
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
linked_list.c
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * linked_list.c 00039 * 00040 * Created by Matthias Ringwald on 7/13/09. 00041 */ 00042 00043 #include <btstack/linked_list.h> 00044 #include <stdlib.h> 00045 /** 00046 * tests if list is empty 00047 */ 00048 int linked_list_empty(linked_list_t * list){ 00049 return *list == (void *) 0; 00050 } 00051 00052 /** 00053 * linked_list_get_last_item 00054 */ 00055 linked_item_t * linked_list_get_last_item(linked_list_t * list){ // <-- find the last item in the list 00056 linked_item_t *lastItem = NULL; 00057 linked_item_t *it; 00058 for (it = *list; it ; it = it->next){ 00059 if (it) { 00060 lastItem = it; 00061 } 00062 } 00063 return lastItem; 00064 } 00065 00066 00067 /** 00068 * linked_list_add 00069 */ 00070 void linked_list_add(linked_list_t * list, linked_item_t *item){ // <-- add item to list 00071 // check if already in list 00072 linked_item_t *it; 00073 for (it = *list; it ; it = it->next){ 00074 if (it == item) { 00075 return; 00076 } 00077 } 00078 // add first 00079 item->next = *list; 00080 *list = item; 00081 } 00082 00083 void linked_list_add_tail(linked_list_t * list, linked_item_t *item){ // <-- add item to list as last element 00084 // check if already in list 00085 linked_item_t *it; 00086 for (it = (linked_item_t *) list; it->next ; it = it->next){ 00087 if (it->next == item) { 00088 return; 00089 } 00090 } 00091 item->next = (linked_item_t*) 0; 00092 it->next = item; 00093 } 00094 00095 /** 00096 * Remove data_source from run loop 00097 * 00098 * @note: assumes that data_source_t.next is first element in data_source 00099 */ 00100 int linked_list_remove(linked_list_t * list, linked_item_t *item){ // <-- remove item from list 00101 linked_item_t *it; 00102 for (it = (linked_item_t *) list; it ; it = it->next){ 00103 if (it->next == item){ 00104 it->next = item->next; 00105 return 0; 00106 } 00107 } 00108 return -1; 00109 } 00110 00111 void linked_item_set_user(linked_item_t *item, void *user_data){ 00112 item->next = (linked_item_t *) 0; 00113 item->user_data = user_data; 00114 } 00115 00116 void * linked_item_get_user(linked_item_t *item) { 00117 return item->user_data; 00118 } 00119 00120 #if 0 00121 #include <stdio.h> 00122 void test_linked_list(){ 00123 linked_list_t testList = 0; 00124 linked_item_t itemA; 00125 linked_item_t itemB; 00126 linked_item_t itemC; 00127 linked_item_set_user(&itemA, (void *) 0); 00128 linked_item_set_user(&itemB, (void *) 0); 00129 linked_list_add(&testList, &itemA); 00130 linked_list_add(&testList, &itemB); 00131 linked_list_add_tail(&testList, &itemC); 00132 // linked_list_remove(&testList, &itemB); 00133 linked_item_t *it; 00134 for (it = (linked_item_t *) &testList; it ; it = it->next){ 00135 if (it->next == &itemA) printf("Item A\n"); 00136 if (it->next == &itemB) printf("Item B\n"); 00137 if (it->next == &itemC) printf("Item C\n"); 00138 /* if (it->next == &itemB){ 00139 it->next = it->next; 00140 printf(" remove\n"); 00141 } else { 00142 printf(" keep\n"); 00143 00144 */ 00145 } 00146 } 00147 #endif
Generated on Thu Jul 14 2022 15:03:49 by
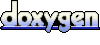