
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
UsbHostMgr2.cpp
00001 #include "UsbHostMgr.h" 00002 //#define __DEBUG 00003 #include "mydbg.h" 00004 00005 UsbDevice* UsbHostMgr::getDeviceByClass(uint8_t IfClass, int count) 00006 { 00007 DBG("IfClass=%02X count=%d\n", IfClass, count); 00008 for(int i = 0; i < USB_HOSTMGR_MAX_DEVS; i++) { 00009 UsbDevice* dev = m_lpDevices[i]; 00010 if (dev) { 00011 if(dev->m_connected && dev->m_enumerated) { 00012 if (dev->m_InterfaceClass == IfClass) { // found 00013 if (count-- <= 0) { 00014 return dev; 00015 } 00016 } 00017 } 00018 } 00019 } 00020 return NULL; 00021 } 00022 00023 void UsbHostMgr::onUsbDeviceConnected(int hub, int port) 00024 { 00025 DBG("%p hub=%d port=%d\n", this, hub, port); 00026 for(int i = 0; i < USB_HOSTMGR_MAX_DEVS; i++) { 00027 UsbDevice* dev = m_lpDevices[i]; 00028 if (dev) { 00029 if (dev->m_hub == hub && dev->m_port == port) { // skip 00030 return; 00031 } 00032 } 00033 } 00034 00035 int item = devicesCount(); 00036 DBG_ASSERT(item < USB_HOSTMGR_MAX_DEVS); 00037 if( item == USB_HOSTMGR_MAX_DEVS ) 00038 return; //List full... 00039 //Find a free address (not optimized, but not really important) 00040 int addr = 1; 00041 for(int i = 0; i < item; i++) 00042 { 00043 DBG_ASSERT(m_lpDevices[i]); 00044 addr = MAX( addr, m_lpDevices[i]->m_addr + 1 ); 00045 } 00046 DBG("item=%d addr=%d\n", item, addr); 00047 UsbDevice* dev = new UsbDevice( this, hub, port, addr ); 00048 DBG_ASSERT(dev); 00049 dev->m_connected = true; 00050 m_lpDevices[item] = dev; 00051 }
Generated on Thu Jul 14 2022 15:03:49 by
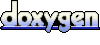