Found this library on Github and so far it is as complete as the Arduino Adafruit library.
Fork of BNO055_fusion by
BNO055.h
00001 #ifndef BNO055_H 00002 #define BNO055_H 00003 00004 #include "mbed.h" 00005 00006 #define BNOAddress (0x28 << 1) 00007 //Register definitions 00008 /* Page id register definition */ 00009 #define BNO055_PAGE_ID_ADDR 0x07 00010 /* PAGE0 REGISTER DEFINITION START*/ 00011 #define BNO055_CHIP_ID_ADDR 0x00 00012 #define BNO055_ACCEL_REV_ID_ADDR 0x01 00013 #define BNO055_MAG_REV_ID_ADDR 0x02 00014 #define BNO055_GYRO_REV_ID_ADDR 0x03 00015 #define BNO055_SW_REV_ID_LSB_ADDR 0x04 00016 #define BNO055_SW_REV_ID_MSB_ADDR 0x05 00017 #define BNO055_BL_REV_ID_ADDR 0x06 00018 /* Accel data register */ 00019 #define BNO055_ACCEL_DATA_X_LSB_ADDR 0x08 00020 #define BNO055_ACCEL_DATA_X_MSB_ADDR 0x09 00021 #define BNO055_ACCEL_DATA_Y_LSB_ADDR 0x0A 00022 #define BNO055_ACCEL_DATA_Y_MSB_ADDR 0x0B 00023 #define BNO055_ACCEL_DATA_Z_LSB_ADDR 0x0C 00024 #define BNO055_ACCEL_DATA_Z_MSB_ADDR 0x0D 00025 /* Mag data register */ 00026 #define BNO055_MAG_DATA_X_LSB_ADDR 0x0E 00027 #define BNO055_MAG_DATA_X_MSB_ADDR 0x0F 00028 #define BNO055_MAG_DATA_Y_LSB_ADDR 0x10 00029 #define BNO055_MAG_DATA_Y_MSB_ADDR 0x11 00030 #define BNO055_MAG_DATA_Z_LSB_ADDR 0x12 00031 #define BNO055_MAG_DATA_Z_MSB_ADDR 0x13 00032 /* Gyro data registers */ 00033 #define BNO055_GYRO_DATA_X_LSB_ADDR 0x14 00034 #define BNO055_GYRO_DATA_X_MSB_ADDR 0x15 00035 #define BNO055_GYRO_DATA_Y_LSB_ADDR 0x16 00036 #define BNO055_GYRO_DATA_Y_MSB_ADDR 0x17 00037 #define BNO055_GYRO_DATA_Z_LSB_ADDR 0x18 00038 #define BNO055_GYRO_DATA_Z_MSB_ADDR 0x19 00039 /* Euler data registers */ 00040 #define BNO055_EULER_H_LSB_ADDR 0x1A 00041 #define BNO055_EULER_H_MSB_ADDR 0x1B 00042 #define BNO055_EULER_R_LSB_ADDR 0x1C 00043 #define BNO055_EULER_R_MSB_ADDR 0x1D 00044 #define BNO055_EULER_P_LSB_ADDR 0x1E 00045 #define BNO055_EULER_P_MSB_ADDR 0x1F 00046 /* Quaternion data registers */ 00047 #define BNO055_QUATERNION_DATA_W_LSB_ADDR 0x20 00048 #define BNO055_QUATERNION_DATA_W_MSB_ADDR 0x21 00049 #define BNO055_QUATERNION_DATA_X_LSB_ADDR 0x22 00050 #define BNO055_QUATERNION_DATA_X_MSB_ADDR 0x23 00051 #define BNO055_QUATERNION_DATA_Y_LSB_ADDR 0x24 00052 #define BNO055_QUATERNION_DATA_Y_MSB_ADDR 0x25 00053 #define BNO055_QUATERNION_DATA_Z_LSB_ADDR 0x26 00054 #define BNO055_QUATERNION_DATA_Z_MSB_ADDR 0x27 00055 /* Linear acceleration data registers */ 00056 #define BNO055_LINEAR_ACCEL_DATA_X_LSB_ADDR 0x28 00057 #define BNO055_LINEAR_ACCEL_DATA_X_MSB_ADDR 0x29 00058 #define BNO055_LINEAR_ACCEL_DATA_Y_LSB_ADDR 0x2A 00059 #define BNO055_LINEAR_ACCEL_DATA_Y_MSB_ADDR 0x2B 00060 #define BNO055_LINEAR_ACCEL_DATA_Z_LSB_ADDR 0x2C 00061 #define BNO055_LINEAR_ACCEL_DATA_Z_MSB_ADDR 0x2D 00062 /* Gravity data registers */ 