
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
l2cap.h
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * l2cap.h 00039 * 00040 * Logical Link Control and Adaption Protocl (L2CAP) 00041 * 00042 * Created by Matthias Ringwald on 5/16/09. 00043 */ 00044 00045 #pragma once 00046 00047 #include "hci.h" 00048 #include "l2cap_signaling.h" 00049 #include <btstack/utils.h> 00050 #include <btstack/btstack.h> 00051 00052 #if defined __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 #define L2CAP_SIG_ID_INVALID 0 00057 00058 #define L2CAP_HEADER_SIZE 4 00059 00060 // size of HCI ACL + L2CAP Header for regular data packets (8) 00061 #define COMPLETE_L2CAP_HEADER (HCI_ACL_HEADER_SIZE + L2CAP_HEADER_SIZE) 00062 00063 // minimum signaling MTU 00064 #define L2CAP_MINIMAL_MTU 48 00065 #define L2CAP_DEFAULT_MTU 672 00066 00067 // check L2CAP MTU 00068 #if (L2CAP_MINIMAL_MTU + L2CAP_HEADER_SIZE) > HCI_ACL_PAYLOAD_SIZE 00069 #error "HCI_ACL_PAYLOAD_SIZE too small for minimal L2CAP MTU of 48 bytes" 00070 #endif 00071 00072 // L2CAP Fixed Channel IDs 00073 #define L2CAP_CID_SIGNALING 0x0001 00074 #define L2CAP_CID_CONNECTIONLESS_CHANNEL 0x0002 00075 #define L2CAP_CID_ATTRIBUTE_PROTOCOL 0x0004 00076 #define L2CAP_CID_SIGNALING_LE 0x0005 00077 #define L2CAP_CID_SECURITY_MANAGER_PROTOCOL 0x0006 00078 00079 void l2cap_init(void); 00080 void l2cap_register_packet_handler(void (*handler)(void * connection, uint8_t packet_type, uint16_t channel, uint8_t *packet, uint16_t size)); 00081 void l2cap_create_channel_internal(void * connection, btstack_packet_handler_t packet_handler, bd_addr_t address, uint16_t psm, uint16_t mtu); 00082 void l2cap_disconnect_internal(uint16_t local_cid, uint8_t reason); 00083 uint16_t l2cap_get_remote_mtu_for_local_cid(uint16_t local_cid); 00084 uint16_t l2cap_max_mtu(void); 00085 00086 void l2cap_block_new_credits(uint8_t blocked); 00087 int l2cap_can_send_packet_now(uint16_t local_cid); // non-blocking UART write 00088 00089 // get outgoing buffer and prepare data 00090 uint8_t *l2cap_get_outgoing_buffer(void); 00091 00092 int l2cap_send_prepared(uint16_t local_cid, uint16_t len); 00093 int l2cap_send_internal(uint16_t local_cid, uint8_t *data, uint16_t len); 00094 00095 int l2cap_send_prepared_connectionless(uint16_t handle, uint16_t cid, uint16_t len); 00096 int l2cap_send_connectionless(uint16_t handle, uint16_t cid, uint8_t *data, uint16_t len); 00097 00098 void l2cap_close_connection(void *connection); 00099 00100 void l2cap_register_service_internal(void *connection, btstack_packet_handler_t packet_handler, uint16_t psm, uint16_t mtu); 00101 void l2cap_unregister_service_internal(void *connection, uint16_t psm); 00102 00103 void l2cap_accept_connection_internal(uint16_t local_cid); 00104 void l2cap_decline_connection_internal(uint16_t local_cid, uint8_t reason); 00105 00106 // Bluetooth 4.0 - allows to register handler for Attribute Protocol and