
PROGRAMA PARA HACER SEGUIMIENTO DE FLOTAS CON GPS Y CONEXION A LA RED GPRS
Dependencies: mbed DebouncedIn GPS_G
Dependents: 61_RASTREO_GPRS_copy
main.cpp
00001 /* 00002 PROGRAMA PARA HACER SEGUIMIENTO DE FLOTAS CON GPS Y CONEXION A LA RED GPRS 00003 se pueden simular trayectorias gravando archivos kml en google earth y usando 00004 SatGen 00005 version 2.0, sep 3 / 2019 00006 00007 se asigna un APN segun el servicio GPRS de la SIMCARD (claro Colombia) 00008 se asigna un nombre de host de su pagina web 00009 se asigna el path de los archivos y mapas ejecutables en su servidor 00010 se asigna un ciclo de repeticion de lecturas de GPS. 00011 00012 */ 00013 00014 #include "mbed.h" 00015 #include "stdio.h" 00016 #include "string.h" 00017 #include "GPS.h" 00018 00019 Timer t; 00020 DigitalOut LedVerde(LED2); 00021 DigitalOut LedRojo(LED1); 00022 DigitalOut LedAzul(LED3); 00023 InterruptIn button(PTA13); 00024 00025 00026 float lo,la; 00027 char lon[15], lat[15]; // Cadenas a capturar para latitud y longitud. 00028 char gprsBuffer[20]; 00029 char resp[15]; 00030 Serial GSM(PTE0,PTE1); // Puertos del FRDM para el Módem. 00031 Serial pc(USBTX,USBRX); 00032 GPS gps(PTE22, PTE23); // Puerto del FDRM para el GPS. 00033 int result; 00034 int z=0; 00035 int i=0; 00036 int count=0; 00037 int g=0; 00038 00039 //----------------------------------------------------------------------------------------------------------------------------- 00040 // Esta funcion de abajo lee todo un bufer hasta encontrar CR o LF y el resto lo rellena de 00041 // $, count es lo que va a leer. Lo leido lo mete en buffer que es una cadena previamente definida 00042 // incorpora medida de tiempo si se demora mas de tres segundos retorna fracaso con -1 00043 int readBuffer(char *buffer,int count){ 00044 int i=0; 00045 t.start(); // start timer 00046 while(1) { 00047 while (GSM.readable()) { 00048 char c = GSM.getc(); 00049 if (c == '\r' || c == '\n') c = '$'; 00050 buffer[i++] = c; 00051 if(i > count)break; 00052 } 00053 if(i > count)break; 00054 if(t.read() > 3) { 00055 t.stop(); 00056 t.reset(); 00057 break; 00058 } 00059 } 00060 wait(0.5); 00061 while(GSM.readable()){ // display the other thing.. 00062 char c = GSM.getc(); 00063 } 00064 return 0; 00065 } 00066 //-------------------------------------------------------------------------------------------------------------- 00067 // Esta función de abajo limpia o borra todo un "buffer" de tamaño "count", 00068 // lo revisa elemento por elemento y le mete el caracter null que indica fin de cadena. 00069 // No retorna nada. 00070 void cleanBuffer(char *buffer, int count){ 00071 for(int i=0; i < count; i++) { 00072 buffer[i] = '\0'; 00073 } 00074 } 00075 00076 //-------------------------------------------------------------------------------------------------------------- 00077 // Esta función de abajo envia un comando parametrizado como cadena 00078 // puede ser un comando tipo AT. 00079 void sendCmd(char *cmd){ 00080 GSM.puts(cmd); 00081 } 00082 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00083 // Esta función de abajo espera la respuesta de un comando que debe ser idéntica a la cadena "resp" y un tiempo "timeout", 00084 // si todo sale bien retorna un cero que en la programacion hay que validar, 00085 // si algo sale mal (no se parece o se demora mucho) retorna -1 que debera validarse con alguna expresion logica. 