
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
usb_mem.c
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 #include "mbed.h" 00024 //#define __DEBUG 00025 #include "mydbg.h" 00026 #include "usb_mem.h" 00027 #include "string.h" //For memcpy, memmove, memset 00028 //#include "netCfg.h" 00029 #include "UsbInc.h" 00030 00031 //#if NET_USB 00032 00033 #define EDS_COUNT 16 00034 #define TDS_COUNT 0 00035 #define ITDS_COUNT 0 00036 #define UTDS_COUNT 32 00037 #define BPS_COUNT 8 00038 00039 #define HCCA_SIZE 0x100 00040 #define ED_SIZE 0x10 00041 #define TD_SIZE 0x10 00042 #define ITD_SIZE 0x20 00043 #define UTD_SIZE (32+16) 00044 #define BP_SIZE (128*8) 00045 00046 #define TOTAL_SIZE (HCCA_SIZE + (EDS_COUNT*ED_SIZE) + (TDS_COUNT*TD_SIZE) + (ITDS_COUNT*ITD_SIZE)) 00047 00048 static volatile __align(256) byte usb_buf[TOTAL_SIZE] __attribute((section("AHBSRAM0"),aligned)); //256 bytes aligned! 00049 static volatile __align(32) uint8_t usb_utdBuf[UTDS_COUNT*UTD_SIZE] __attribute((section("AHBSRAM0"),aligned)); 00050 00051 static volatile byte* usb_hcca; //256 bytes aligned! 00052 00053 static volatile byte* usb_edBuf; //4 bytes aligned! 00054 00055 static byte usb_edBufAlloc[EDS_COUNT] __attribute((section("AHBSRAM0"),aligned)); 00056 static uint8_t usb_utdBufAlloc[UTDS_COUNT] __attribute((section("AHBSRAM0"),aligned)); 00057 static uint8_t usb_bpBufAlloc[BPS_COUNT] __attribute((section("AHBSRAM0"),aligned)); 00058 static uint8_t usb_bpBuf[BP_SIZE*BPS_COUNT] __attribute((section("AHBSRAM0"),aligned)); 00059 00060 static void utd_init() 00061 { 00062 DBG_ASSERT(sizeof(HCTD) == 16); 00063 DBG_ASSERT(sizeof(HCITD) == 32); 00064 DBG_ASSERT(sizeof(HCUTD) == 48); 00065 00066 DBG_ASSERT(((uint32_t)usb_utdBuf % 16) == 0); 00067 DBG_ASSERT(((uint32_t)usb_utdBuf % 32) == 0); 00068 00069 DBG_ASSERT((uint32_t)usb_utdBufAlloc >= 0x2007c000); 00070 DBG_ASSERT((uint32_t)&usb_utdBufAlloc[UTDS_COUNT] <= 0x2007ffff); 00071 00072 DBG_ASSERT((uint32_t)usb_utdBuf >= 0x2007c000); 00073 DBG_ASSERT((uint32_t)&usb_utdBuf[UTD_SIZE*UTDS_COUNT] <= 0x2007cfff); 00074 00075 memset(usb_utdBufAlloc, 0x00, UTDS_COUNT); 00076 } 00077 00078 static void pb_init() 00079 { 00080 memset(usb_bpBufAlloc, 0x00, BPS_COUNT); 00081 00082 DBG_ASSERT((uint32_t)usb_bpBufAlloc >= 0x2007c000); 00083 DBG_ASSERT((uint32_t)&usb_bpBufAlloc[BPS_COUNT] <= 0x2007ffff); 00084 DBG_ASSERT((uint32_t)usb_bpBuf >= 0x2007c000); 00085 DBG_ASSERT((uint32_t)(&usb_bpBuf[BP_SIZE*BPS_COUNT]) <= 0x2007ffff); 00086 } 00087 00088 void usb_mem_init() 00089 { 00090 usb_hcca = usb_buf; 00091 usb_edBuf = usb_buf + HCCA_SIZE; 00092 memset(usb_edBufAlloc, 0, EDS_COUNT); 00093 00094 utd_init(); 00095 pb_init(); 00096 00097 DBG("--- Memory Map --- \n"); 00098 DBG("usb_hcca =%p\n", usb_hcca); 00099 DBG("usb_edBuf =%p\n", usb_edBuf); 00100 DBG("usb_utdBuf =%p\n", usb_utdBuf); 00101 DBG("usb_edBufAlloc =%p\n", usb_edBufAlloc); 00102 DBG("usb_utdBufAlloc=%p\n", usb_utdBufAlloc); 00103 DBG("usb_bpBufAlloc =%p\n", usb_bpBufAlloc); 00104 DBG("usb_bpBuf =%p\n", usb_bpBuf); 00105 DBG(" =%p\n", &usb_bpBuf[BP_SIZE*BPS_COUNT]); 00106 DBG_ASSERT(((uint32_t)usb_hcca % 256) == 0); 00107 DBG_ASSERT(((uint32_t)usb_edBuf % 16) == 0); 00108 DBG_ASSERT(((uint32_t)usb_utdBuf % 32) == 0); 00109 } 00110 00111 volatile byte* usb_get_hcca() 00112 { 00113 return usb_hcca; 00114 } 00115 00116 volatile byte* usb_get_ed() 00117 { 00118 