00063 #define BNO055_GRAVITY_DATA_X_LSB_ADDR 0x2E 00064 #define BNO055_GRAVITY_DATA_X_MSB_ADDR 0x2F 00065 #define BNO055_GRAVITY_DATA_Y_LSB_ADDR 0x30 00066 #define BNO055_GRAVITY_DATA_Y_MSB_ADDR 0x31 00067 #define BNO055_GRAVITY_DATA_Z_LSB_ADDR 0x32 00068 #define BNO055_GRAVITY_DATA_Z_MSB_ADDR 0x33 00069 /* Temperature data register */ 00070 #define BNO055_TEMP_ADDR 0x34 00071 /* Status registers */ 00072 #define BNO055_CALIB_STAT_ADDR 0x35 00073 #define BNO055_SELFTEST_RESULT_ADDR 0x36 00074 #define BNO055_INTR_STAT_ADDR 0x37 00075 #define BNO055_SYS_CLK_STAT_ADDR 0x38 00076 #define BNO055_SYS_STAT_ADDR 0x39 00077 #define BNO055_SYS_ERR_ADDR 0x3A 00078 /* Unit selection register */ 00079 #define BNO055_UNIT_SEL_ADDR 0x3B 00080 #define BNO055_DATA_SELECT_ADDR 0x3C 00081 /* Mode registers */ 00082 #define BNO055_OPR_MODE_ADDR 0x3D 00083 #define BNO055_PWR_MODE_ADDR 0x3E 00084 #define BNO055_SYS_TRIGGER_ADDR 0x3F 00085 #define BNO055_TEMP_SOURCE_ADDR 0x40 00086 /* Axis remap registers */ 00087 #define BNO055_AXIS_MAP_CONFIG_ADDR 0x41 00088 #define BNO055_AXIS_MAP_SIGN_ADDR 0x42 00089 /* Accelerometer Offset registers */ 00090 #define ACCEL_OFFSET_X_LSB_ADDR 0x55 00091 #define ACCEL_OFFSET_X_MSB_ADDR 0x56 00092 #define ACCEL_OFFSET_Y_LSB_ADDR 0x57 00093 #define ACCEL_OFFSET_Y_MSB_ADDR 0x58 00094 #define ACCEL_OFFSET_Z_LSB_ADDR 0x59 00095 #define ACCEL_OFFSET_Z_MSB_ADDR 0x5A 00096 /* Magnetometer Offset registers */ 00097 #define MAG_OFFSET_X_LSB_ADDR 0x5B 00098 #define MAG_OFFSET_X_MSB_ADDR 0x5C 00099 #define MAG_OFFSET_Y_LSB_ADDR 0x5D 00100 #define MAG_OFFSET_Y_MSB_ADDR 0x5E 00101 #define MAG_OFFSET_Z_LSB_ADDR 0x5F 00102 #define MAG_OFFSET_Z_MSB_ADDR 0x60 00103 /* Gyroscope Offset registers*/ 00104 #define GYRO_OFFSET_X_LSB_ADDR 0x61 00105 #define GYRO_OFFSET_X_MSB_ADDR 0x62 00106 #define GYRO_OFFSET_Y_LSB_ADDR 0x63 00107 #define GYRO_OFFSET_Y_MSB_ADDR 0x64 00108 #define GYRO_OFFSET_Z_LSB_ADDR 0x65 00109 #define GYRO_OFFSET_Z_MSB_ADDR 0x66 00110 /* Radius registers */ 00111 #define ACCEL_RADIUS_LSB_ADDR 0x67 00112 #define ACCEL_RADIUS_MSB_ADDR 0x68 00113 #define MAG_RADIUS_LSB_ADDR 0x69 00114 #define MAG_RADIUS_MSB_ADDR 0x6A 00115 00116 /* Page 1 registers */ 00117 #define BNO055_UNIQUE_ID_ADDR 0x50 00118 00119 //Definitions for unit selection 00120 #define MPERSPERS 0x00 00121 #define MILLIG 0x01 00122 #define DEG_PER_SEC 0x00 00123 #define RAD_PER_SEC 0x02 00124 #define DEGREES 0x00 00125 #define RADIANS 0x04 00126 #define CENTIGRADE 0x00 00127 #define FAHRENHEIT 0x10 00128 #define WINDOWS 0x00 00129 #define ANDROID 0x80 00130 00131 //Definitions for power mode 00132 #define POWER_MODE_NORMAL 0x00 00133 #define POWER_MODE_LOWPOWER 0x01 00134 #define POWER_MODE_SUSPEND 0x02 00135 00136 //Definitions for operating mode 00137 #define OPERATION_MODE_CONFIG 