PARA IRDA
Dependents: irda irda1 Tarea5 irda ... more
Fork of Pulse by
Pulse1.h
00001 00002 #ifndef MBED_PULSE1_H 00003 #define MBED_PULSE1_H 00004 00005 #include "mbed.h" 00006 00007 /** Pulse Input/Output Class(es) 00008 */ 00009 00010 class PulseInOut { 00011 public: 00012 /** Create a PulseInOut object connected to the specified pin 00013 * @param pin i/o pin to connect to 00014 */ 00015 PulseInOut(PinName); 00016 ~PulseInOut(); 00017 /** Set the value of the pin 00018 * @param val Value to set, 0 for LOW, otherwise HIGH 00019 */ 00020 void write(int val); 00021 /** Send a pulse of a given value for a specified time 00022 * @param val Value to set, 0 for LOW, otherwise HIGH 00023 * @param time Length of pulse in microseconds 00024 */ 00025 void write_us(int val, int time); 00026 /** Return the length of the next HIGH pulse in microsconds 00027 */ 00028 int read_high_us(); 00029 /** Return the length of the next HIGH pulse in microseconds or -1 if longer than timeout 00030 * @param timeout Time before pulse reading aborts and returns -1, in microseconds 00031 */ 00032 int read_high_us(int timeout); 00033 /** Return the length of the next LOW pulse in microsconds 00034 */ 00035 int read_low_us(); 00036 /** Return the length of the next LOW pulse in microseconds or -1 if longer than timeout 00037 * @param timeout Time before pulse reading aborts and returns -1, in microseconds 00038 */ 00039 int read_low_us(int timeout); 00040 /** Return the length of the next pulse in microsconds 00041 */ 00042 int read_us(); 00043 /** Return the length of the next pulse in microseconds or -1 if longer than timeout 00044 * @param timeout Time before pulse reading aborts and returns -1, in microseconds 00045 */ 00046 int read_us(int timeout); 00047 private: 00048 int startval; 00049 Timer pulsetime, runtime; 00050 DigitalInOut io; 00051 }; 00052 00053 #endif
Generated on Wed Jul 13 2022 00:06:09 by
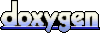