00086 int waitForResp(char *resp, int timeout){ 00087 int len = strlen(resp); 00088 int sum=0; 00089 t.start(); 00090 00091 while(1) { 00092 if(GSM.readable()) { 00093 char c = GSM.getc(); 00094 sum = (c==resp[sum]) ? sum+1 : 0;// esta linea de C# sum se incrementa o se hace cero segun c 00095 if(sum == len)break; //ya acabo se sale 00096 } 00097 if(t.read() > timeout) { // time out chequea el tiempo minimo antes de salir perdiendo 00098 t.stop(); 00099 t.reset(); 00100 return -1; 00101 } 00102 } 00103 t.stop(); // stop timer antes de retornar 00104 t.reset(); // clear timer 00105 while(GSM.readable()) { // display the other thing.. 00106 char c = GSM.getc(); 00107 } 00108 return 0; 00109 } 00110 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00111 // Esta función de abajo es muy completa y útil, se encarga de enviar el comando y esperar la respuesta. 00112 // Si todo sale bien retorna un cero (herencia de las funciones contenedoras) que en la programacion hay que validar 00113 // con alguna expresion lógica. 00114 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout){ 00115 sendCmd(cmd); 00116 return waitForResp(resp,timeout); 00117 } 00118 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00119 // Esta función de abajo chequea que el módem este vivo, envia AT, le contesta con OK y espera 2 segundos. 00120 int powerCheck(void){ // Este comando se manda para verificar si el módem esta vivo o conectado. 00121 return sendCmdAndWaitForResp("AT\r\n", "OK", 2); 00122 } 00123 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00124 // Esta función de abajo chequea el estado de la sim card 00125 // y si todo sale bien retorna un cero que en la programacion hay que validar 00126 // con alguna expresión lógica. 00127 int checkSIMStatus(void){ 00128 char gprsBuffer[30]; 00129 int count = 0; 00130 cleanBuffer(gprsBuffer, 30); 00131 while(count < 3){ 00132 sendCmd("AT+CPIN?\r\n"); 00133 readBuffer(gprsBuffer,30); 00134 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))){ 00135 break; 00136 } 00137 count++; 00138 wait(1); 00139 } 00140 00141 if(count == 3){ 00142 return -1; 00143 } 00144 return 0; 00145 } 00146 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00147 00148 // Esta función de abajo chequea la calidad de la señal 00149 // y si todo sale bien retorna con el valor de señal útil o un -1 si no es aceptable, en la programacion hay que validar 00150 // con alguna expresión lógica. 00151 int checkSignalStrength(void){ 00152 char gprsBuffer[100]; 00153 int index, count = 0; 00154 cleanBuffer(gprsBuffer,100); 00155 while(count < 3){ 00156 sendCmd("AT+CSQ\r\n"); 00157 readBuffer(gprsBuffer,25); 00158 if(sscanf(gprsBuffer, "AT+CSQ$$$$+CSQ: %d", &index)>0) { 00159 break; 00160 } 00161 count++; 00162 wait(1); 00163 } 00164 if(count == 3){ 00165 return -1; 00166 } 00167 return index; 00168 } 00169 00170 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00171 00172 // detectar direcion