
RASTREADOR SATELITAÑ GSM
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00002 // Programa para establecer la comunicación con un módem Siemens A56 y un modulo STM32F103 BLUEPILL CHINO. 00003 // opera como rastreador satelital para Geolocalizacion. 00004 // Por la UART (1) se conecta el MODEM y por la uart (2) el GPS (se lee en modo NEMEA) 00005 // Este sistema genera una cadena de geolocalizacion para GoogleMaps con las coordenadas locales 00006 // Si previamente se envia el mensaje (Coordenadas o coordenadas) 00007 // El sistema ademas recibe ordenes de tipo mensaje GSM PDU para accionar cargas 00008 // 1----Una supuesta valvula de combustible (On y Off.....on y off......) 00009 // 2----Una cantonera para cerradura electrica (Pulso o pulso) (pulso de 7 segundos) 00010 // Este sistema responde con un mensaje si el mensaje fue recibido (Mensaje Recibido) 00011 // 00012 // Adicionalmente este sistema mide un valor analogico en respuesta al mensaje..(Voltaje o voltaje) 00013 // El sistema dispone de un jumper que permite operar el sistema con o sin GPS (jumper a tierra) 00014 // El sistema detecta si el modem GSM esta bien conectado configurado y respondiendo correctamente 00015 // El sistema detecta si el GPS emite cadenas NEMEA y señaliza con un led si es exitosa la conexion 00016 // Presenta borrado automatico de SMS entrantes para evitar perdida de sincronismo en la deteccion 00017 // de cadenas y prefijos. 00018 // Este codigo compila sin problemas para un modulo chino bluepill STM32F103 00019 // con las modificaciones adecuadas de puertos y leds 00020 00021 //+++++++++++++++++++++++++++++++ARCHIVOS INCLUIDOS************************************************************************* 00022 #include "mbed.h" 00023 #include "DebouncedIn.h" 00024 #include "stdio.h" 00025 #include "string.h" 00026 #include "GPS.h" 00027 00028 Timer t; 00029 //++++++++++++++++++++++++++++++++++++salidas y entradas digitales+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00030 DigitalOut LedVerde(PA_8); 00031 DigitalOut LedRojo(PB_15); 00032 DigitalOut LedAzul(PB_14); 00033 DigitalOut valvula(PB_13);//salida de la valvula 00034 DigitalOut puerta(PB_12);//salida de cerradura magnetica (en caso de usarlo en casa o desbloquear puertas de carro) 00035 DigitalIn sin_gps(PA_11); 00036 00037 // Entrada análoga 00038 AnalogIn v(PA_0); 00039 float medi; 00040 00041 // Declaración de los puertos de la FRDM, Módem GSM y GPS. 00042 Serial GSM(PB_6,PB_7); // Puertos del FRDM para el Módem. 00043 Serial pc(PB_10,PB_11); 00044 GPS gps(PA_9,PA_10); // Puerto del FDRM para el GPS. 00045 00046 // Declaración de variables 00047 // Cadenas de caracteres con las que se va a trabajar. 00048 char DE1[255]; 00049 char DS1[255]; 00050 char DE2[255]; 00051 char DS2[255]; 00052 char buffer[512]; 00053 char resp[6]; 00054 char tam[2]; 00055 char mensaje[100]; 00056 00057 //Variables enteras y caracteres 00058 int g=0; 00059 int count; 00060 int i, K, LENOUT1, LENIN1, LENOUT2, LENIN2, C; 00061 int c=0; 00062 char r[]=""; 00063 char msg[256]; 00064 char char1; 00065 int ind; 00066 float med; 00067 char outmed[16], outmedn[16]; 00068 int ret = 1; 00069 00070 // Adquisición de números de teléfono, emisor - receptor 00071 char tel[15]; 00072 00073 // El GPS entregará al celular coordenadas expresadas en latitud y longitud 00074 // según la ubicación que encuentre, por lo tanto se declaran estas variables. 