
Test of Embedded Artists LPCXpresso baseboard ethernet, SD card, audio and OLED display facilities. The program displays the day, date and time on the baseboard OLED and sounds the Big Ben chimes on the hour and quarter hour. On initial startup the program checks that the mbed clock is set and that the chime wav files can be accessed on the SD card. If not it asks to be connected to the internet to obtain the current time and to download the wav files to the SD card.
Dependencies: EthernetNetIf NTPClient_NetServices mbed EAOLED
wavplayer.h
00001 /* 00002 Library wave file player by Tom Coxon 00003 00004 Based on WAVEplayer by Vlad Cazan/Stephan Rochon modified by Tom Coxon to: 00005 00006 1. Run correctly on the Embedded Artists LPCXpresso baseboard. 00007 2. To play 8 bit sample size in addition to original 16 bit 00008 3. To be more fault tolerant when playing wav files. 00009 */ 00010 00011 #ifndef WAVPLAYER_H 00012 #define WAVPLAYER_H 00013 00014 #include "mbed.h" 00015 00016 #define SAMPLE_FREQ 40000 00017 #define BUF_SIZE (SAMPLE_FREQ/10) 00018 #define SLICE_BUF_SIZE 1 00019 00020 typedef struct uFMT_STRUCT { 00021 short comp_code; 00022 short num_channels; 00023 unsigned sample_rate; 00024 unsigned avg_Bps; 00025 short block_align; 00026 short sig_bps; 00027 } FMT_STRUCT; 00028 00029 class WavPlayer { 00030 00031 public: 00032 00033 void play_wave(char *wavname); 00034 00035 private: 00036 00037 void cleanup(char *); 00038 void fill_adc_buf(short *, unsigned); 00039 void swapword(unsigned *); 00040 00041 // a FIFO for the DAC 00042 short DAC_fifo[256]; 00043 short DAC_wptr; 00044 short DAC_rptr; 00045 short DAC_on; 00046 00047 void dac_out(); 00048 00049 }; 00050 00051 #endif
Generated on Mon Jul 25 2022 09:57:29 by
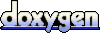