
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
rfcomm.h
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * RFCOMM.h 00039 */ 00040 00041 #include <btstack/btstack.h> 00042 #include <btstack/utils.h> 00043 00044 #include <stdint.h> 00045 00046 #if defined __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 void rfcomm_init(void); 00051 00052 // register packet handler 00053 void rfcomm_packet_handler(uint8_t packet_type, uint16_t channel, uint8_t *packet, uint16_t size); 00054 void rfcomm_register_packet_handler(void (*handler)(void * connection, uint8_t packet_type, 00055 uint16_t channel, uint8_t *packet, uint16_t size)); 00056 00057 // BTstack Internal RFCOMM API 00058 void rfcomm_create_channel_internal(void * connection, bd_addr_t *addr, uint8_t channel); 00059 void rfcomm_create_channel_with_initial_credits_internal(void * connection, bd_addr_t *addr, uint8_t server_channel, uint8_t initial_credits); 00060 void rfcomm_disconnect_internal(uint16_t rfcomm_cid); 00061 void rfcomm_register_service_internal(void * connection, uint8_t channel, uint16_t max_frame_size); 00062 void rfcomm_register_service_with_initial_credits_internal(void * connection, uint8_t channel, uint16_t max_frame_size, uint8_t initial_credits); 00063 void rfcomm_unregister_service_internal(uint8_t service_channel); 00064 void rfcomm_accept_connection_internal(uint16_t rfcomm_cid); 00065 void rfcomm_decline_connection_internal(uint16_t rfcomm_cid); 00066 void rfcomm_grant_credits(uint16_t rfcomm_cid, uint8_t credits); 00067 int rfcomm_send_internal(uint16_t rfcomm_cid, uint8_t *data, uint16_t len); 00068 void rfcomm_close_connection(void *connection); 00069 00070 #define UNLIMITED_INCOMING_CREDITS 0xff 00071 00072 // private structs 00073 typedef enum { 00074 RFCOMM_MULTIPLEXER_CLOSED = 1, 00075 RFCOMM_MULTIPLEXER_W4_CONNECT, // outgoing 00076 RFCOMM_MULTIPLEXER_SEND_SABM_0, // " 00077 RFCOMM_MULTIPLEXER_W4_UA_0, // " 00078 RFCOMM_MULTIPLEXER_W4_SABM_0, // incoming 00079 RFCOMM_MULTIPLEXER_SEND_UA_0, 00080 RFCOMM_MULTIPLEXER_OPEN, 00081 RFCOMM_MULTIPLEXER_SEND_UA_0_AND_DISC 00082 } RFCOMM_MULTIPLEXER_STATE; 00083 00084 typedef enum { 00085 MULT_EV_READY_TO_SEND = 1, 00086 00087 } RFCOMM_MULTIPLEXER_EVENT; 00088 00089 typedef enum { 00090 RFCOMM_CHANNEL_CLOSED = 1, 00091 RFCOMM_CHANNEL_W4_MULTIPLEXER, 00092 RFCOMM_CHANNEL_SEND_UIH_PN, 00093 RFCOMM_CHANNEL_W4_PN_RSP, 00094 RFCOMM_CHANNEL_SEND_SABM_W4_UA, 00095 RFCOMM_CHANNEL_W4_UA, 00096 RFCOMM_CHANNEL_INCOMING_SETUP, 00097 RFCOMM_CHANNEL_DLC_SETUP, 00098 RFCOMM_CHANNEL_OPEN, 00099 RFCOMM_CHANNEL_SEND_UA_AFTER_DISC, 00100 RFCOMM_CHANNEL_SEND_DISC, 00101 RFCOMM_CHANNEL_SEND_DM, 00102 00103 } RFCOMM_CHANNEL_STATE; 00104 00105 typedef enum { 00106 RFCOMM_CHANNEL_STATE_VAR_NONE = 0, 00107 RFCOMM_CHANNEL_STATE_VAR_CLIENT_ACCEPTED = 1 << 0, 00108 RFCOMM_CHANNEL_STATE_VAR_RCVD_PN = 1 << 1, 00109 RFCOMM_CHANNEL_STATE_VAR_RCVD_RPN = 1 << 2, 00110 RFCOMM_CHANNEL_STATE_VAR_RCVD_SABM = 1 << 3, 00111 00112 RFCOMM_CHANNEL_STATE_VAR_RCVD_MSC_CMD = 1 << 4, 00113 RFCOMM_CHANNEL_STATE_VAR_RCVD_MSC_RSP = 1 << 5, 00114 RFCOMM_CHANNEL_STATE_VAR_SEND_PN_RSP = 1 << 6, 00115 RFCOMM_CHANNEL_STATE_VAR_SEND_RPN_INFO = 1 << 7, 00116 00117 RFCOMM_CHANNEL_STATE_VAR_SEND_RPN_RSP = 1 << 8, 00118 RFCOMM_CHANNEL_STATE_VAR_SEND_UA = 1 << 9, 00119 RFCOMM_CHANNEL_STATE_VAR_SEND_MSC_CMD = 1 << 10, 00120 RFCOMM_CHANNEL_STATE_VAR_SEND_MSC_RSP = 1 << 11, 00121 00122 RFCOMM_CHANNEL_STATE_VAR_SEND_CREDITS = 1 << 12, 00123 RFCOMM_CHANNEL_STATE_VAR_SENT_MSC_CMD = 1 << 13, 00124 RFCOMM_CHANNEL_STATE_VAR_SENT_MSC_RSP = 1 << 14, 00125 RFCOMM_CHANNEL_STATE_VAR_SENT_CREDITS = 1 << 15, 00126 } RFCOMM_CHANNEL_STATE_VAR; 00127 00128 typedef enum { 00129 CH_EVT_RCVD_SABM = 1, 00130 CH_EVT_RCVD_UA, 00131 CH_EVT_RCVD_PN, 00132 CH_EVT_RCVD_PN_RSP, 00133 CH_EVT_RCVD_DISC, 