set pin to output data 00088 DATA = 0; // MCU send out start signal to RHT22 00089 wait_ms(18); // 18 ms wait (spec: at least 1ms) 00090 DATA = 1; // MCU pull up 00091 wait_us(40); 00092 DATA.input(); // set pin to receive data 00093 // Find the start of the ACK Pulse 00094 retryCount = 0; 00095 do { 00096 if (retryCount > 40) { // (Spec is 20-40 us high) 00097 //pc1.printf("RHT22 not responding!"); 00098 err = RHT_ERROR_NOT_PRESENT; 00099 return err; 00100 } 00101 retryCount++; 00102 wait_us(1); 00103 } while (DATA==1); // Exit on RHT22 pull low within 40us 00104 if (err != RHT_ERROR_NONE) { 00105 // initialisation failed 00106 return err; 00107 } 00108 wait_us(80); // RHT pull up ready to transmit data 00109 00110 /* 00111 if (DATA == 0) { 00112 printf("RHT22 not ready!"); 00113 err = RHT_ERROR_ACK_TOO_LONG; 00114 return err; 00115 } 00116 */ 00117 00118 // Reading the 5 byte data stream 00119 // Step 2: RHT22 send data to MCU 00120 // Start bit -> low volage within 50us (actually could be anything from 35-75us) 00121 // 0 -> high volage within 26-28us (actually could be 10-40us) 00122 // 1 -> high volage within 70us (actually could be 60-85us) 00123 // See http://www.sparkfun.com/products/10167#comment-4f118d6e757b7f536e000000 00124 00125 00126 for (i = 0; i < 5; i++) { 00127 for (j = 0; j < 8; j++) { 00128 // Instead of relying on the data sheet, just wait while the RHT03 pin is low 00129 retryCount = 0; 00130 do { 00131 if (retryCount > 75) { 00132 //pc1.printf("RHT22 timeout waiting for data!"); 00133 err = RHT_ERROR_DATA_TIMEOUT; 00134 } 00135 retryCount++; 00136 wait_us(1); 00137 } while (DATA == 0); 00138 // We now wait for 40us 00139 wait_us(40); 00140 if (DATA == 1) { 00141 // If pin is still high, bit value is a 1 00142 bitTimes[i*8+j] = 1; 00143 } else { 00144 // The bit value is a 0 00145 bitTimes[i*8+j] = 0; 00146 } 00147 int count = 0; 00148 while (DATA == 1 && count < 100) { 00149 wait_us(1); // Delay for 1 microsecond 00150 count++; 00151 } 00152 } 00153 } 00154 // Re-init RHT22 pin 00155 DATA.output(); 00156 DATA = 1; 00157 00158 // Now bitTimes have the actual bits 00159 // that were needed to find the end of each data bit 00160 // Note: the bits are offset by one from the data sheet, not sure why 00161 currentHumidity = 0; 00162 currentTemperature = 0; 00163 checkSum = 0; 00164 // First 16 bits is Humidity 00165 for (i=0; i<16; i++) { 00166 //printf("bit %d: %d ", i, bitTimes[i+1]); 00167 if (bitTimes[i+1] > 0) { 00168 currentHumidity |= ( 1 << (15-i)); 00169 } 00170 } 00171 00172 // Second 16 bits is Temperature 00173 for (i=0; i<16; i ++) { 00174 //printf("bit %d: %d ", i+16, bitTimes[i+17]); 00175 if (bitTimes[i+17] > 0) { 00176 currentTemperature |= (1 <<(15-i)); 00177 } 00178 } 00179 00180 // Last 8 bit is Checksum 00181 for (i=0; i<8; i++) { 00182 //printf("bit %d: %d ", i+32, bitTimes[i+33]); 00183 if (bitTimes[i+33] > 0) { 00184 checkSum |= (1 << (7-i)); 00185 } 00186 } 00187 00188 _lastHumidity = (float(currentHumidity) / 10.0); 00189 00190 // if first bit of currentTemperature is 1, it is negative value. 00191 if ((currentTemperature & 0x8000)==0x8000) { 00192 _lastTemperature = (float(currentTemperature & 0x7FFF) / 10.0) * -1.0; 00193 } else { 00194 _lastTemperature = float(currentTemperature) / 10.0; 00195 } 00196 00197 // Calculate Check Sum 00198 csPart1 = currentHumidity >> 8; 00199 csPart2 = currentHumidity & 0xFF; 00200 csPart3 = currentTemperature >> 8; 00201 csPart4 = currentTemperature & 0xFF; 00202 if (checkSum == ((csPart1 + csPart2 + csPart3 + csPart4) & 0xFF)) { 00203 _lastReadTime = currentTime; 00204 //pc1.printf("OK-->Temperature:%f, Humidity:%f\r\n", _lastTemperature, _lastHumidity); 00205 err = RHT_ERROR_NONE; 00206 } else { 00207 //pc1.printf("RHT22 Checksum error!\n"); 00208 //pc1.printf("Calculate check sum is %d\n",(csPart1 + csPart2 + csPart3 + csPart4) & 0xFF); 00209 //pc1.printf("Reading check sum is %d",checkSum); 00210 err = RHT_ERROR_CHECKSUM; 00211 } 00212 return err; 00213 } 00214 00215 float RHT03::getTemperatureC() { 00216 return _lastTemperature; 00217 } 00218 00219 float RHT03::getHumidity() { 00220 return _lastHumidity; 00221 }
Generated on Tue Jul 12 2022 17:09:55 by
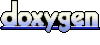