
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
UsbEndpoint2.cpp
00001 /* 00002 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 #include "UsbEndpoint.h" 00023 #include "UsbDevice.h" 00024 #include "usb_mem.h" 00025 00026 //#define __DEBUG 00027 //#define __DEBUG3 00028 //#include "dbg/dbg.h" 00029 #include "mydbg.h" 00030 00031 void UsbEndpoint::UsbEndpoint_iso(UsbDevice* pDevice, uint8_t ep, bool dir, UsbEndpointType type, uint16_t size, int addr) 00032 { 00033 m_itdActive = 0; 00034 m_pEd = (volatile HCED*)usb_get_ed(); 00035 DBG_ASSERT(m_pEd); 00036 memset((void*)m_pEd, 0, sizeof(HCED)); 00037 00038 m_pTdHead = NULL; 00039 m_pTdTail = NULL; 00040 00041 volatile HCTD* itd = (volatile HCTD*)usb_get_itd((uint32_t)this); 00042 DBG_ASSERT(itd); 00043 memset((void*)itd, 0, sizeof(HCITD)); 00044 DBG3("m_pEd =%p\n", m_pEd); 00045 DBG3("itd =%p\n", itd); 00046 00047 if(addr == -1) 00048 addr = pDevice->m_addr; 00049 00050 //Setup Ed 00051 //printf("\r\n--Ep Setup--\r\n"); 00052 m_pEd->Control = addr | /* USB address */ 00053 ((ep & 0x7F) << 7) | /* Endpoint address */ 00054 ((dir?2:1) << 11) | /* direction : Out = 1, 2 = In */ 00055 (1 << 15) | /* F Format */ 00056 (size << 16); /* MaxPkt Size */ 00057 00058 DBG3("m_pEd->Control=%08X\n", m_pEd->Control); 00059 00060 m_dir = dir; 00061 m_setup = false; 00062 m_type = type; 00063 00064 m_pEd->TailTd = m_pEd->HeadTd = (uint32_t)itd; //Empty TD list 00065 00066 DBG("Before link\n"); 00067 00068 //printf("\r\n--Ep Reg--\r\n"); 00069 //Append Ed to Ed list 00070 HCCA* hcca = (HCCA*)usb_get_hcca(); 00071 for(int i = 0; i < 32; i++) { 00072 if (hcca->IntTable[i] == 0) { 00073 hcca->IntTable[i] = (uint32_t)m_pEd; 00074 } else { 00075 volatile HCED* nextEd = (volatile HCED*)hcca->IntTable[i]; 00076 while(nextEd->Next && nextEd->Next != (uint32_t)m_pEd) { 00077 nextEd = (volatile HCED*)nextEd->Next; 00078 } 00079 nextEd->Next = (uint32_t)m_pEd; 00080 } 00081 } 00082 } 00083 00084 // for isochronous 00085 UsbErr UsbEndpoint::transfer(uint16_t frame, int count, volatile uint8_t* buf, int len) 00086 { 00087 DBG_ASSERT(count >= 1 && count <= 8); 00088 DBG_ASSERT(buf); 00089 DBG_ASSERT((len % count) == 0); 00090 HCITD *itd = (HCITD*)m_pEd->TailTd; 00091 DBG_ASSERT(itd); 00092 HCITD *new_itd = (HCITD*)usb_get_itd((uint32_t)this); 00093 DBG_ASSERT(new_itd); 00094 if (itd == NULL) { 00095 return USBERR_ERROR; 00096 } 00097 DBG("itd=%p\n", itd); 00098 DBG2("new_itd=%p\n", new_itd); 00099 int di = 0; //DelayInterrupt 00100 itd->Control = 0xe0000000 | // CC ConditionCode NOT ACCESSED 00101 ((count-1) << 24) | // FC FrameCount 00102 TD_DELAY_INT(di) | // DI DelayInterrupt 00103 frame; // SF StartingFrame 00104 itd->BufferPage0 = (uint32_t)buf; 00105 itd->BufferEnd = (uint32_t)buf+len-1; 00106 itd->Next = (uint32_t)new_itd; 00107 uint16_t offset[8]; 00108 for(int i = 0; i < 8; i++) { 00109 uint32_t addr = (uint32_t)buf + i*(len/count); 00110 offset[i] = addr & 0x0fff; 00111 if ((addr&0xfffff000) == (itd->BufferEnd&0xfffff000)) { 00112 offset[i] |= 0x1000; 00113 } 00114 offset[i] |= 0xe000; 00115 } 00116 itd->OffsetPSW10 = (offset[1]<<16) | offset[0]; 00117 itd->OffsetPSW32 = (offset[3]<<16) | offset[2]; 00118 itd->OffsetPSW54 = (offset[5]<<16) | offset[4]; 00119 itd->OffsetPSW76 = (offset[7]<<16) | offset[6]; 00120 m_itdActive++; 00121 DBG2("itd->Control =%08X\n", itd->Control); 00122 DBG2("itd->BufferPage0=%08X\n", itd->BufferPage0); 00123 DBG2("itd->Next =%08X\n", itd->Next); 00124 DBG2("itd->BufferEnd =%08X\n", itd->BufferEnd); 00125 DBG2("itd->OffsetPSW10=%08X\n", itd->OffsetPSW10); 00126 DBG2("itd->OffsetPSW32=%08X\n", itd->OffsetPSW32); 00127 DBG2("itd->OffsetPSW54=%08X\n", itd->OffsetPSW54); 00128 DBG2("itd->OffsetPSW76=%08X\n", itd->OffsetPSW76); 00129 m_pEd->TailTd = (uint32_t)new_itd; // start!!! 00130 LPC_USB->HcControl |= OR_CONTROL_PLE; //Enable Periodic 00131 return USBERR_PROCESSING; 00132 }
Generated on Thu Jul 14 2022 15:03:49 by
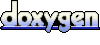