00075 float lo,la; 00076 char clo[255], cla[255]; // Cadenas a capturar para latitud y longitud. 00077 char la_lo[255], volt[255]; 00078 00079 // Cadena de google maps 00080 char http2[255]; 00081 char http[] = "http://maps.google.com/maps?q="; 00082 char buf[100]; 00083 00084 // Relleno de datos propio del protocolo de SMS. 00085 char relle1[] = "0011000A91"; 00086 char relle2[] = "0000AA"; 00087 00088 // Reverses a string 'str' of length 'len' 00089 // driver program to test above funtion. 00090 void reverse(char *str, int len) 00091 { 00092 int i=0, j=len-1, temp; 00093 while (i<j) 00094 { 00095 temp = str[i]; 00096 str[i] = str[j]; 00097 str[j] = temp; 00098 i++; j--; 00099 } 00100 } 00101 00102 // Converts a given integer x to string str[]. d is the number 00103 // of digits required in output. If d is more than the number 00104 // of digits in x, then 0s are added at the beginning. 00105 int intToStr(int x, char str[], int d) 00106 { 00107 int i = 0; 00108 while (x) 00109 { 00110 str[i++] = (x%10) + '0'; 00111 x = x/10; 00112 } 00113 00114 // If number of digits required is more, then 00115 // add 0s at the beginning 00116 while (i < d) 00117 str[i++] = '0'; 00118 00119 reverse(str, i); 00120 str[i] = '\0'; 00121 return i; 00122 } 00123 00124 // Converts a floating point number to string. 00125 void ftoa(float n, char *res, int afterpoint) 00126 { 00127 // Extract integer part 00128 int ipart = (int)n; 00129 00130 // Extract floating part 00131 float fpart = n - (float)ipart; 00132 00133 // convert integer part to string 00134 int i = intToStr(ipart, res, 0); 00135 00136 // check for display option after point 00137 if (afterpoint != 0) 00138 { 00139 res[i] = '.'; // add dot 00140 00141 // Get the value of fraction part upto given no. 00142 // of points after dot. The third parameter is needed 00143 // to handle cases like 233.007 00144 float fp=10; 00145 fpart =fpart * pow(fp,afterpoint); 00146 00147 intToStr((int)fpart, res + i + 1, afterpoint); 00148 } 00149 } 00150 //++++++++++++++++++++++++++++++++++++libreria para vaciar el modem+++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00151 void FlushGSM(void) { 00152 char1 = 0; 00153 while (GSM.readable()){ 00154 char1 = GSM.getc(); 00155 } 00156 return; 00157 } 00158 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00159 void callback(){ 00160 // Note: you need to actually read from the serial to clear the RX interrupt 00161 pc.printf("%c\n", GSM.getc()); 00162 } 00163 //----------------------------------------------------------------------------------------------------------------------------- 00164 // Esta funcion de abajo lee todo un bufer hasta encontrar CR o LF y el resto lo rellena de 00165 // $, count es lo que va a leer. Lo leido lo mete en buffer que es una cadena previamente definida 00166 // incorpora medida de tiempo si se demora mas de tres segundos retorna fracaso con -1 00167 int readBuffer(char *buffer,int count){ 00168 int i=0; 00169 t.start(); // start timer 00170 while(1) { 00171 while (GSM.readable()) { 00172 char c = GSM.getc(); 00173 if (c == '\r' || c == '\n') c = '$'; 00174 buffer[i++] = c; 00175 if(i > count)break; 00176 } 00177 if(i > count)break; 00178 if(t.read() > 3) { 00179 t.stop(); 00180 t.reset(); 00181 break; 00182 } 00183 } 00184 wait(0.5); 00185 while(GSM.readable()){ // display the other thing.. 