
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
run_loop.c
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * run_loop.c 00039 * 00040 * Created by Matthias Ringwald on 6/6/09. 00041 */ 00042 00043 #include <btstack/run_loop.h> 00044 00045 #include <stdio.h> 00046 #include <stdlib.h> // exit() 00047 00048 #include "run_loop_private.h" 00049 00050 #include "debug.h" 00051 #include "config.h" 00052 00053 static run_loop_t * the_run_loop = NULL; 00054 00055 extern const run_loop_t run_loop_embedded; 00056 00057 #ifdef USE_POSIX_RUN_LOOP 00058 extern run_loop_t run_loop_posix; 00059 #endif 00060 00061 #ifdef USE_COCOA_RUN_LOOP 00062 extern run_loop_t run_loop_cocoa; 00063 #endif 00064 00065 // assert run loop initialized 00066 void run_loop_assert(void){ 00067 #ifndef EMBEDDED 00068 if (!the_run_loop){ 00069 log_error("ERROR: run_loop function called before run_loop_init!\n"); 00070 exit(10); 00071 } 00072 #endif 00073 } 00074 00075 /** 00076 * Add data_source to run_loop 00077 */ 00078 void run_loop_add_data_source(data_source_t *ds){ 00079 run_loop_assert(); 00080 the_run_loop->add_data_source(ds); 00081 } 00082 00083 /** 00084 * Remove data_source from run loop 00085 */ 00086 int run_loop_remove_data_source(data_source_t *ds){ 00087 run_loop_assert(); 00088 return the_run_loop->remove_data_source(ds); 00089 } 00090 00091 /** 00092 * Add timer to run_loop (keep list sorted) 00093 */ 00094 void run_loop_add_timer(timer_source_t *ts){ 00095 run_loop_assert(); 00096 the_run_loop->add_timer(ts); 00097 } 00098 00099 /** 00100 * Remove timer from run loop 00101 */ 00102 int run_loop_remove_timer(timer_source_t *ts){ 00103 run_loop_assert(); 00104 return the_run_loop->remove_timer(ts); 00105 } 00106 00107 void run_loop_timer_dump(){ 00108 run_loop_assert(); 00109 the_run_loop->dump_timer(); 00110 } 00111 00112 /** 00113 * Execute run_loop 00114 */ 00115 void run_loop_execute() { 00116 run_loop_assert(); 00117 the_run_loop->execute(); 00118 } 00119 00120 // init must be called before any other run_loop call 00121 void run_loop_init(RUN_LOOP_TYPE type){ 00122 #ifndef EMBEDDED 00123 if (the_run_loop){ 00124 log_error("ERROR: run loop initialized twice!\n"); 00125 exit(10); 00126 } 00127 #endif 00128 switch (type) { 00129 #ifdef EMBEDDED 00130 case RUN_LOOP_EMBEDDED: 00131 the_run_loop = (run_loop_t*) &run_loop_embedded; 00132 break; 00133 #endif 00134 #ifdef USE_POSIX_RUN_LOOP 00135 case RUN_LOOP_POSIX: 00136 the_run_loop = &run_loop_posix; 00137 break; 00138 #endif 00139 #ifdef USE_COCOA_RUN_LOOP 00140 case RUN_LOOP_COCOA: 00141 the_run_loop = &run_loop_cocoa; 00142 break; 00143 #endif 00144 default: 00145 #ifndef EMBEDDED 00146 log_error("ERROR: invalid run loop type %u selected!\n", type); 00147 exit(10); 00148 #endif 00149 break; 00150 } 00151 the_run_loop->init(); 00152 } 00153
Generated on Thu Jul 14 2022 15:03:49 by
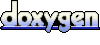