
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
uvc.h
00001 #ifndef UVC_H 00002 #define UVC_H 00003 #include "UsbBaseClass.h" 00004 #include "usb_mem.h" 00005 #include "usb_mjpeg.h" 00006 00007 #define CLASS_VIDEO 0x0E 00008 00009 #define SET_CUR 0x01 00010 #define GET_CUR 0x81 00011 #define GET_MIN 0x82 00012 #define GET_MAX 0x83 00013 #define GET_RES 0x84 00014 #define GET_LEN 0x85 00015 #define GET_INFO 0x86 00016 #define GET_DEF 0x87 00017 00018 #define VS_PROBE_CONTROL 0x01 00019 #define VS_COMMIT_CONTROL 0x02 00020 00021 #define PAYLOAD_UNDEF 0 00022 #define PAYLOAD_MJPEG 1 00023 #define PAYLOAD_YUY2 2 00024 00025 class uvc : public UsbBaseClass { 00026 public: 00027 uvc(int cam = 0); 00028 ~uvc(); 00029 int setup(); 00030 int get_jpeg(const char* path); 00031 int get_jpeg(uint8_t* buf, int size); 00032 bool interrupt(); 00033 int isochronous(); 00034 void attach(usb_stream* stream); 00035 void detach(); 00036 ///set format index 00037 void SetFormatIndex(int index = 1); 00038 ///set frame index 00039 void SetFrameIndex(int index = 1); 00040 ///set frame interval 00041 void SetFrameInterval(int val = 2000000); 00042 ///set packet size 00043 void SetPacketSize(int size = 128); 00044 ///set image size 00045 void SetImageSize(int width = 160, int height = 120); 00046 ///set payload MJPEG or YUY2 00047 void SetPayload(int payload); // MJPEG,YUV422(YUY2) 00048 UsbErr Control(int req, int cs, int index, uint8_t* buf, int size); 00049 ///Setups the result callback 00050 /** 00051 @param pMethod : callback function 00052 */ 00053 void setOnResult( void (*pMethod)(uint16_t, uint8_t*, int) ); 00054 00055 ///Setups the result callback 00056 /** 00057 @param pItem : instance of class on which to execute the callback method 00058 @param pMethod : callback method 00059 */ 00060 class CDummy; 00061 template<class T> 00062 void setOnResult( T* pItem, void (T::*pMethod)(uint16_t, uint8_t*, int) ) 00063 { 00064 m_pCb = NULL; 00065 m_pCbItem = (CDummy*) pItem; 00066 m_pCbMeth = (void (CDummy::*)(uint16_t, uint8_t*, int)) pMethod; 00067 } 00068 void clearOnResult(); 00069 00070 void poll(); 00071 void wait(float s); 00072 void wait_ms(int ms); 00073 uint16_t ReportConditionCode[16]; 00074 protected: 00075 int _init(); 00076 void _config(struct stcamcfg* cfg); 00077 void onResult(uint16_t frame, uint8_t* buf, int len); 00078 bool m_connect; 00079 bool m_init; 00080 int m_cam; 00081 UsbDevice* m_pDev; 00082 UsbEndpoint* m_pEpIntIn; 00083 UsbEndpoint* m_pEpIsoIn; 00084 int m_width; 00085 int m_height; 00086 int m_payload; 00087 int m_FormatIndex; 00088 int m_FrameIndex; 00089 int m_FrameInterval; 00090 int m_PacketSize; 00091 int m_FrameCount; // 1-8 00092 int m_itdCount; 00093 uint8_t m_int_buf[16]; 00094 int m_int_seq; 00095 int m_iso_seq; 00096 uint16_t m_iso_frame; 00097 usb_stream* m_stream; 00098 CDummy* m_pCbItem; 00099 void (CDummy::*m_pCbMeth)(uint16_t, uint8_t*, int); 00100 void (*m_pCb)(uint16_t, uint8_t*, int); 00101 }; 00102 #endif //UVC_H
Generated on Thu Jul 14 2022 15:03:49 by
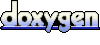