00186 char c = GSM.getc(); 00187 } 00188 return 0; 00189 } 00190 //-------------------------------------------------------------------------------------------------------------- 00191 // Esta función de abajo limpia o borra todo un "buffer" de tamaño "count", 00192 // lo revisa elemento por elemento y le mete el caracter null que indica fin de cadena. 00193 // No retorna nada. 00194 void cleanBuffer(char *buffer, int count){ 00195 for(int i=0; i < count; i++) { 00196 buffer[i] = '\0'; 00197 } 00198 } 00199 //-------------------------------------------------------------------------------------------------------------- 00200 // Esta función de abajo envia un comando parametrizado como cadena 00201 // puede ser un comando tipo AT. 00202 void sendCmd(char *cmd){ 00203 GSM.puts(cmd); 00204 } 00205 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00206 // Esta función de abajo espera la respuesta de un comando que debe ser idéntica a la cadena "resp" y un tiempo "timeout", 00207 // si todo sale bien retorna un cero que en la programacion hay que validar, 00208 // si algo sale mal (no se parece o se demora mucho) retorna -1 que debera validarse con alguna expresion logica. 00209 int waitForResp(char *resp, int timeout){ 00210 int len = strlen(resp); 00211 int sum=0; 00212 t.start(); 00213 00214 while(1) { 00215 if(GSM.readable()) { 00216 char c = GSM.getc(); 00217 sum = (c==resp[sum]) ? sum+1 : 0;// esta linea de C# sum se incrementa o se hace cero segun c 00218 if(sum == len)break; //ya acabo se sale 00219 } 00220 if(t.read() > timeout) { // time out chequea el tiempo minimo antes de salir perdiendo 00221 t.stop(); 00222 t.reset(); 00223 return -1; 00224 } 00225 } 00226 t.stop(); // stop timer antes de retornar 00227 t.reset(); // clear timer 00228 while(GSM.readable()) { // display the other thing.. 00229 char c = GSM.getc(); 00230 } 00231 return 0; 00232 } 00233 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00234 // Esta función de abajo es muy completa y útil, se encarga de enviar el comando y esperar la respuesta. 00235 // Si todo sale bien retorna un cero (herencia de las funciones contenedoras) que en la programacion hay que validar 00236 // con alguna expresion lógica. 00237 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout){ 00238 sendCmd(cmd); 00239 return waitForResp(resp,timeout); 00240 } 00241 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00242 // Esta función de abajo chequea que el módem este vivo, envia AT, le contesta con OK y espera 2 segundos. 00243 int powerCheck(void){ // Este comando se manda para verificar si el módem esta vivo o conectado. 00244 return sendCmdAndWaitForResp("AT\r\n", "OK", 2); 00245 } 00246 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00247 // Esta función de abajo chequea el estado de la sim card 00248 // y si todo sale bien retorna un cero que en la programacion hay que validar 00249 // con alguna expresión lógica. 00250 int checkSIMStatus(void){ 00251 char gprsBuffer[30]; 00252 int count = 0; 00253 cleanBuffer(gprsBuffer, 30); 00254 while(count < 3){ 00255 sendCmd("AT+CPIN?