Driver for TI TMP007 thermopile. Reads object temperature, internal temperature, and sensor voltage.
Embed:
(wiki syntax)
Show/hide line numbers
tmp007.cpp
00001 #include "mbed.h" 00002 00003 #define TMP007_Voltage 0x00 00004 #define TMP007_LocalTemp 0x01 00005 #define TMP007_Conf 0x02 00006 #define TMP007_ObjTemp 0x03 00007 00008 #define TMP007_ADDR 0x80 00009 00010 I2C i2c(PA_10, PA_9); 00011 00012 //DigitalOut myled(LED1); 00013 00014 Serial pc(PA_2, PA_3); 00015 00016 int16_t temp_c, temp_c_2s, voltage_tot, voltage_h, voltage_2s, voltage_tot_a; 00017 float celsius, farenheit, voltage; 00018 char temp_write[3]; 00019 char temp_read[2]; 00020 uint8_t temp_h, temp_l, temp_h_and, voltage_l, voltage_h_and; 00021 int sign; 00022 00023 //char TMP007_ObjTemp = 0x03; 00024 00025 00026 int main() 00027 { 00028 00029 00030 00031 /* Configure the Temp Sensor: 00032 00033 */ 00034 temp_write[0] = TMP007_Conf; 00035 temp_write[2] = 0x40; //Mode On, Alert, TC, 16 samples 00036 temp_write[1] = 0x19; //check order of these (endianness) 00037 i2c.write(TMP007_ADDR, temp_write, 3, 0); 00038 00039 while (1) { 00040 // Read Temp Sensor 00041 00042 //Tell the temp sensor to take a one shot temperature measurement 00043 //temp_write[0] = LM75B_Conf; 00044 //temp_write[1] = 0x50; //One shot, low power mode 00045 //i2c.write(LM75B_ADDR, temp_write, 2, 0); 00046 00047 //Read Temperature Register 00048 temp_write[0] = TMP007_ObjTemp; 00049 i2c.write(TMP007_ADDR, temp_write, 1, false); // no stop (unsure) 00050 i2c.read(TMP007_ADDR, temp_read, 2, 0); 00051 00052 temp_h = temp_read[0]; 00053 temp_l = temp_read[1]; 00054 00055 pc.printf("Object Temperature\n"); 00056 pc.printf("high bit = %x\n", temp_h); 00057 pc.printf("low bit = %x\n", temp_l); 00058 00059 temp_c = ((temp_h) << 8) | temp_l; 00060 00061 //int tempval = (int)((int)data_read[0] << 8) | data_read[1]; 00062 pc.printf("combined bit = %x\n", temp_c); 00063 temp_h_and = temp_h & 0x80; 00064 //check for negative number 00065 if (temp_h_and == 0x80) 00066 { 00067 00068 //flip bits and add 1 00069 temp_c_2s = temp_c ^ 0x7FFF + 0x04; 00070 //temp_c_shift = ((temp_c_2s)>>2); 00071 celsius = ((float)temp_c_2s * -0.25f) *.03125f; 00072 pc.printf("negative\n"); 00073 pc.printf("anded high bit = %x\n", temp_h_and); 00074 } 00075 else 00076 { 00077 //temp_c_shift = ((temp_c)>>2); 00078 //pc.printf("shifted bits: %x\n", temp_c_shift); 00079 celsius = ((float)temp_c * 0.25f) *.03125f; 00080 pc.printf("positive\n"); 00081 } 00082 pc.printf("Capsule Temperature is: %f C\n", celsius); 00083 farenheit = 1.8*celsius + 32; 00084 pc.printf("Capsule Temperature is: %f F\n\n", farenheit); 00085 00086 //Read Internal Temperature Register 00087 temp_write[0] = TMP007_LocalTemp; 00088 i2c.write(TMP007_ADDR, temp_write, 1, false); // no stop (unsure) 00089 i2c.read(TMP007_ADDR, temp_read, 2, 0); 00090 00091 temp_h = temp_read[0]; 00092 temp_l = temp_read[1]; 00093 00094 pc.printf("Internal Temperature\n"); 00095 pc.printf("high bit = %x\n", temp_h); 00096 pc.printf("low bit = %x\n", temp_l); 00097 00098 temp_c = ((temp_h) << 8) | temp_l; 00099 00100 //int tempval = (int)((int)data_read[0] << 8) | data_read[1]; 00101 pc.printf("combined bit = %x\n", temp_c); 00102 temp_h_and = temp_h & 0x80; 00103 //check for negative number 00104 if (temp_h_and == 0x80) 00105 { 00106 00107 //flip bits and add 1 00108 temp_c_2s = temp_c ^ 0x7FFF + 0x04; 00109 //temp_c_shift = ((temp_c_2s)>>2); 00110 //scale 00111 celsius = ((float)temp_c_2s * -0.25f) *.03125f; 00112 pc.printf("negative\n"); 00113 //pc.printf("anded high bit = %x\n", temp_h_and); 00114 } 00115 else 00116 { 00117 //temp_c_shift = ((temp_c)>>2); 00118 //pc.printf("shifted bits: %x\n", temp_c_shift); 00119 //scale 00120 celsius = ((float)temp_c * 0.25f) *.03125f; 00121 pc.printf("positive\n"); 00122 } 00123 pc.printf("Internal Temperature is: %f C\n", celsius); 00124 farenheit = 1.8*celsius + 32; 00125 pc.printf("Internal Temperature is: %f F\n\n", farenheit); 00126 00127 //Read Voltage Register 00128 temp_write[0] = TMP007_Voltage; 00129 i2c.write(TMP007_ADDR, temp_write, 1, false); // no stop (unsure) 00130 i2c.read(TMP007_ADDR, temp_read, 2, 0); 00131 00132 voltage_h = temp_read[0]; 00133 voltage_l = temp_read[1]; 00134 00135 pc.printf("Sensor Voltage\n"); 00136 pc.printf("high bit = %x\n", voltage_h); 00137 pc.printf("low bit = %x\n", voltage_l); 00138 00139 voltage_tot = ((voltage_h) << 8) | voltage_l; 00140 voltage_tot_a = voltage_tot & 0x0000FFFF; 00141 00142 //int tempval = (int)((int)data_read[0] << 8) | data_read[1]; 00143 pc.printf("combined bit = %x\n", voltage_tot_a); 00144 voltage_h_and = voltage_h & 0x80; 00145 //check for negative number 00146 if (voltage_h_and == 0x80) 00147 { 00148 00149 //flip bits and add 1 00150 //voltage_2s = voltage_tot ^ 0x7FFF + 0x01; 00151 voltage_2s = ~voltage_tot_a + 0x01; 00152 pc.printf("2's compliment conversion: %x\n", voltage_2s); 00153 //temp_c_shift = ((temp_c_2s)>>2); 00154 voltage = ((float)voltage_2s * -1.0f) *.00015625f; 00155 pc.printf("negative\n"); 00156 //pc.printf("anded high bit = %x\n", temp_h_and); 00157 } 00158 else 00159 { 00160 //temp_c_shift = ((temp_c)>>2); 00161 //pc.printf("shifted bits: %x\n", temp_c_shift); 00162 voltage = ((float)voltage_tot_a * 1.0f) *.00015625f; 00163 pc.printf("positive\n"); 00164 } 00165 pc.printf("Sensor Voltage is: %f mV\n\n\n", voltage); 00166 00167 00168 00169 00170 wait(4); 00171 } 00172 }
Generated on Tue Aug 2 2022 08:21:14 by
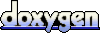