
can't push chnages :(
Fork of FBRDash by
Embed:
(wiki syntax)
Show/hide line numbers
bigchar.cpp
00001 #include "TextLCD.h" 00002 #include "bigchar.h" 00003 00004 //Set up character memory on LCD for drawing two line numbers 00005 void setup_bigchar(TextLCD* lcd) 00006 { 00007 int top_left[8] = {0x07, 0x0F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, }; 00008 lcd->writeCGRAM(TOP_LEFT, top_left); 00009 00010 int top_right[8] = {0x1C, 0x1E, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, }; 00011 lcd->writeCGRAM(TOP_RIGHT, top_right); 00012 00013 int bottom_right[8] = {0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1E, 0x1C, }; 00014 lcd->writeCGRAM(BOTTOM_RIGHT, bottom_right); 00015 00016 int bottom_left[8] = {0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x0F, 0x07, }; 00017 lcd->writeCGRAM(BOTTOM_LEFT, bottom_left); 00018 00019 int two_lines[8] = {0x1F, 0x1F, 0x1F, 0x00, 0x00, 0x00, 0x1F, 0x1F, }; 00020 lcd->writeCGRAM(TWO_LINES, two_lines); 00021 00022 int bottom[8] = {0x00, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x1F, 0x1F, }; 00023 lcd->writeCGRAM(BOTTOM, bottom); 00024 00025 int top[8] = {0x1F, 0x1F, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x00, }; 00026 lcd->writeCGRAM(TOP, top); 00027 00028 int two_lines_5[8] = {0x1F, 0x1F, 0x1F, 0x00, 0x00, 0x00, 0x1E, 0x1F, }; 00029 lcd->writeCGRAM(TWO_LINES_5, two_lines_5); 00030 } 00031 00032 //Draw two-line numbers 00033 void write_bigchar(TextLCD* lcd, int position, int number) 00034 { 00035 switch(number) 00036 { 00037 case 0: 00038 lcd->locate(position, 0); 00039 lcd->putc(TOP_LEFT); 00040 lcd->putc(TOP); 00041 lcd->putc(TOP_RIGHT); 00042 lcd->locate(position, 1); 00043 lcd->putc(BOTTOM_LEFT); 00044 lcd->putc(BOTTOM); 00045 lcd->putc(BOTTOM_RIGHT); 00046 break; 00047 case 1: 00048 lcd->locate(position, 0); 00049 lcd->putc(TOP); 00050 lcd->putc(TOP_RIGHT); 00051 lcd->putc(BLANK); 00052 lcd->locate(position, 1); 00053 lcd->putc(BOTTOM); 00054 lcd->putc(FULL); 00055 lcd->putc(BOTTOM); 00056 break; 00057 case 2: 00058 lcd->locate(position, 0); 00059 lcd->putc(TWO_LINES); 00060 lcd->putc(TWO_LINES); 00061 lcd->putc(TOP_RIGHT); 00062 lcd->locate(position, 1); 00063 lcd->putc(BOTTOM_LEFT); 00064 lcd->putc(BOTTOM); 00065 lcd->putc(BOTTOM); 00066 break; 00067 case 3: 00068 lcd->locate(position, 0); 00069 lcd->putc(TOP); 00070 lcd->putc(TWO_LINES); 00071 lcd->putc(TOP_RIGHT); 00072 lcd->locate(position, 1); 00073 lcd->putc(BOTTOM); 00074 lcd->putc(BOTTOM); 00075 lcd->putc(BOTTOM_RIGHT); 00076 break; 00077 case 4: 00078 lcd->locate(position, 0); 00079 lcd->putc(BOTTOM_LEFT); 00080 lcd->putc(BOTTOM); 00081 lcd->putc(FULL); 00082 lcd->locate(position, 1); 00083 lcd->putc(BLANK); 00084 lcd->putc(BLANK); 00085 lcd->putc(FULL); 00086 break; 00087 case 5: 00088 lcd->locate(position, 0); 00089 lcd->putc(FULL); 00090 lcd->putc(TWO_LINES); 00091 lcd->putc(TWO_LINES_5); 00092 lcd->locate(position, 1); 00093 lcd->putc(BOTTOM); 00094 lcd->putc(BOTTOM); 00095 lcd->putc(BOTTOM_RIGHT); 00096 break; 00097 case 6: 00098 lcd->locate(position, 0); 00099 lcd->putc(TOP_LEFT); 00100 lcd->putc(TWO_LINES); 00101 lcd->putc(TWO_LINES_5); 00102 lcd->locate(position, 1); 00103 lcd->putc(BOTTOM_LEFT); 00104 lcd->putc(BOTTOM); 00105 lcd->putc(BOTTOM_RIGHT); 00106 break; 00107 default: 00108 lcd->locate(position, 0); 00109 lcd->putc(BLANK); 00110 lcd->putc(BLANK); 00111 lcd->putc(BLANK); 00112 lcd->locate(position, 1); 00113 lcd->putc(BLANK); 00114 lcd->putc(BLANK); 00115 lcd->putc(BLANK); 00116 break; 00117 } 00118 }
Generated on Wed Jul 13 2022 14:23:01 by
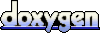