\r\n"); 00256 readBuffer(gprsBuffer,30); 00257 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))){ 00258 break; 00259 } 00260 count++; 00261 wait(1); 00262 } 00263 00264 if(count == 3){ 00265 return -1; 00266 } 00267 return 0; 00268 } 00269 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00270 // Esta función de abajo chequea la calidad de la señal 00271 // y si todo sale bien retorna con el valor de señal útil o un -1 si no es aceptable, en la programacion hay que validar 00272 // con alguna expresión lógica. 00273 int checkSignalStrength(void){ 00274 char gprsBuffer[100]; 00275 int index, count = 0; 00276 cleanBuffer(gprsBuffer,100); 00277 while(count < 3){ 00278 sendCmd("AT+CSQ\r\n"); 00279 readBuffer(gprsBuffer,25); 00280 if(sscanf(gprsBuffer, "AT+CSQ$$$$+CSQ: %d", &index)>0) { 00281 break; 00282 } 00283 count++; 00284 wait(1); 00285 } 00286 if(count == 3){ 00287 return -1; 00288 } 00289 return index; 00290 } 00291 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00292 // Esta funcion de abajo inicaliza el módem. Se compone de un grupo de subfunciones ya definidas previamente 00293 // primero chequea que este vivo, 00294 // segundo chequea el estado de la simcard, 00295 // tercero chequea la intencidad de señal celular, 00296 // cuarto aplica la configuracion 00297 // y si todo sale bien retorna un cero que en la programacion hay que validar 00298 // con alguna expresión lógica. 00299 int init(){ 00300 if (0 != sendCmdAndWaitForResp("AT\r\n", "OK", 3)){ 00301 return -1; 00302 } 00303 if (0 != sendCmdAndWaitForResp("AT+CNMI=1,1\r\n", "OK", 3)){ 00304 return -1; 00305 } 00306 if (0 != sendCmdAndWaitForResp("AT+CMGF=0\r\n", "OK", 3)){ 00307 return -1; 00308 } 00309 if (0 != sendCmdAndWaitForResp("AT+CBST=7,0,1\r\n", "OK", 3)){ //velocidad fija a 9600, modem asincronico no transparente 00310 return -1; 00311 } 00312 if (0 != sendCmdAndWaitForResp("ATE\r\n", "OK", 3)){ //se le quita el eco al modem GSM 00313 return -1; 00314 } 00315 LedVerde=0; 00316 return 0; 00317 } 00318 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00319 // Esta funcion de abajo intenta leer un mensaje de texto en formato PDU o HEX 00320 // y si todo sale bien retorna un cero que en la programacion hay que validar 00321 // con alguna expresión lógica. 00322 int readSMSpdu(char *message, int index){ 00323 int i = 0; 00324 char gprsBuffer[100]; 00325 char *p,*s; 00326 GSM.printf("AT+CMGR=%d\r\n",index); 00327 cleanBuffer(gprsBuffer,100); 00328 readBuffer(gprsBuffer,100); 00329 if(NULL == ( s = strstr(gprsBuffer,"+CMGR"))) { 00330 return -1; 00331 } 00332 if(NULL != ( s = strstr(gprsBuffer,"+32"))) { 00333 p = s + 6; 00334 while((*p != '$')&&(i < 5)) { 00335 message[i++] = *(p++); 00336 } 00337 message[i] = '\0'; 00338 } 00339 return 0; 00340 } 00341 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00342 // Esta función de abajo borra mensajes SMS del modem 00343 // y si todo sale bien retorna un cero que en la programacion hay que validar 00344 // con alguna expresion logica. 00345 int deleteSMS(int index){ 00346 char cmd[32]; 00347 snprintf(cmd,sizeof(cmd),"AT+CMGD=%d\r\n",index); 00348 sendCmd(cmd); 00349 return 0; 00350 } 00351 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00352 // Esta función devuelve la confirmacion del mensaje a quien lo envio. 00353 int recibe_ok(){ 00354 pc.printf("AT+CMGS=27\n\r"); 00355 GSM.printf("AT+CMGS=27\n\r"); 00356 wait(1); 00357 GSM.printf("0011000A91%s0000AA10CDB27B1E569741F2F2382D4E93DF",tel); 00358 GSM.printf("\n\r"); 00359 pc.printf("0011000A91%s0000AA10CDB27B1E569741F2F2382D4E93DF\n\r",tel); 00360 pc.printf("\n\r");//control+Z 00361 wait(1); 00362 return 0; 00363 } 00364 //******************************************************************************************************************************* 00365 // Programas a ejecutar. 