Security Manager Protocol 00107 void l2cap_register_fixed_channel(btstack_packet_handler_t packet_handler, uint16_t channel_id); 00108 00109 00110 // private structs 00111 typedef enum { 00112 L2CAP_STATE_CLOSED = 1, // no baseband 00113 L2CAP_STATE_WILL_SEND_CREATE_CONNECTION, 00114 L2CAP_STATE_WAIT_CONNECTION_COMPLETE, 00115 L2CAP_STATE_WAIT_CLIENT_ACCEPT_OR_REJECT, 00116 L2CAP_STATE_WAIT_CONNECT_RSP, // from peer 00117 L2CAP_STATE_CONFIG, 00118 L2CAP_STATE_OPEN, 00119 L2CAP_STATE_WAIT_DISCONNECT, // from application 00120 L2CAP_STATE_WILL_SEND_CONNECTION_REQUEST, 00121 L2CAP_STATE_WILL_SEND_CONNECTION_RESPONSE_DECLINE, 00122 L2CAP_STATE_WILL_SEND_CONNECTION_RESPONSE_ACCEPT, 00123 L2CAP_STATE_WILL_SEND_DISCONNECT_REQUEST, 00124 L2CAP_STATE_WILL_SEND_DISCONNECT_RESPONSE, 00125 } L2CAP_STATE; 00126 00127 typedef enum { 00128 L2CAP_CHANNEL_STATE_VAR_NONE = 0, 00129 L2CAP_CHANNEL_STATE_VAR_RCVD_CONF_REQ = 1 << 0, 00130 L2CAP_CHANNEL_STATE_VAR_RCVD_CONF_RSP = 1 << 1, 00131 L2CAP_CHANNEL_STATE_VAR_SEND_CONF_REQ = 1 << 2, 00132 L2CAP_CHANNEL_STATE_VAR_SEND_CONF_RSP = 1 << 3, 00133 L2CAP_CHANNEL_STATE_VAR_SENT_CONF_REQ = 1 << 4, 00134 L2CAP_CHANNEL_STATE_VAR_SENT_CONF_RSP = 1 << 5, 00135 } L2CAP_CHANNEL_STATE_VAR; 00136 00137 // info regarding an actual coneection 00138 typedef struct { 00139 // linked list - assert: first field 00140 linked_item_t item; 00141 00142 L2CAP_STATE state; 00143 L2CAP_CHANNEL_STATE_VAR state_var; 00144 00145 bd_addr_t address; 00146 hci_con_handle_t handle; 00147 00148 uint8_t remote_sig_id; // used by other side, needed for delayed response 00149 uint8_t local_sig_id; // own signaling identifier 00150 00151 uint16_t local_cid; 00152 uint16_t remote_cid; 00153 00154 uint16_t local_mtu; 00155 uint16_t remote_mtu; 00156 00157 uint16_t psm; 00158 00159 uint8_t packets_granted; // number of L2CAP/ACL packets client is allowed to send 00160 00161 uint8_t reason; // used in decline internal 00162 00163 // client connection 00164 void * connection; 00165 00166 // internal connection 00167 btstack_packet_handler_t packet_handler; 00168 00169 } l2cap_channel_t; 00170 00171 // info regarding potential connections 00172 typedef struct { 00173 // linked list - assert: first field 00174 linked_item_t item; 00175 00176 // service id 00177 uint16_t psm; 00178 00179 // incoming MTU 00180 uint16_t mtu; 00181 00182 // client connection 00183 void *connection; 00184 00185 // internal connection 00186 btstack_packet_handler_t packet_handler; 00187 00188 } l2cap_service_t; 00189 00190 00191 typedef struct l2cap_signaling_response { 00192 hci_con_handle_t handle; 00193 uint8_t sig_id; 00194 uint8_t code; 00195 uint16_t data; // infoType for INFORMATION REQUEST, result for CONNECTION request 00196 } l2cap_signaling_response_t; 00197 00198 00199 #if defined __cplusplus 00200 } 00201 #endif
Generated on Thu Jul 14 2022 15:03:48 by
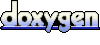