
This is the one where I went back and un-did the cube.cpp file
Dependencies: BNO055_fusion_tom FastPWM mbed
Fork of NucleoCube1 by
cube.h
00001 /* 00002 * cube.h 00003 * April 11, 2017 00004 * 00005 * Control software for balancing cube senior design project 00006 * 00007 * Will Church 00008 * Tom Rasmussen 00009 */ 00010 00011 #ifndef CUBE_H 00012 #define CUBE_H 00013 00014 /* -- INCLUDES -- */ 00015 #include "mbed.h" 00016 #include "BNO055.h" 00017 00018 /* -- STRUCT -- */ 00019 struct config { 00020 double Kbt; // Body angle gain 00021 double Kbv; // Body velocity gain 00022 double Kwv; // Wheel velocity gain 00023 double eqAngle; // Equilibrium angle 00024 double *angle; // Points to angle in IMU Euler_angle struct 00025 double *vel; // Points to vel in IMU VEL struct 00026 PwmOut *pwm; // PWM out for motor control 00027 AnalogIn *hall; // Analog in for wheel velocity 00028 char *descr; // Description of config 00029 }; 00030 00031 /* -- GLOBALS -- */ 00032 Ticker pwmint; // Button interrupt 00033 BNO055_EULER_TypeDef euler_angles; 00034 BNO055_VEL_TypeDef velocity; 00035 config *currentConfig; // Stores current config 00036 bool isActive; // Control loop bool 00037 00038 /* -- CONSTANTS -- */ 00039 const double pi = 3.14159265; 00040 const float TM = .25; //threshold for main axis 00041 const float TA = .2; //threshold for aux axis 00042 00043 /* -- CONFIGS -- */ 00044 /* Define our parameters here for each balancing configuration */ 00045 struct config balX = {-89.9276, //Kbt 00046 -14.9398, //Kbv 00047 -0.001, //Kwv 00048 pi/4.0, //eqAngle 00049 &(euler_angles.r), //angle 00050 &(velocity.x), //vel 00051 new PwmOut(PE_9), //pwm 00052 new AnalogIn(A0), //hall 00053 "Balancing on X edge"}; //descr 00054 00055 struct config balY = {0, //Kbt 00056 0, //Kbv 00057 0, //Kwv 00058 -pi/4.0, //eqAngle 00059 &(euler_angles.p), //angle 00060 &(velocity.y), //vel 00061 new PwmOut(PE_9), //pwm 00062 new AnalogIn(A0), //hall 00063 "Balancing on Y edge"}; //descr 00064 00065 struct config balZ = {-72.4921, //Kbt 00066 -9.9672, //Kbv 00067 -0.00025, //Kwv 00068 -.7000, //eqAngle 00069 &(euler_angles.p), //angle 00070 &(velocity.z), //vel 00071 new PwmOut(PE_9), //pwm 00072 new AnalogIn(A0), //hall 00073 "Balancing on Z edge"}; //descr 00074 00075 struct config fall = {0, //Kbt 00076 0, //Kbv 00077 0, //Kwv 00078 0, //eqAngle 00079 &(euler_angles.p), //angle 00080 &(velocity.z), //vel 00081 NULL, //pwm 00082 NULL, //hall 00083 "Fallen"}; //descr 00084 00085 // Other constants 00086 00087 00088 /* -- NOTES -- */ 00089 //------------------------------------ 00090 // Hyperterminal configuration 00091 // 9600 bauds, 8-bit data, no parity 00092 //------------------------------------ 00093 00094 /* -- PROTOTYPES -- */ 00095 00096 /* 00097 * Checks and prints calibration until sys calib is at 3 / 3 00098 */ 00099 //void checkCalib(BNO055 *imu, Serial *pc); 00100 00101 /* 00102 * TODO: Documentation here 00103 * Note: should this function be inline? 00104 */ 00105 double calcPWM(config *c); 00106 void updatePWM(config *c); 00107 #endif
Generated on Thu Jul 14 2022 01:09:05 by
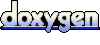