
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
att.h
00001 /* 00002 * Copyright (C) 2011-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 #pragma once 00038 00039 #include <stdint.h> 00040 00041 // MARK: Attribute PDU Opcodes 00042 #define ATT_ERROR_RESPONSE 0x01 00043 00044 #define ATT_EXCHANGE_MTU_REQUEST 0x02 00045 #define ATT_EXCHANGE_MTU_RESPONSE 0x03 00046 00047 #define ATT_FIND_INFORMATION_REQUEST 0x04 00048 #define ATT_FIND_INFORMATION_REPLY 0x05 00049 #define ATT_FIND_BY_TYPE_VALUE_REQUEST 0x06 00050 #define ATT_FIND_BY_TYPE_VALUE_RESPONSE 0x07 00051 00052 #define ATT_READ_BY_TYPE_REQUEST 0x08 00053 #define ATT_READ_BY_TYPE_RESPONSE 0x09 00054 #define ATT_READ_REQUEST 0x0a 00055 #define ATT_READ_RESPONSE 0x0b 00056 #define ATT_READ_BLOB_REQUEST 0x0c 00057 #define ATT_READ_BLOB_RESPONSE 0x0d 00058 #define ATT_READ_MULTIPLE_REQUEST 0x0e 00059 #define ATT_READ_MULTIPLE_RESPONSE 0x0f 00060 #define ATT_READ_BY_GROUP_TYPE_REQUEST 0x10 00061 #define ATT_READ_BY_GROUP_TYPE_RESPONSE 0x11 00062 00063 #define ATT_WRITE_REQUEST 0x12 00064 #define ATT_WRITE_RESPONSE 0x13 00065 00066 #define ATT_PREPARE_WRITE_REQUEST 0x16 00067 #define ATT_PREPARE_WRITE_RESPONSE 0x17 00068 #define ATT_EXECUTE_WRITE_REQUEST 0x18 00069 #define ATT_EXECUTE_WRITE_RESPONSE 0x19 00070 00071 #define ATT_HANDLE_VALUE_NOTIFICATION 0x1b 00072 #define ATT_HANDLE_VALUE_CONFIRMATION 0x1c 00073 #define ATT_HANDLE_VALUE_INDICATION 0x1d 00074 00075 00076 #define ATT_WRITE_COMMAND 0x52 00077 #define ATT_SIGNED_WRITE_COMAND 0xD2 00078 00079 // MARK: ATT Error Codes 00080 #define ATT_ERROR_ATTRIBUTE_INVALID 0x01 00081 #define ATT_ERROR_INVALID_OFFSET 0x07 00082 #define ATT_ERROR_ATTRIBUTE_NOT_FOUND 0x0a 00083 #define ATT_ERROR_UNSUPPORTED_GROUP_TYPE 0x10 00084 00085 // MARK: Attribute Property Flags 00086 #define ATT_PROPERTY_BROADCAST 0x01 00087 #define ATT_PROPERTY_READ 0x02 00088 #define ATT_PROPERTY_WRITE_WITHOUT_RESPONSE 0x04 00089 #define ATT_PROPERTY_WRITE 0x08 00090 #define ATT_PROPERTY_NOTIFY 0x10 00091 #define ATT_PROPERTY_INDICATE 0x20 00092 #define ATT_PROPERTY_AUTHENTICATED_SIGNED_WRITE 0x40 00093 #define ATT_PROPERTY_EXTENDED_PROPERTIES 0x80 00094 00095 // MARK: Attribute Property Flag, BTstack extension 00096 // value is asked from client 00097 #define ATT_PROPERTY_DYNAMIC 0x100 00098 // 128 bit UUID used 00099 #define ATT_PROPERTY_UUID128 0x200 00100 00101 // MARK: GATT UUIDs 00102 #define GATT_PRIMARY_SERVICE_UUID 0x2800 00103 #define GATT_SECONDARY_SERVICE_UUID 0x2801 00104 #define GATT_CHARACTERISTICS_UUID 0x2803 00105 00106 #define GAP_SERVICE_UUID 0x1800 00107 #define GAP_DEVICE_NAME_UUID 0x2a00 00108 00109 #define ATT_TRANSACTION_MODE_NONE 0x0 00110 #define ATT_TRANSACTION_MODE_ACTIVE 0x1 00111 #define ATT_TRANSACTION_MODE_EXECUTE 0x2 00112 #define ATT_TRANSACTION_MODE_CANCEL 0x3 00113 00114 typedef struct att_connection { 00115 uint16_t mtu; 00116 } att_connection_t; 00117 00118 typedef uint8_t signature_t[12]; 00119 00120 // ATT Client Read Callback for Dynamic Data 00121 // - if buffer == NULL, don't copy data, just return size of value 00122 // - if buffer != NULL, copy data and return number bytes copied 00123 // @param offset defines start of attribute value 00124 typedef uint16_t (*att_read_callback_t)(uint16_t handle, uint16_t offset, uint8_t * buffer, uint16_t buffer_size); 00125 00126 // ATT Client Write Callback for Dynamic Data 00127 // @param handle to be written 00128 // @param transaction - ATT_TRANSACTION_MODE_NONE for regular writes, ATT_TRANSACTION_MODE_ACTIVE for prepared writes and ATT_TRANSACTION_MODE_EXECUTE 00129 // @param offset into the value - used for queued writes and long attributes 00130 // @param buffer 00131 // @param buffer_size 00132 // @Param signature used for signed write commmands 00133 typedef void (*att_write_callback_t)(uint16_t handle, uint16_t transaction_mode, uint16_t offset, uint8_t *buffer, uint16_t buffer_size, signature_t * signature); 00134 00135 // MARK: ATT Operations 00136 00137 void att_set_db(uint8_t const * db); 00138 00139 void att_set_read_callback(att_read_callback_t callback); 00140 00141 void att_set_write_callback(att_write_callback_t callback); 00142 00143 void att_dump_attributes(void); 00144 00145 // response buffer size = att_connection->mtu 00146 uint16_t att_handle_request(att_connection_t * att_connection, 00147 uint8_t * request_buffer, 00148 uint16_t request_len, 00149 uint8_t * response_buffer); 00150 00151 uint16_t att_prepare_handle_value_notification(att_connection_t * att_connection, 00152 uint16_t handle, 00153 uint8_t *value, 00154 uint16_t value_len, 00155 uint8_t * response_buffer); 00156 00157 uint16_t att_prepare_handle_value_indication(att_connection_t * att_connection, 00158 uint16_t handle, 00159 uint8_t *value, 00160 uint16_t value_len, 00161 uint8_t * response_buffer); 00162 00163 00164
Generated on Thu Jul 14 2022 15:03:48 by
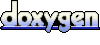