int i; 00119 for(i = 0; i < EDS_COUNT; i++) 00120 { 00121 if( !usb_edBufAlloc[i] ) 00122 { 00123 usb_edBufAlloc[i] = 1; 00124 return usb_edBuf + i*ED_SIZE; 00125 } 00126 } 00127 return NULL; //Could not alloc ED 00128 } 00129 00130 static uint8_t* usb_get_utd(int al) 00131 { 00132 DBG_ASSERT(al == 16 || al == 32); // GTD or ITD 00133 if (al == 16) { 00134 for(int i = 1; i < UTDS_COUNT; i += 2) { 00135 if (usb_utdBufAlloc[i] == 0) { 00136 uint32_t p = (uint32_t)usb_utdBuf + i * UTD_SIZE; 00137 if ((p % al) == 0) { 00138 usb_utdBufAlloc[i] = 1; 00139 DBG_ASSERT((p % al) == 0); 00140 return (uint8_t*)p; 00141 } 00142 } 00143 } 00144 } 00145 for(int i = 0; i < UTDS_COUNT; i++) { 00146 if (usb_utdBufAlloc[i] == 0) { 00147 uint32_t p = (uint32_t)usb_utdBuf + i * UTD_SIZE; 00148 if ((p % al) == 0) { 00149 usb_utdBufAlloc[i] = 1; 00150 DBG_ASSERT((p % al) == 0); 00151 return (uint8_t*)p; 00152 } 00153 } 00154 } 00155 return NULL; 00156 } 00157 00158 volatile byte* usb_get_td(uint32_t endpoint) 00159 { 00160 DBG_ASSERT(endpoint); 00161 uint8_t* td = usb_get_utd(16); 00162 if (td) { 00163 HCUTD* utd = (HCUTD*)td; 00164 memset(utd, 0x00, sizeof(HCTD)); 00165 utd->type = 1; 00166 utd->UsbEndpoint = endpoint; 00167 } 00168 return td; 00169 } 00170 00171 volatile byte* usb_get_itd(uint32_t endpoint) 00172 { 00173 DBG_ASSERT(endpoint); 00174 uint8_t* itd = usb_get_utd(32); 00175 if (itd) { 00176 HCUTD* utd = (HCUTD*)itd; 00177 memset(utd, 0x00, sizeof(HCITD)); 00178 utd->type = 2; 00179 utd->UsbEndpoint = endpoint; 00180 } 00181 return itd; 00182 } 00183 00184 volatile byte* usb_get_bp(int size) 00185 { 00186 DBG_ASSERT(size >= 128 && size <= BP_SIZE); 00187 if (size > BP_SIZE) { 00188 return NULL; 00189 } 00190 for(int i = 0; i < BPS_COUNT; i++) 00191 { 00192 if( !usb_bpBufAlloc[i] ) 00193 { 00194 usb_bpBufAlloc[i] = 1; 00195 return usb_bpBuf + i*BP_SIZE; 00196 } 00197 } 00198 return NULL; //Could not alloc Buffer Page 00199 } 00200 00201 int usb_bp_size() 00202 { 00203 return BP_SIZE; 00204 } 00205 00206 void usb_free_ed(volatile byte* ed) 00207 { 00208 int i; 00209 i = (ed - usb_edBuf) / ED_SIZE; 00210 usb_edBufAlloc[i] = 0; 00211 } 00212 00213 static void usb_free_utd(volatile uint8_t* utd) 00214 { 00215 DBG_ASSERT(utd >= usb_utdBuf); 00216 DBG_ASSERT(utd <= (usb_utdBuf+UTD_SIZE*(UTDS_COUNT-1))); 00217 DBG_ASSERT(((uint32_t)utd % 16) == 0); 00218 int i = (utd - usb_utdBuf) / UTD_SIZE; 00219 DBG_ASSERT(usb_utdBufAlloc[i] == 1); 00220 usb_utdBufAlloc[i] = 0; 00221 } 00222 00223 void usb_free_td(volatile byte* td) 00224 { 00225 usb_free_utd(td); 00226 return; 00227 } 00228 00229 void usb_free_itd(volatile byte* itd) 00230 { 00231 usb_free_utd(itd); 00232 return; 00233 } 00234 00235 void usb_free_bp(volatile byte* bp) 00236 { 00237 DBG_ASSERT(bp >= usb_bpBuf); 00238 int i; 00239 i = (bp - usb_bpBuf) / BP_SIZE; 00240 DBG_ASSERT(usb_bpBufAlloc[i] == 1); 00241 usb_bpBufAlloc[i] = 0; 00242 } 00243 00244 bool usb_is_td(volatile byte* td) 00245 { 00246 DBG_ASSERT(td); 00247 HCUTD* utd = (HCUTD*)td; 00248 DBG_ASSERT(utd->type != 0); 00249 return utd->type == 1; 00250 } 00251 00252 bool usb_is_itd(volatile byte* itd) 00253 { 00254 DBG_ASSERT(itd); 00255 HCUTD* utd = (HCUTD*)itd; 00256 DBG_ASSERT(utd->type != 0); 00257 return utd->type == 2; 00258 } 00259 00260 //#endif
Generated on Thu Jul 14 2022 15:03:49 by
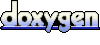