0x00 00138 #define OPERATION_MODE_ACCONLY 0x01 00139 #define OPERATION_MODE_MAGONLY 0x02 00140 #define OPERATION_MODE_GYRONLY 0x03 00141 #define OPERATION_MODE_ACCMAG 0x04 00142 #define OPERATION_MODE_ACCGYRO 0x05 00143 #define OPERATION_MODE_MAGGYRO 0x06 00144 #define OPERATION_MODE_AMG 0x07 00145 #define OPERATION_MODE_IMUPLUS 0x08 00146 #define OPERATION_MODE_COMPASS 0x09 00147 #define OPERATION_MODE_M4G 0x0A 00148 #define OPERATION_MODE_NDOF_FMC_OFF 0x0B 00149 #define OPERATION_MODE_NDOF 0x0C 00150 00151 typedef struct values 00152 { 00153 int16_t rawx,rawy,rawz; 00154 float x,y,z; 00155 } values; 00156 00157 typedef struct angles 00158 { 00159 int16_t rawroll,rawpitch,rawyaw; 00160 float roll, pitch, yaw; 00161 } angles; 00162 00163 typedef struct quaternion 00164 { 00165 int16_t raww,rawx,rawy,rawz; 00166 float w,x,y,z; 00167 } quaternion; 00168 00169 typedef struct calstatus 00170 { 00171 char raw; // Raw byte from calibration register 00172 int mag; // Bits 0,1 00173 int accel; // Bits 2,3 00174 int gyro; // Bits 4,5 00175 int system; // Bits 6,7 00176 } calstatus; 00177 00178 typedef struct chip 00179 { 00180 char id; 00181 char accel; 00182 char gyro; 00183 char mag; 00184 char sw[2]; 00185 char bootload; 00186 char serial[16]; 00187 } chip; 00188 00189 /** Class for operating Bosch BNO055 sensor over I2C **/ 00190 class BNO055 00191 { 00192 public: 00193 00194 /** Create BNO055 instance **/ 00195 BNO055(PinName SDA, PinName SCL); 00196 //BNO055(I2C& p_i2c); 00197 00198 /** Perform a power-on reset of the BNO055 **/ 00199 void reset(); 00200 /** Check that the BNO055 is connected and download the software details 00201 and serial number of chip and store in ID structure **/ 00202 bool check(); 00203 /** Turn the external timing crystal on/off **/ 00204 void SetExternalCrystal(bool yn); 00205 /** Set the operation mode of the sensor **/ 00206 void setmode(char mode); 00207 /** Set the power mode of the sensor **/ 00208 void setpowermode(char mode); 00209 00210 /** Set the output units from the accelerometer, either MPERSPERS or MILLIG **/ 00211 void set_accel_units(char units); 00212 /** Set the output units from the gyroscope, either DEG_PER_SEC or RAD_PER_SEC **/ 00213 void set_anglerate_units(char units); 00214 /** Set the output units from the IMU, either DEGREES or RADIANS **/ 00215 void set_angle_units(char units); 00216 /** Set the output units from the temperature sensor, either CENTIGRADE or FAHRENHEIT **/ 00217 void set_temp_units(char units); 00218 /** Set the data output format to either WINDOWS or ANDROID **/ 00219 void set_orientation(char units); 00220 /** Set the mapping of the exes/directions as per page 25 of datasheet 00221 range 0-7, any value outside this will set the orientation to P1 (default at power up) **/ 00222 