
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
run_loop.h
00001 /* 00002 * Copyright (C) 2009 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 00017 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00018 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00019 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00020 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00021 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00022 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00023 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00024 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00025 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00026 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00027 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00028 * SUCH DAMAGE. 00029 * 00030 */ 00031 00032 /* 00033 * run_loop.h 00034 * 00035 * Created by Matthias Ringwald on 6/6/09. 00036 */ 00037 00038 #pragma once 00039 00040 #include "config.h" 00041 00042 #include "btstack/linked_list.h" 00043 00044 #include <stdint.h> 00045 00046 #ifdef HAVE_TIME 00047 #include <sys/time.h> 00048 #endif 00049 00050 #if defined __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 typedef enum { 00055 RUN_LOOP_POSIX = 1, 00056 RUN_LOOP_COCOA, 00057 RUN_LOOP_EMBEDDED 00058 } RUN_LOOP_TYPE; 00059 00060 typedef struct data_source { 00061 linked_item_t item; 00062 int fd; // <-- file descriptor to watch or 0 00063 int (*process)(struct data_source *ds); // <-- do processing 00064 } data_source_t; 00065 00066 typedef struct timer { 00067 linked_item_t item; 00068 #ifdef HAVE_TIME 00069 struct timeval timeout; // <-- next timeout 00070 #endif 00071 #ifdef HAVE_TICK 00072 uint32_t timeout; // timeout in system ticks 00073 #endif 00074 void (*process)(struct timer *ts); // <-- do processing 00075 } timer_source_t; 00076 00077 00078 // set timer based on current time 00079 void run_loop_set_timer(timer_source_t *a, uint32_t timeout_in_ms); 00080 00081 // add/remove timer_source 00082 void run_loop_add_timer(timer_source_t *timer); 00083 int run_loop_remove_timer(timer_source_t *timer); 00084 00085 // init must be called before any other run_loop call 00086 void run_loop_init(RUN_LOOP_TYPE type); 00087 00088 // add/remove data_source 00089 void run_loop_add_data_source(data_source_t *dataSource); 00090 int run_loop_remove_data_source(data_source_t *dataSource); 00091 00092 00093 // execute configured run_loop 00094 void run_loop_execute(void); 00095 00096 // hack to fix HCI timer handling 00097 #ifdef HAVE_TICK 00098 uint32_t embedded_get_ticks(void); 00099 uint32_t embedded_ticks_for_ms(uint32_t time_in_ms); 00100 #endif 00101 #ifdef EMBEDDED 00102 void embedded_trigger(void); 00103 #endif 00104 #if defined __cplusplus 00105 } 00106 #endif 00107
Generated on Thu Jul 14 2022 15:03:49 by
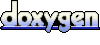