00366 int main(){ 00367 //configuramos los puertos seriales 00368 GSM.baud(9600);//configura los baudios de la FRDMKL25Z en 9600 00369 GSM.format(8,Serial::None,1); //configura el formato de los datos de la UART 00370 LedVerde = 1; // APAGO LOS LEDS 00371 LedRojo = 1; 00372 LedAzul = 1; 00373 LedRojo = 0; // PRENDO EL LED ROJO 00374 valvula=1; // se enciende la valvula por defecto 00375 00376 //****************** CONFIGURACIÓN DEL MODEM GSM (TELEFONO CELULAR SIEMENS A56i). 00377 inicio1: 00378 ret = init(); 00379 if(ret==0){ // esta bien conectado el modem GSM......................................................................... 00380 LedRojo = 1; // apagar led rojo 00381 LedVerde = 0; // Enciende LED Verde para confirmar la comunicación OK con el módem. 00382 pc.printf("Modem configurado\n");//envia mensaje a la terminal........................................................ 00383 } 00384 else{ 00385 wait(1); 00386 goto inicio1; // se hace un reintento de conexion o el sistema no funcionara 00387 } 00388 00389 //Luego se verifica GPS bien conectado (si esta habilitado por dip sw)y recibiendo satelites 00390 if(!sin_gps){ 00391 pc.printf("SIN GPS\n\r");//confirma con teminal que no se habilito el gps 00392 goto seguir9;// de inmediato funcionara sin gps 00393 } 00394 //*****************************************Test para un GPS bien instalado*********************************************** 00395 00396 //******************************************esta bien el GPS***************************************************************** 00397 pc.printf("CON GPS\n\r"); 00398 g=gps.sample(); 00399 if(g){ 00400 LedAzul=0; 00401 pc.printf("GPS--OK\n\r"); 00402 } 00403 00404 seguir9: 00405 00406 //**********************inicia codigo ciclico********************************************** 00407 //**********************inicia codigo ciclico********************************************** 00408 //**********************inicia codigo ciclico********************************************** 00409 00410 //****************DESDE ACA SE LEE CUALQUIER MENSAJE SMS ENTRANTE************************** 00411 while(1){ 00412 if (GSM.readable()){ 00413 readBuffer(buffer,110); 00414 pc.printf("%s\r\n",buffer); 00415 for(i=0; i<5; i++){ 00416 resp[i] = buffer[i]; 00417 } 00418 00419 pc.printf("%s\r\n", resp); 00420 if(strcmp("$$+CM", resp) == 0){ //COMPARA resp con "+CMTI" 00421 pc.printf("Llego MSG\r\n"); 00422 cleanBuffer(buffer,10); 00423 GSM.printf("AT+CMGL=0\r\n"); // Envío comando para leer mensaje 00424 pc.printf("AT+CMGL=0\r\n"); 00425 //GSM.printf("AT+CMGD=0\r\n"); // Envío comando para borrar el mensaje. 00426 readBuffer(buffer,110); 00427 pc.printf("%s\r\n",buffer); 00428 00429 // Lectura el teléfono emisor 00430 for(i=0; i<10; i++){ 00431 tel[i] = buffer[i+40]; 00432 } 00433 pc.printf("Telefono: %c%c%c%c%c%c%c%c%c%c\r\n", tel[1], tel[0], tel[3], tel[2], tel[5], tel[4], tel[7], tel[6], tel[9], tel[8]); 00434 00435 // Lectura del tamaño 00436 for(i=0;i<2;i++){ 00437 tam[i] = buffer[i + 68]; 00438 } 00439 pc.printf("%s-\r\n", tam); 00440 00441 // Lectura del mensaje 00442 for(i=0;i<26;i++){ 00443 msg[i] = buffer[i+70]; // Lee un mensaje de 26 caracteres máximo desde la posición 70 del buffer. 