ip 00173 int ip_detect(){ 00174 if (GSM.readable()){ 00175 readBuffer(gprsBuffer,15); 00176 for(i=0;i<15;i++) 00177 { 00178 resp[i]=gprsBuffer[i]; 00179 } 00180 cleanBuffer(gprsBuffer,20); 00181 for(i=0;i<15;i++){ 00182 if(resp[i]== '.'){ 00183 z++; 00184 } 00185 } 00186 if(z==3){ //CUENTO TRES PUNTOS LO MAS PROBABLE ES QUE SEA UNA DIRECCION ip 00187 pc.printf("llego ip=%d\r\n",z); 00188 z=0; 00189 return 0; //RETORNA CON CERO SI LLEGARON TRES PUNTOS 00190 } 00191 }//fin check GSM modem 00192 z=0; 00193 return -1; //NO LLEGARON 3 PUNTOS RETORNA CON -1 00194 }//fin funcion 00195 00196 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00197 // Esta funcion de abajo inicia la comunicacion GPRS con la red celular, Se compone de un grupo de subfunciones ya definidas previamente 00198 // envia las secuencias de forma ordenada esparando la respuesta adecuada 00199 // si todo sale bien retorna un cero que en la programacion hay que validar 00200 // con alguna expresión lógica. 00201 //********************************************************************************************************* 00202 00203 int init_gprs(void){ 00204 00205 if (0 != sendCmdAndWaitForResp("AT\r\n", "OK", 5)){ 00206 return -1; 00207 } 00208 if (0 != sendCmdAndWaitForResp("ATE0\r\n", "OK", 5)){ //sin eco 00209 return -1; 00210 } 00211 if (0 != sendCmdAndWaitForResp("AT+CGATT=1\r\n", "OK", 5)){//inicia conexion GPRS 00212 return -1; 00213 } 00214 if (0 != sendCmdAndWaitForResp("AT+CSTT=internet.comcel.com.co,comcel,comcel\r\n", "OK", 5)){ //set apn 00215 return -1; 00216 } 00217 if (0 != sendCmdAndWaitForResp("AT+CIICR\r\n", "OK", 5)){ //habilitar conexion inalambrica 00218 return -1; 00219 } 00220 00221 cleanBuffer(gprsBuffer,25); 00222 sendCmd("AT+CIFSR\r\n"); //obtener direccion ip 00223 if(0 != ip_detect()){ //esperar la llegada de un direccion IP 00224 return -1; 00225 } 00226 wait(1); 00227 LedVerde=0;//CONEXION OK... PRENDE LED VERDE.. 00228 return 0; 00229 } 00230 00231 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00232 //ESTA FUNCION DE ABAJO CIERRA UNA CONEXION GPRS 00233 00234 int end_gprs(void){ 00235 if (0 != sendCmdAndWaitForResp("AT+CIPSHUT\r\n", "OK", 5)){ 00236 return -1; 00237 } 00238 if (0 != sendCmdAndWaitForResp("AT+CGATT=0\r\n", "SHUT OK", 5)){ 00239 return -1; 00240 } 00241 LedVerde=0; 00242 LedRojo=1; 00243 return 0; 00244 } 00245 00246 //++++++++++++++++++++++++++++++++++++++++++++++++++++ 00247 //interupcion perdida de energia 00248 //++++++++++++++++++++++++++++++++++++++++++++++++++++ 00249 00250 void off_gprs(){ 00251 end_gprs(); 00252 } 00253 00254 //+++++++++++++++++++++++++++++++++++++++++++++++++++++ 00255 //inicio del programa principal 00256 //+++++++++++++++++++++++++++++++++++++++++++++++++++++ 00257 int main(){ 00258 00259 loop1:g=gps.sample(); //GPS bien conectado???? de primero antes que todo!!!! 00260 if(g){ 00261 LedAzul=0;//prende led azul GPS ok!!! 00262 pc.printf("GPS--OK\n\r"); 00263 //si no hay GPS no intentar dada, ni conexion ni envio de datos y esperar el GPS 00264 goto lop1; 00265 } 00266 goto loop1; //chequeo infinito de un GPS bien instalado... 