
eDisp library and sample code
Embed:
(wiki syntax)
Show/hide line numbers
eDisp.cpp
00001 /* mbed eDISP Library 00002 * Copyright (c) 2010 todotani 00003 * Version 0.1 (March 6, 2010) 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 #include "eDisp.h" 00008 #include "error.h" 00009 00010 using namespace mbed; 00011 00012 eDisp::eDisp(PinName tx, PinName rx, int baud, int columns, int rows) 00013 :_serial(tx, rx), _columns(columns), _rows(rows) { 00014 _serial.baud(baud); 00015 cls(); 00016 } 00017 00018 int eDisp::_putc(int value) { 00019 if(value == '\n') { 00020 newline(); 00021 } else { 00022 _serial.putc(value); 00023 } 00024 return value; 00025 } 00026 00027 int eDisp::_getc() { 00028 return 0; 00029 } 00030 00031 void eDisp::newline() { 00032 _column = 0; 00033 _row++; 00034 if (_row >= _rows) { 00035 _row = 0; 00036 } 00037 locate(_column, _row); 00038 } 00039 00040 void eDisp::locate(int column, int row) { 00041 if (column < 0 || column >= _columns || row < 0 || row >= _rows) { 00042 error("locate(%d,%d) out of range on %dx%d display", column, row, _columns, _rows); 00043 return; 00044 } 00045 00046 _row = row; 00047 _column = column; 00048 _serial.printf("\x1B[%d;%dH", _row, _column); 00049 } 00050 00051 void eDisp::cls() { 00052 locate(0, 0); 00053 _serial.printf("\x1B[J"); 00054 } 00055 00056 void eDisp::reset() { 00057 _serial.printf("\x1B[m"); 00058 cls(); 00059 } 00060 00061 void eDisp::textColor (int color) { 00062 _serial.printf("\x1B[%dm", color); 00063 } 00064 00065 void eDisp::backgroundColor (int color) { 00066 _serial.printf("\x1B[%dm", color + 10); 00067 } 00068 00069 void eDisp::fillRect (int buffer, int width, int hight, int x, int y, int color ) { 00070 _serial.printf("\x1B@0;%d;%d;%d;%d;%d;%dz", buffer, width, hight, x, y, color); 00071 } 00072 00073 void eDisp::drawLine (int buffer, int x0, int y0, int x1, int y1, int color) { 00074 _serial.printf("\x1B@2;%d;%d;%d;%d;%d;%dz", buffer, x0, y0, x1, y1, color); 00075 }
Generated on Sat Jul 23 2022 13:30:29 by
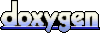