00444 } 00445 pc.printf("%s-\r\n", msg); 00446 00447 // Decodificación del mensaje 00448 00449 00450 // Comparar el mensaje 00451 deleteSMS(1); // Se borran los mensajes por medio de una función 00452 readBuffer(buffer, 110); 00453 //*************************SEFGUNDO CASO ACTIVAR SALIDAS************************************ } 00454 //.................activar valvula de combustible...............On..on....................... 00455 if((strncmp("4F37", msg, 4) == 0) || (strncmp("6F37", msg, 4) == 0)){ 00456 recibe_ok(); 00457 valvula = 1; // Encender valvula. 00458 cleanBuffer(buffer, 110); 00459 00460 } 00461 // ...................................................... off y Off........................ 00462 if((strncmp("4FB319", msg, 6) == 0) || (strncmp("6FB319", msg, 6) == 0)){ 00463 recibe_ok(); 00464 valvula = 0; // apagar valvula. 00465 cleanBuffer(buffer, 110); 00466 } 00467 00468 //............................Envia un Pulso Para Puerta Pulso...D03A7BFE06 pulso.F03A7BFE06.............. 00469 if((strncmp("D03A7BFE06", msg, 10) == 0) || (strncmp("F03A7BFE06", msg, 10) == 0)){ 00470 recibe_ok();//CONFIRMACION DE RECEPCION REMOTA 00471 puerta = 1; // Encender Puerta. 00472 wait(7);//pulso de 7 segundos 00473 puerta = 0;//apaga puerta 00474 cleanBuffer(buffer, 110); 00475 } 00476 00477 // COMPARA resp con "E3F75B4E2EBBC3E4F01C" que es "coordenadas", o "C3F75B4E2EBBC3E4F01C" que es "Coordenadas". 00478 if((strncmp("E3F75B4E2EBBC3E4F01C", msg, 20) == 0) || (strncmp("C3F75B4E2EBBC3E4F01C", msg, 20) == 0)){ 00479 recibe_ok(); 00480 LedVerde = 1; // Encender LED azul. 00481 LedAzul = 0; 00482 wait(2); 00483 00484 if(gps.sample()){ 00485 lo = gps.longitude; 00486 la = gps.latitude; 00487 pc.printf("\nLongitud entera = %f, Latitud entera = %f\n", lo, la); 00488 //wait(0.5); 00489 00490 //LONGITUD 00491 sprintf (clo, "%f", lo); 00492 pc.printf ("\nLongitud = %s\n",clo); 00493 //wait(0.5); 00494 00495 // LATITUD 00496 sprintf (cla, "%f", la); 00497 pc.printf ( "\nLatitud = %s\n",cla); 00498 //wait(0.5); 00499 00500 // Concatenando las cadenas de Latitud y Longitud 00501 strcpy(la_lo,cla); 00502 strcat(la_lo,","); 00503 strcat(la_lo,clo); 00504 pc.printf("\nLatitud, Longitud: %s\n",la_lo); 00505 00506 //Ahora se juntan las cadenas obtenidas y se agrega el protocolo de transferencia de hipertextos http 00507 strcpy(DE1,http); 00508 strcat(DE1,la_lo); 00509 pc.printf("\nDireccion: %s\n",DE1); 00510 pc.printf("\n"); 00511 LENIN1 = strlen(DE1); 00512 00513 //Conversión a octetos. 00514 K = 0; 00515 C = 0; 00516 for (i = 0; i < LENIN1; i++){ 00517 DS1[i] = DE1[i + C] >> K | DE1[i + C + 1] << (7 - K); 00518 if(DS1[i] == 0x00) {LENOUT1 = i; goto salir1;} 00519 K++; 00520 if (K == 7) {K = 0; C++;} // se chequea que ya se acabaron los bits en un ciclo de conversion. 00521 } 00522 00523 salir1: 00524 for (i = 0; i < LENIN1; i++){ 00525 pc.printf("%X", DE1[i]); 00526 } 00527 00528 pc.printf(":\r\n"); 00529 for (i = 0; i < LENOUT1; i++){ 00530 pc.printf("%2X", DS1[i]&0x000000FF); 00531 } 00532 pc.printf("\r\nLENOUT GPS: %d, LENIN GPS: %2X\r\n", LENOUT1, LENIN1); 00533 00534 // Concatenación del mensaje en formato PDU y envío del mismo. 