
Download NHK English news podcast automatically. This application requires mpod mother board. See also http://mbed.org/users/geodenx/notebook/mpod/
Dependencies: BlinkLed HTTPClient EthernetInterface FatFileSystemCpp MSCFileSystem mbed-rtos mbed
HTTPFile.h
00001 /* HTTPFile.h */ 00002 #ifndef HTTPFILE_H_ 00003 #define HTTPFILE_H_ 00004 00005 #include "IHTTPData.h" 00006 #include "FATFileSystem.h" 00007 #include <string> 00008 00009 using std::string; 00010 00011 /** A data endpoint to store file 00012 */ 00013 class HTTPFile : public IHTTPDataIn, public IHTTPDataOut 00014 { 00015 public: 00016 /** Create an HTTPFile instance for input 00017 * @param path Path of file to store the incoming string 00018 */ 00019 HTTPFile(const char* path); 00020 00021 ~HTTPFile(); 00022 00023 /** Forces file closure 00024 */ 00025 void clear(); 00026 00027 protected: 00028 /** Reset stream to its beginning 00029 * Called by the HTTPClient on each new request 00030 */ 00031 virtual void readReset(); 00032 00033 /** Read a piece of data to be transmitted 00034 * @param buf Pointer to the buffer on which to copy the data 00035 * @param len Length of the buffer 00036 * @param pReadLen Pointer to the variable on which the actual copied data length will be stored 00037 */ 00038 virtual int read(char* buf, size_t len, size_t* pReadLen); 00039 00040 /** Reset stream to its beginning 00041 * Called by the HTTPClient on each new request 00042 */ 00043 virtual void writeReset(); 00044 00045 /** Write a piece of data transmitted by the server 00046 * @param buf Pointer to the buffer from which to copy the data 00047 * @param len Length of the buffer 00048 */ 00049 virtual int write(const char* buf, size_t len); 00050 00051 /** Get MIME type 00052 * @param type Internet media type from Content-Type header 00053 */ 00054 virtual int getDataType(char* type, size_t maxTypeLen); //Internet media type for Content-Type header 00055 00056 /** Set MIME type 00057 * @param type Internet media type from Content-Type header 00058 */ 00059 virtual void setDataType(const char* type); 00060 00061 /** Determine whether the HTTP client should chunk the data 00062 * Used for Transfer-Encoding header 00063 */ 00064 virtual bool getIsChunked(); 00065 00066 /** Determine whether the data is chunked 00067 * Recovered from Transfer-Encoding header 00068 */ 00069 virtual void setIsChunked(bool chunked); 00070 00071 /** If the data is not chunked, get its size 00072 * Used for Content-Length header 00073 */ 00074 virtual size_t getDataLen(); 00075 00076 /** If the data is not chunked, set its size 00077 * From Content-Length header 00078 */ 00079 virtual void setDataLen(size_t len); 00080 00081 private: 00082 bool openFile(const char* mode); //true on success, false otherwise 00083 void closeFile(); 00084 00085 FILE* m_fp; 00086 string m_path; 00087 size_t m_len; 00088 bool m_chunked; 00089 }; 00090 00091 #endif /* HTTPFILE_H_ */
Generated on Wed Jul 13 2022 10:38:55 by
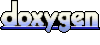