00134 CH_EVT_RCVD_DM, 00135 CH_EVT_RCVD_MSC_CMD, 00136 CH_EVT_RCVD_MSC_RSP, 00137 CH_EVT_RCVD_RPN_CMD, 00138 CH_EVT_RCVD_RPN_REQ, 00139 CH_EVT_RCVD_CREDITS, 00140 CH_EVT_MULTIPLEXER_READY, 00141 CH_EVT_READY_TO_SEND, 00142 } RFCOMM_CHANNEL_EVENT; 00143 00144 typedef struct rfcomm_channel_event { 00145 RFCOMM_CHANNEL_EVENT type; 00146 } rfcomm_channel_event_t; 00147 00148 typedef struct rfcomm_channel_event_pn { 00149 rfcomm_channel_event_t super; 00150 uint16_t max_frame_size; 00151 uint8_t priority; 00152 uint8_t credits_outgoing; 00153 } rfcomm_channel_event_pn_t; 00154 00155 typedef struct rfcomm_rpn_data { 00156 uint8_t baud_rate; 00157 uint8_t flags; 00158 uint8_t flow_control; 00159 uint8_t xon; 00160 uint8_t xoff; 00161 uint8_t parameter_mask_0; // first byte 00162 uint8_t parameter_mask_1; // second byte 00163 } rfcomm_rpn_data_t; 00164 00165 typedef struct rfcomm_channel_event_rpn { 00166 rfcomm_channel_event_t super; 00167 rfcomm_rpn_data_t data; 00168 } rfcomm_channel_event_rpn_t; 00169 00170 // info regarding potential connections 00171 typedef struct { 00172 // linked list - assert: first field 00173 linked_item_t item; 00174 00175 // server channel 00176 uint8_t server_channel; 00177 00178 // incoming max frame size 00179 uint16_t max_frame_size; 00180 00181 // use incoming flow control 00182 uint8_t incoming_flow_control; 00183 00184 // initial incoming credits 00185 uint8_t incoming_initial_credits; 00186 00187 // client connection 00188 void *connection; 00189 00190 // internal connection 00191 btstack_packet_handler_t packet_handler; 00192 00193 } rfcomm_service_t; 00194 00195 // info regarding multiplexer 00196 // note: spec mandates single multplexer per device combination 00197 typedef struct { 00198 // linked list - assert: first field 00199 linked_item_t item; 00200 00201 timer_source_t timer; 00202 int timer_active; 00203 00204 RFCOMM_MULTIPLEXER_STATE state; 00205 00206 uint16_t l2cap_cid; 00207 uint8_t l2cap_credits; 00208 00209 bd_addr_t remote_addr; 00210 hci_con_handle_t con_handle; 00211 00212 uint8_t outgoing; 00213 00214 // hack to deal with authentication failure only observed by remote side 00215 uint8_t at_least_one_connection; 00216 00217 uint16_t max_frame_size; 00218 00219 // send DM for DLCI != 0 00220 uint8_t send_dm_for_dlci; 00221 00222 } rfcomm_multiplexer_t; 00223 00224 // info regarding an actual coneection 00225 typedef struct { 00226 // linked list - assert: first field 00227 linked_item_t item; 00228 00229 rfcomm_multiplexer_t *multiplexer; 00230 uint16_t rfcomm_cid; 00231 uint8_t outgoing; 00232 uint8_t dlci; 00233 00234 // number of packets granted to client 00235 uint8_t packets_granted; 00236 00237 // credits for outgoing traffic 00238 uint8_t credits_outgoing; 00239 00240 // number of packets remote will be granted 00241 uint8_t new_credits_incoming; 00242 00243 // credits for incoming traffic 00244 uint8_t credits_incoming; 00245 00246 // use incoming flow control 00247 uint8_t incoming_flow_control; 00248 00249 // channel state 00250 RFCOMM_CHANNEL_STATE state; 00251 00252 // state variables used in RFCOMM_CHANNEL_INCOMING 00253 RFCOMM_CHANNEL_STATE_VAR state_var; 00254 00255 // priority set by incoming side in PN 00256 uint8_t pn_priority; 00257 00258 // negotiated frame size 00259 uint16_t max_frame_size; 00260 00261 // rpn data 00262 rfcomm_rpn_data_t rpn_data; 00263 00264 // server channel (see rfcomm_service_t) - NULL => outgoing channel 00265 rfcomm_service_t * service; 00266 00267 // internal connection 00268 btstack_packet_handler_t packet_handler; 00269 00270 // client connection 00271 void * connection; 00272 00273 } rfcomm_channel_t; 00274 00275 00276 #if defined __cplusplus 00277 } 00278 #endif
Generated on Thu Jul 14 2022 15:03:49 by
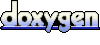