00535 ind = 14 + LENOUT1 - 1; 00536 00537 GSM.printf("AT+CMGS=%d\r\n",ind); 00538 pc.printf("AT+CMGS=%d\r\n",ind); 00539 pc.printf(relle1); 00540 GSM.printf(relle1); 00541 00542 for (i=0 ;i<=9; i++) { 00543 pc.printf("%c",tel[i]); 00544 GSM.printf("%c",tel[i]); 00545 } 00546 00547 pc.printf(relle2); 00548 GSM.printf(relle2); 00549 pc.printf("%2X", LENIN1); 00550 GSM.printf("%2X", LENIN1); 00551 00552 for (i = 0; i < LENOUT1; i++){ 00553 pc.printf("%02X", DS1[i]&0x000000FF); 00554 GSM.printf("%02X", DS1[i]&0x000000FF); 00555 } 00556 wait(1); 00557 GSM.putc((char)0x1A); // Ctrl - Z. 00558 GSM.scanf("%s",buf); // Estado del mensaje (Envió o Error). 00559 pc.printf(">%s\n",buf); 00560 pc.printf("\n"); 00561 } 00562 00563 wait(2); 00564 LedAzul = 1; 00565 LedVerde = 0; 00566 GSM.printf("AT+CMGD=0\r\n"); // Borra el mensaje actual (Posición "0"). 00567 //pc.printf("\n%s\n\n", "Borro mensaje"); 00568 } 00569 00570 // COMPARA resp con "F6379B1E569701" que es "voltaje", o "F6379B1E569701" que es "Voltaje". 00571 if((strncmp("F6379B1E569701", msg, 14) == 0) || (strncmp("D6379B1E569701", msg, 14) == 0)){ 00572 //recibe_ok(); 00573 LedRojo = 0; // Encender LED amarillo. 00574 LedVerde = 0; 00575 LedAzul = 1; 00576 wait(2); 00577 00578 med = v.read()*3.3; 00579 medi = v.read(); 00580 pc.printf("\n%f\n", medi); 00581 00582 cleanBuffer(outmed, 16); 00583 if (med < 1){ // Se convierte la Medida a caracter. 00584 strcat(outmed, "0"); 00585 ftoa(med, outmedn, 5); 00586 strcat(outmed, outmedn); 00587 } 00588 else{ 00589 ftoa(med, outmed, 5); 00590 } 00591 strcpy(DE2,"Voltaje: "); 00592 strcat(DE2,outmed); 00593 pc.printf("\n%s\n\n", DE2); 00594 LENIN2 = strlen(DE2); 00595 00596 //Conversión a octetos. 00597 K = 0; 00598 C = 0; 00599 for (i = 0; i < LENIN2; i++){ 00600 DS2[i] = DE2[i + C] >> K | DE2[i + C + 1] << (7 - K); 00601 if(DS2[i] == 0x00) {LENOUT2 = i; goto salir2;} 00602 K++; 00603 if (K == 7) {K = 0; C++;} // se chequea que ya se acabaron los bits en un ciclo de conversion. 00604 } 00605 00606 salir2: 00607 for (i = 0; i < LENIN2; i++){ 00608 pc.printf("%X", DE2[i]); 00609 } 00610 00611 pc.printf(":\r\n"); 00612 for (i = 0; i < LENOUT2; i++){ 00613 pc.printf("%2X", DS2[i]&0x000000FF); 00614 } 00615 pc.printf("\r\nLENOUT VOLTAJE: %d, LENIN VOLTAJE: %2X\r\n", LENOUT2, LENIN2); 00616 00617 // Concatenación del mensaje en formato PDU y envío del mismo. 00618 ind = 14 + LENOUT2 - 1; 00619 00620 GSM.printf("AT+CMGS=%d\r\n",ind); 00621 pc.printf("AT+CMGS=%d\r\n",ind); 00622 pc.printf(relle1); 00623 GSM.printf(relle1); 00624 00625 for (i=0; i <= 9; i++) { 00626 pc.printf("%c",tel[i]); 00627 GSM.printf("%c",tel[i]); 00628 } 00629 00630 pc.printf(relle2); 00631 GSM.printf(relle2); 00632 pc.printf("%2X", LENIN2); 00633 GSM.printf("%2X", LENIN2); 00634 /*pc.printf("0F"); 00635 GSM.printf("0F");*/ 00636 00637 for (i = 0; i < LENOUT2; i++){ 00638 pc.printf("%02X", DS2[i]&0x000000FF); 00639 GSM.printf("%02X", DS2[i]&0x000000FF); 00640 } 00641 00642 wait(1); 00643 GSM.putc((char)0x1A); // Ctrl - Z 00644 GSM.scanf("%s",buf); // Estado del mensaje (Envió o Error). 00645 pc.printf(">%s\n\n",buf); 00646 pc.printf("\n"); 00647 00648 wait(2); 00649 LedRojo=1; 00650 LedVerde=0; 00651 GSM.printf("AT+CMGD=0\r\n"); // Borra el mensaje actual (Posición "0"). 00652 //pc.printf("\n%s\n\n", "Borro mensaje"); 00653 } 00654 } 00655 } 00656 } 00657 }
Generated on Sat Jul 23 2022 05:14:00 by
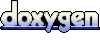