void set_mapping(char orient); 00223 00224 /** Get the current values from the accelerometer **/ 00225 void get_accel(void); 00226 /** Get the current values from the gyroscope **/ 00227 void get_gyro(void); 00228 /** Get the current values from the magnetometer **/ 00229 void get_mag(void); 00230 /** Get the corrected linear acceleration **/ 00231 void get_lia(void); 00232 /** Get the current gravity vector **/ 00233 void get_grv(void); 00234 /** Get the output quaternion **/ 00235 void get_quat(void); 00236 /** Get the current Euler angles **/ 00237 void get_angles(void); 00238 /** Get the current temperature **/ 00239 void get_temp(void); 00240 00241 /** Read the calibration status register and store the result in the calib variable **/ 00242 void get_calib(void); 00243 00244 /** Read the offset and radius values into the calibration array**/ 00245 void read_calibration_data(void); 00246 /** Write the contents of the calibration array into the registers **/ 00247 void write_calibration_data(void); 00248 00249 /** Structures containing 3-axis data for acceleration, rate of turn and magnetic field. 00250 x,y,z are the scale floating point values and 00251 rawx, rawy, rawz are the int16_t values read from the sensors **/ 00252 values accel,gyro,mag,lia,gravity; 00253 00254 /** Stucture containing the Euler angles as yaw, pitch, roll as scaled floating point 00255 and rawyaw, rawroll & rollpitch as the int16_t values loaded from the registers **/ 00256 angles euler; 00257 00258 /** Quaternion values as w,x,y,z (scaled floating point) and raww etc... as int16_t loaded from the 00259 registers **/ 00260 quaternion quat; 00261 00262 /** Current contents of calibration status register **/ 00263 calstatus cal; 00264 00265 /** Contents of the 22 registers containing offset and radius values used as calibration by the sensor **/ 00266 char calibration[22]; 00267 /** Structure containing sensor numbers, software version and chip UID **/ 00268 chip ID; 00269 /** Current temperature **/ 00270 int temperature; 00271 00272 private: 00273 00274 I2C _i2c; 00275 char rx,tx[2],address; //I2C variables 00276 char rawdata[22]; //Temporary array for input data values 00277 char op_mode; 00278 char pwr_mode; 00279 float accel_scale,rate_scale,angle_scale; 00280 int temp_scale; 00281 00282 void readchar(char location) 00283 { 00284 tx[0] = location; 00285 _i2c.write(address,tx,1,true); 00286 _i2c.read(address,&rx,1,false); 00287 } 00288 00289 void writechar(char location, char value) 00290 { 00291 tx[0] = location; 00292 tx[1] = value; 00293 _i2c.write(address,tx,2); 00294 } 00295 00296 void setpage(char value) 00297 { 00298 writechar(BNO055_PAGE_ID_ADDR,value); 00299 } 00300 }; 00301 #endif
Generated on Tue Jul 12 2022 19:18:52 by
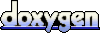