
Dependencies: ChaNFSSD mbed BMP085 SHT2x
FATFileSystem.cpp
00001 /* mbed Microcontroller Library - FATFileSystem 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "FATFileSystem.h" 00006 00007 #include "mbed.h" 00008 00009 #include "FileSystemLike.h" 00010 #include "FATFileHandle.h" 00011 #include "FATDirHandle.h" 00012 #include "ff.h" 00013 //#include "Debug.h" 00014 #include <stdio.h> 00015 #include <stdlib.h> 00016 00017 DWORD get_fattime (void) { 00018 DWORD retval = 0; 00019 time_t seconds = time(NULL); // RTC time. 00020 if(seconds <= 0) 00021 seconds = 999; 00022 struct tm *t = localtime(&seconds); 00023 retval = 0; 00024 retval |= (t->tm_year - 80) << 25; 00025 retval |= (t->tm_mon + 1) << 21; 00026 retval |= (t->tm_mday) << 16; 00027 retval |= (t->tm_hour) << 11; 00028 retval |= (t->tm_min) << 5; 00029 retval |= (t->tm_sec) << 0; 00030 return retval; 00031 } 00032 00033 namespace mbed { 00034 00035 #if FFSDEBUG_ENABLED 00036 static const char *FR_ERRORS[] = { 00037 "FR_OK = 0", 00038 "FR_NOT_READY", 00039 "FR_NO_FILE", 00040 "FR_NO_PATH", 00041 "FR_INVALID_NAME", 00042 "FR_INVALID_DRIVE", 00043 "FR_DENIED", 00044 "FR_EXIST", 00045 "FR_RW_ERROR", 00046 "FR_WRITE_PROTECTED", 00047 "FR_NOT_ENABLED", 00048 "FR_NO_FILESYSTEM", 00049 "FR_INVALID_OBJECT", 00050 "FR_MKFS_ABORTED" 00051 }; 00052 #endif 00053 00054 FATFileSystem *FATFileSystem::_ffs[_DRIVES] = {0}; 00055 00056 FATFileSystem::FATFileSystem(const char* n) : FileSystemLike(n) { 00057 FFSDEBUG("FATFileSystem(%s)\n", n); 00058 for(int i=0; i<_DRIVES; i++) { 00059 if(_ffs[i] == 0) { 00060 _ffs[i] = this; 00061 _fsid = i; 00062 FFSDEBUG("Mounting [%s] on ffs drive [%d]\n", _name, _fsid); 00063 f_mount(i, &_fs); 00064 return; 00065 } 00066 } 00067 error("Couldn't create %s in FATFileSystem::FATFileSystem\n",n); 00068 } 00069 00070 FATFileSystem::~FATFileSystem() { 00071 for(int i=0; i<_DRIVES; i++) { 00072 if(_ffs[i] == this) { 00073 _ffs[i] = 0; 00074 f_mount(i, NULL); 00075 } 00076 } 00077 } 00078 00079 FileHandle *FATFileSystem::open(const char* name, int flags) { 00080 FFSDEBUG("open(%s) on filesystem [%s], drv [%d]\n", name, _name, _fsid); 00081 char n[64]; 00082 sprintf(n, "%d:/%s", _fsid, name); 00083 00084 /* POSIX flags -> FatFS open mode */ 00085 BYTE openmode; 00086 if(flags & O_RDWR) { 00087 openmode = FA_READ|FA_WRITE; 00088 } else if(flags & O_WRONLY) { 00089 openmode = FA_WRITE; 00090 } else { 00091 openmode = FA_READ; 00092 } 00093 if(flags & O_CREAT) { 00094 if(flags & O_TRUNC) { 00095 openmode |= FA_CREATE_ALWAYS; 00096 } else { 00097 openmode |= FA_OPEN_ALWAYS; 00098 } 00099 } 00100 00101 FIL_t fh; 00102 FRESULT res = f_open(&fh, n, openmode); 00103 if(res) { 00104 FFSDEBUG("f_open('w') failed (%d, %s)\n", res, FR_ERRORS[res]); 00105 return NULL; 00106 } 00107 if(flags & O_APPEND) { 00108 f_lseek(&fh, fh.fsize); 00109 } 00110 return new FATFileHandle(fh); 00111 } 00112 00113 int FATFileSystem::remove(const char *filename) { 00114 FRESULT res = f_unlink(filename); 00115 if(res) { 00116 FFSDEBUG("f_unlink() failed (%d, %s)\n", res, FR_ERRORS[res]); 00117 return -1; 00118 } 00119 return 0; 00120 } 00121 00122 int FATFileSystem::format() { 00123 FFSDEBUG("format()\n"); 00124 FRESULT res = f_mkfs(_fsid, 0, 512); // Logical drive number, Partitioning rule, Allocation unit size (bytes per cluster) 00125 if(res) { 00126 FFSDEBUG("f_mkfs() failed (%d, %s)\n", res, FR_ERRORS[res]); 00127 return -1; 00128 } 00129 return 0; 00130 } 00131 00132 DirHandle *FATFileSystem::opendir(const char *name) { 00133 DIR_t dir; 00134 FRESULT res = f_opendir(&dir, name); 00135 if(res != 0) { 00136 return NULL; 00137 } 00138 return new FATDirHandle(dir); 00139 } 00140 00141 int FATFileSystem::mkdir(const char *name, mode_t mode) { 00142 FRESULT res = f_mkdir(name); 00143 return res == 0 ? 0 : -1; 00144 } 00145 00146 } // namespace mbed
Generated on Thu Jul 14 2022 16:50:44 by
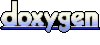