
Dependencies: ChaNFSSD mbed BMP085 SHT2x
NMEA_parse.h
00001 /* 00002 * Copyright (c) 2011 Toshihisa T 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #ifndef _NMEA_PARSE_H 00007 #define _NMEA_PARSE_H 00008 #ifdef __cplusplus 00009 extern "C" { 00010 #endif 00011 00012 #define LIBNMEA_PARSE_NONE (0) 00013 #define LIBNMEA_PARSE_SUMOK (10) 00014 #define LIBNMEA_PARSE_COMPLETE (100) 00015 00016 #define LIBNMEA_NUMSOFARRAY(array) (sizeof((array))/sizeof((array)[0])) 00017 00018 typedef double libNMEA_realnum_t; 00019 typedef long libNMEA_intnum_t; 00020 00021 typedef struct { 00022 // unsigned char isValid; 00023 unsigned char active; /* '0' or '1' = Fix not available,'2' = 2D,'3' = 3D */ 00024 unsigned char positioning; /* 'A' = 単独測位,D = DGPS,N = 無効 */ 00025 } libNMEA_gps_status_t; 00026 00027 typedef struct { 00028 unsigned long res1:1; 00029 unsigned long year:12; 00030 unsigned long mon:4; 00031 unsigned long day:6; 00032 unsigned long res2:9; 00033 } libNMEA_gps_date_t; 00034 00035 typedef struct { 00036 unsigned long res:1; 00037 unsigned long hour:5; 00038 unsigned long min:6; 00039 unsigned long sec:6; 00040 unsigned long msec:14; 00041 } libNMEA_gps_time_t; 00042 00043 typedef struct { 00044 // unsigned char isValid; 00045 unsigned char unit; 00046 libNMEA_realnum_t deg; 00047 } libNMEA_gps_deg_t; 00048 00049 typedef struct { 00050 unsigned char lat_unit; /* 'N'(Nouth) or 'S'(South) */ 00051 unsigned char lon_unit; /* 'W'(West) or 'E'(East) */ 00052 libNMEA_realnum_t latitude; 00053 libNMEA_realnum_t longitude; 00054 } libNMEA_gps_latlon_t; 00055 00056 typedef struct { 00057 // unsigned char isValid; 00058 libNMEA_realnum_t ground; 00059 } libNMEA_gps_speed_t; 00060 00061 typedef struct { 00062 // unsigned char isValid; 00063 libNMEA_realnum_t dop; 00064 } libNMEA_gps_dop_t; 00065 00066 typedef struct { 00067 libNMEA_gps_deg_t trueNorth; 00068 libNMEA_gps_deg_t magnetic; 00069 libNMEA_gps_deg_t mag_dec; 00070 } libNMEA_gps_direction_t; 00071 00072 typedef struct { 00073 libNMEA_gps_dop_t h; 00074 libNMEA_gps_dop_t v; 00075 libNMEA_gps_dop_t p; 00076 } libNMEA_gps_accuracy_t; 00077 00078 typedef struct { 00079 unsigned long status:1; /* これが1ならば,GPS のデータを信用する */ 00080 unsigned long active:1; 00081 unsigned long positioning:1; 00082 unsigned long date:1; 00083 unsigned long timeRMC:1; 00084 unsigned long timeZDA:1; 00085 unsigned long latitude:1; 00086 unsigned long longitude:1; 00087 unsigned long speed:1; 00088 unsigned long direction:1; 00089 unsigned long accuracy_hdop:1; 00090 unsigned long accuracy_vdop:1; 00091 unsigned long accuracy_pdop:1; 00092 unsigned long res:19; 00093 } libNMEA_gps_valid_t; 00094 00095 typedef struct { 00096 libNMEA_gps_valid_t valid; 00097 libNMEA_gps_status_t status; 00098 libNMEA_gps_date_t date; 00099 libNMEA_gps_time_t time; 00100 libNMEA_gps_latlon_t latlon; 00101 libNMEA_gps_speed_t speed; 00102 libNMEA_gps_direction_t direction; 00103 libNMEA_gps_accuracy_t accuracy; 00104 } libNMEA_gps_info_t; 00105 00106 typedef struct { 00107 short cjobst; 00108 unsigned char raw[82+2]; 00109 short rawLength; 00110 unsigned char sum; 00111 unsigned char sumwk; 00112 short argc; 00113 short argv[31]; /* NMEA センテンスで処理できる最大トークン数.メモリを節約したいのでポインタではなく先頭からのオフセットにしている. */ 00114 } libNMEA_data_t; 00115 00116 extern short libNMEA_Parse1Char(unsigned char c,libNMEA_data_t *buffer); 00117 short libNMEA_Parse1Line(libNMEA_data_t *buffer,libNMEA_gps_info_t *GPSinfo); 00118 00119 #ifdef __cplusplus 00120 } 00121 #endif 00122 00123 #endif /* _NMEA_PARSE_H */
Generated on Thu Jul 14 2022 16:50:44 by
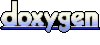