
Download NHK English news podcast automatically. This application requires mpod mother board. See also http://mbed.org/users/geodenx/notebook/mpod/
Dependencies: BlinkLed HTTPClient EthernetInterface FatFileSystemCpp MSCFileSystem mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MSCFileSystem.h" 00003 #include "EthernetInterface.h" 00004 #include "HTTPClient.h" 00005 #include "HTTPFile.h" 00006 #include "BlinkLed.h" 00007 #include "tinyxml2.h" 00008 00009 using namespace tinyxml2; 00010 00011 int GetFile(const char *path, const char *url); 00012 00013 EthernetInterface eth; 00014 HTTPClient http; 00015 MSCFileSystem usb("usb"); 00016 BlinkLed led3(LED3, 0.02); 00017 BlinkLed led4(LED4, 0.2); 00018 BlinkLed ethGreen(p26, 0.02); 00019 BlinkLed ethYellow(p25, 0.2); 00020 DigitalOut fsusb30s(p9); 00021 00022 const char* rssUrl = "http://www3.nhk.or.jp/rj/podcast/rss/english.xml"; 00023 const char* rssPath = "/usb/english.xml"; 00024 const char* mp3Path = "/usb/english.mp3"; 00025 00026 int main() 00027 { 00028 printf("\n\n================================\n"); 00029 printf("mpod NHK English news Downloader\n"); 00030 printf("================================\n\n"); 00031 00032 // Indicate downloading 00033 led4.startBlink(); 00034 ethYellow.startBlink(); 00035 00036 // FSUSB30 switches to HSD1 (mbed) 00037 printf("USB host was switched to HSD1(mbed).\n\n"); 00038 fsusb30s = 0; // HSD1 00039 00040 // Network setup 00041 printf("Setup EtherNet with DHCP.\n"); 00042 eth.init(); //Use DHCP 00043 eth.connect(); 00044 00045 // Obtain original lastBuildDate 00046 char lastBuildDateOriginal[128] = {0}; 00047 { 00048 XMLDocument docOriginal; 00049 if(XML_SUCCESS != docOriginal.LoadFile(rssPath)) 00050 { 00051 strcpy(lastBuildDateOriginal, "No original english.xml in USB memory"); 00052 } 00053 else 00054 { 00055 XMLElement* lastBuildDateOriginalElement = docOriginal.FirstChildElement("rss")->FirstChildElement("channel")->FirstChildElement("lastBuildDate"); 00056 if(NULL == lastBuildDateOriginalElement) 00057 { 00058 strcpy(lastBuildDateOriginal, "No \"lastBuildDate\" element in original RSS"); 00059 } 00060 else 00061 { 00062 strcpy(lastBuildDateOriginal, lastBuildDateOriginalElement->GetText()); 00063 } 00064 } 00065 } 00066 printf("\nlastBuildDate (original): %s\n", lastBuildDateOriginal); 00067 00068 // Download RSS 00069 GetFile(rssPath, rssUrl); 00070 00071 // Obtain current lastBuildDate 00072 char lastBuildDateCurrent[128] = {0}; 00073 char mp3Url[256] = {0}; 00074 char mp3Length[32] = {0}; 00075 { 00076 XMLDocument docCurrent; 00077 if(XML_SUCCESS != docCurrent.LoadFile(rssPath)) 00078 { 00079 fsusb30s = 1; // HSD2 00080 error("No current english.xml in USB memory.\n"); 00081 } 00082 00083 XMLElement* lastBuildDateCurrentElement = docCurrent.FirstChildElement("rss")->FirstChildElement("channel")->FirstChildElement("lastBuildDate"); 00084 if(NULL == lastBuildDateCurrentElement) 00085 { 00086 fsusb30s = 1; // HSD2 00087 error("No \"lastBuildDate\" element in current RSS.\n"); 00088 } 00089 strcpy(lastBuildDateCurrent, lastBuildDateCurrentElement->GetText()); 00090 00091 XMLElement* enclosureElement = docCurrent.FirstChildElement("rss")->FirstChildElement("channel")->FirstChildElement("item")->FirstChildElement("enclosure"); 00092 if(NULL == enclosureElement) 00093 { 00094 fsusb30s = 1; // HSD2 00095 error("No \"enclosure\" element in current RSS.\n"); 00096 } 00097 strcpy(mp3Url, enclosureElement->Attribute("url")); 00098 strcpy(mp3Length, enclosureElement->Attribute("length")); 00099 } 00100 printf("\nlastBuildDate (current) : %s\n", lastBuildDateCurrent); 00101 00102 // Determine the necessity of downloading new MP3. 00103 bool flgDownloadMp3 = false; 00104 if ( strcmp(lastBuildDateOriginal, lastBuildDateCurrent) == 0 ) 00105 { 00106 printf("lastBuildDate (original) == lastBuildDate (current)\n"); 00107 FILE* mp3fp = fopen(mp3Path, "r"); // check an existance of english.mp3 00108 if (mp3fp != NULL) 00109 { 00110 fseek(mp3fp, 0, SEEK_END); // seek to end of file 00111 if (ftell(mp3fp) != atol(mp3Length)) 00112 { 00113 printf("MP3 file size is invalid.\n"); 00114 flgDownloadMp3 = true; 00115 } 00116 fclose(mp3fp); 00117 } 00118 else 00119 { 00120 printf("However, no enlish.mp3 in USB memory\n"); 00121 flgDownloadMp3 = true; 00122 } 00123 } 00124 else 00125 { 00126 printf("lastBuildDate (original) != lastBuildDate (current)\n"); 00127 flgDownloadMp3 = true; 00128 } 00129 00130 // Download new MP3 00131 if(flgDownloadMp3 == true) 00132 { 00133 GetFile(mp3Path, mp3Url); 00134 } 00135 00136 // Wait for the completion of writing to USB Mass Storage Device. 00137 wait(1); 00138 00139 // FSUSB30 switches to HSD2 (External Device) 00140 printf("\nUSB host was switched to HSD2(External Device).\n"); 00141 fsusb30s = 1; // HSD2 00142 00143 // Indicate finish downloading 00144 led4.finishBlink(); 00145 ethYellow.finishBlink(); 00146 led3.startBlink(); 00147 ethGreen.startBlink(); 00148 00149 while(true){} 00150 } 00151 00152 int GetFile(const char *path, const char *url) 00153 { 00154 printf("Getting %s -> %s\n", url, path); 00155 00156 HTTPFile file(path); 00157 HTTPResult retGet = http.get(url, &file); 00158 if (retGet != HTTP_OK) 00159 { 00160 fsusb30s = 1; // HSD2 00161 error("Error in http.get in GetFile(): %d\n", retGet); 00162 } 00163 file.clear(); 00164 00165 return (0); 00166 }
Generated on Wed Jul 13 2022 10:38:55 by
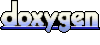