00267 lop1: 00268 if(init_gprs()<0){ 00269 LedRojo=0;//PRENDE ROJO, APAGA VERDE 00270 LedVerde=1; 00271 goto lop1;//NO SE PUEDE RECONECTAR INFINITAMENTE ESTE SALTO ES PROVISIONAL 00272 //CONTAR LOS INTENTOS Y DAR SEÑAL DE ERROR PERMANENTE 00273 } 00274 button.fall(&off_gprs);//perdida de alimentacion,apagaron carro ejecuta interupcion 00275 //que desconecta la conexion GPRS 00276 while(1){ //si el GPS tiene conexion y datos se envian coordenadas a pagina web 00277 LedAzul=1; //APAGAMOS LED AZUL 00278 if(gps.sample()){ //UN GPS RESPONDE! 00279 LedAzul=0; 00280 lo = gps.longitude; 00281 la = gps.latitude; 00282 sprintf (lon, "%f", lo);//pasa de flotante a caracter 00283 sprintf (lat, "%f", la);//pasa de flotante a caracter 00284 cleanBuffer(gprsBuffer,10); 00285 00286 envio1: 00287 GSM.printf("AT+CIPSTART=\"TCP\",\"unrobotica.com\",\"80\"\r\n"); 00288 //respuesta a este comando debe ser "CONNECT OK" 00289 wait(6);//espero un poquito a que conteste y luego leo el buffer 00290 if (GSM.readable()){ 00291 readBuffer(gprsBuffer,20); 00292 pc.printf("%s\r\n",gprsBuffer); 00293 for(i=8;i<18;i++) 00294 { 00295 resp[i]=gprsBuffer[i]; 00296 } 00297 if(strcmp("CONNECT OK",resp) == 0){ 00298 goto envio2; 00299 } 00300 goto envio1; //no llego connect ok 00301 } 00302 goto envio1;//se repite comando 1. si no encontro respuesta 00303 envio2: 00304 if(0 = sendCmdAndWaitForResp("AT+CIPSEND\r\n","",5)){ //devuelve control+Z 00305 goto envio3; 00306 } 00307 goto envio2;//no llego control Z volver a enviar comando AT 00308 envio3: 00309 GSM.printf("GET /gpstracker/gps1.php?lat=%s&lon=%s HTTP/1.0\r\n",lat,lon); 00310 wait(3); 00311 envio4: 00312 GSM.printf("Host: unrobotica.com\n\n"); 00313 wait(3); 00314 envio5: 00315 GSM.printf("\r\n"); 00316 wait(3); 00317 envio6: 00318 GSM.printf("\n\r"); 00319 wait(6);//espero un poquito a que conteste y luego leo el buffer 00320 if (GSM.readable()){ 00321 readBuffer(gprsBuffer,12); 00322 pc.printf("%s\r\n",gprsBuffer); 00323 for(i=2;i<9;i++) 00324 { 00325 resp[i]=gprsBuffer[i]; //send ok 00326 } 00327 if(strcmp("SEND OK",resp) == 0){ 00328 wait(50); 00329 goto envio2;//reenviar mas datos, todo salio bien 00330 00331 } 00332 if(end_gprs()=0){ //desconexion correcta reconectar de nuevo todo 00333 goto lop1; 00334 } 00335 goto lop1; 00336 00337 00338 00339 00340 00341 00342 00343 00344 ................ 00345 if(0 !=sendCmdAndWaitForResp("\n\r","SEND OK",10)) 00346 { 00347 00348 } 00349 //mas tarde devuelve SEND OK ...esto es suficiente para indicarnos que los datos se fueron a la nube 00350 //cleanBuffer(gprsBuffer,20); 00351 //leer bufer y encontrar respuesta exitosa devuelve "SEND OK" 00352 // GSM.printf("AT+CIPSTART=\"TCP\",\"unrobotica.com\",\"80\"\r\n"); 00353 // pc.printf("AT+CIPSTART=\"TCP\",\"unrobotica.com\",\"80\"\r\n"); 00354 //readBuffer(gprsBuffer,10); 00355 //if((NULL = strstr(gprsBuffer,""))){ 00356 // wait(1); 00357 // goto repetir2; 00358 LedRojo=1; 00359 LedVerde=0; //envio OK 00360 }// del test del gps 00361 LedRojo=0; // hay problemas 00362 LedVerde=1; 00363 00364 }//del while col 13 00365 00366 }//del main col 11 00367 00368 00369 00370 00371
Generated on Wed Jul 27 2022 15:29:29 by
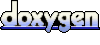