Use this library to upload images to twitpic.com. The library creates and uses its own TCPSocket. Requires EthernetNetIf and DNSResolver. (this library actually designed by a friend of mine, novachild, with no mbed license of his own.)
TwitPic.h
00001 /* 00002 TwitPic.h - TwitPic Image Uploader for mbed 00003 Copyright (c) novachild 2011. All rights reserved. 00004 00005 This library is distributed in the hope that it will be useful, 00006 but WITHOUT ANY WARRANTY; without even the implied warranty of 00007 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. 00008 00009 REQUIRES: EthernetNetIf, DNSResolver, some sort of file access (SD, local, etc) 00010 00011 */ 00012 00013 #ifndef TwitPic_h 00014 #define TwitPic_h 00015 00016 #include "mbed.h" 00017 #include "EthernetNetIf.h" 00018 #include "TCPSocket.h" 00019 #include "host.h" 00020 #include "ipaddr.h" 00021 #include "dnsresolve.h" 00022 00023 class TwitPic { 00024 public: 00025 TwitPic(const char *twitpic_api_key, const char *consumer_key, const char *consumer_secret, const char *access_token, const char *access_token_secret); 00026 int upload(const char *message, 00027 FILE *img, 00028 bool toPost = false); 00029 00030 int uploadAndPost(const char *message, 00031 FILE *imgFile); 00032 00033 private: 00034 Host server; 00035 TCPSocket socket; 00036 00037 00038 void handleTCPSocketEvents(TCPSocketEvent ev); 00039 00040 void sendHeaders(); 00041 void sendParameters(); 00042 void sendImageData(); 00043 void sendFooter(); 00044 void sendNextChunk(); 00045 00046 void getResponse(); 00047 void flushResponse(); 00048 00049 }; 00050 00051 00052 #endif
Generated on Sat Jul 16 2022 03:36:23 by
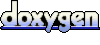