
eDisp library and sample code
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* Sample code for mbed eDISP Library 00002 * Copyright (c) 2010 todotani 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #include "mbed.h" 00007 #include "eDisp.h" 00008 00009 // RGB color code table 00010 int RGB_color[16] = { 00011 RGB_Navy, 00012 RGB_Silver, 00013 RGB_Blue, 00014 RGB_Maroon, 00015 RGB_Purple, 00016 RGB_Red, 00017 RGB_Fuchsia, 00018 RGB_Green, 00019 RGB_Teal, 00020 RGB_Lime, 00021 RGB_Aqua, 00022 RGB_Olive, 00023 RGB_Gray, 00024 RGB_Yellow, 00025 RGB_White, 00026 RGB_Black }; 00027 00028 // RGB color name table 00029 char* colorName[16] = { 00030 "Navy", 00031 "Silver", 00032 "Blue", 00033 "Maroon", 00034 "Purple", 00035 "Red", 00036 "Fuchsia", 00037 "Green", 00038 "Teal", 00039 "Lime", 00040 "Aqua", 00041 "Olive", 00042 "Gray", 00043 "Yellow", 00044 "White", 00045 "Black" }; 00046 00047 00048 eDisp display(p9, p10, 19200); // tx, rx, baud 00049 00050 int main() { 00051 int i; 00052 00053 wait(2); 00054 while (1) { 00055 // Test-1 00056 // clear graphics screen 00057 display.fillRect(0, 320, 240, 0, 0, RGB_Black); 00058 display.reset(); 00059 for (i = 0; i < 15; i++) { 00060 display.fillRect(0, 320, 16, 0, i*16, RGB_color[i]); 00061 display.locate(0, i); 00062 display.printf("%s", colorName[i] ); 00063 } 00064 wait(2); 00065 00066 // Test-2 00067 display.fillRect(0, 320, 240, 0, 0, RGB_Black); 00068 display.cls(); 00069 for (i = 0; i < 15; i++) { 00070 display.drawLine(0, 1, i*16+1, 319, i*16+1, RGB_color[i]); 00071 display.drawLine(0, i*21+1, 0, i*21+1, 239, RGB_color[i]); 00072 } 00073 wait(2); 00074 00075 // Test-3 00076 for (i = 0; i < 15; i++) { 00077 display.fillRect(0, 320, 240, 0, 0, RGB_color[i]); 00078 wait(0.2); // wait until completion of draw 00079 } 00080 wait(2); 00081 00082 // Test-4 00083 display.fillRect(0, 320, 240, 0, 0, RGB_Black); 00084 display.cls(); 00085 for (i = 0; i < 15; i++) { 00086 display.textColor(RED + i%7); 00087 display.printf("漢字表示OK AABBCC\n"); 00088 } 00089 wait(2); 00090 } 00091 }
Generated on Sat Jul 23 2022 13:30:29 by
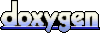