
Simplistic code to test a couple WS2803 in series. WS2803 are 18 channel PWM constant current sources (sinks) normally used to drive 18 LEDs or 6 RGB LEDs.
main.cpp
00001 // WS2803_Testing_MBED 00002 // By Thomas Olson 00003 // teo20130210.01 00004 // MBED LPC1768 00005 // WS2803 pin4 CKI -> ws2803_clockPin(p21) 00006 // WS2803 pin5 SDI -> ws2803_dataPin(p22) 00007 00008 00009 #include "mbed.h" 00010 00011 DigitalOut ws2803_clockPin(p21); 00012 DigitalOut ws2803_dataPin(p22); 00013 00014 #define nLEDs 18 00015 #define nBARs 2 // Number of WS2803 chained together 00016 00017 uint8_t ledBar[nBARs][nLEDs]; 00018 00019 void shiftOut(uint8_t sodata) { 00020 for(int i = 7; i >= 0; i--) { 00021 ws2803_clockPin = 0; 00022 if(sodata & (0x01 << i)){ 00023 ws2803_dataPin = 1; 00024 } else { 00025 ws2803_dataPin = 0; 00026 } 00027 wait_us(1); 00028 ws2803_clockPin = 1; 00029 wait_us(1); 00030 ws2803_clockPin = 0; 00031 } 00032 } 00033 00034 void loadWS2803(){ 00035 for(int wsBar = 0; wsBar < nBARs; wsBar++){ 00036 for (int wsOut = 0; wsOut < nLEDs; wsOut++){ 00037 shiftOut(ledBar[nBARs-(wsBar+1)][wsOut]); 00038 } 00039 } 00040 ws2803_clockPin = 0; 00041 wait_us(600); // 500us-600us needed to reset WS2803s 00042 } 00043 00044 void clearWS2803(){ 00045 for(int wsOut = 0; wsOut < nLEDs; wsOut++){ 00046 ledBar[0][wsOut] = ledBar[1][wsOut] = 0x00; 00047 loadWS2803(); 00048 } 00049 } 00050 00051 int main() { 00052 // initialize WS2803s 00053 ws2803_clockPin = 0; 00054 wait_us(600); 00055 00056 while(1) { 00057 clearWS2803(); 00058 wait(2.0); 00059 // Chase mode 00060 for(int iOut=7; iOut < 256; iOut*=2){ 00061 for(int wsOut = 0; wsOut < nLEDs; wsOut++){ 00062 ledBar[0][wsOut] = ledBar[1][wsOut] = (uint8_t)(0xFF & iOut); 00063 loadWS2803(); 00064 clearWS2803(); 00065 } 00066 } 00067 00068 wait(2.0); 00069 //parallel mode 00070 for(int iOut = 7; iOut < 64; iOut*=4){ 00071 for(int wsOut = 0; wsOut < nLEDs; wsOut++){ 00072 ledBar[0][wsOut] = ledBar[1][wsOut] = (uint8_t)(0xFF & iOut); 00073 loadWS2803(); 00074 wait_us(1000000); 00075 } 00076 wait_us(10000); 00077 } 00078 }// while(1) 00079 }
Generated on Thu Jul 14 2022 10:56:42 by
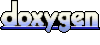