
BLE demo for mbed Ported RunningElectronics's SBDBT firmware for BLE. It can communicate with iOS
Dependencies: FatFileSystem mbed
Fork of BTstack by
UsbDevice2.cpp
00001 #include "UsbDevice.h" 00002 //#define __DEBUG 00003 #include "mydbg.h" 00004 00005 #define PORT_RESET 4 00006 #define PORT_POWER 8 00007 #define C_PORT_CONNECTION 16 00008 #define C_PORT_RESET 20 00009 00010 UsbErr UsbDevice::hub_init() 00011 { 00012 UsbErr rc; 00013 uint8_t buf[9]; 00014 rc = controlReceive( 00015 USB_DEVICE_TO_HOST | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_DEVICE, // 0xa0 00016 GET_DESCRIPTOR, 00017 (USB_DESCRIPTOR_TYPE_HUB << 8), 0, buf, sizeof(buf)); 00018 DBG_ASSERT(rc == USBERR_OK); 00019 DBG_ASSERT(buf[0] == 9); 00020 DBG_ASSERT(buf[1] == 0x29); 00021 DBG_BYTES("HUB DESCRIPTOR", buf, sizeof(buf)); 00022 00023 m_hub_ports = buf[2]; 00024 VERBOSE("NbrPorts: %d\n", m_hub_ports); 00025 int PwrOn2PwrGood = buf[5]; 00026 VERBOSE("PwrOn2PwrGood: %d %d ms\n", PwrOn2PwrGood, PwrOn2PwrGood*2); 00027 VERBOSE("HubContrCurrent: %d\n", buf[6]); 00028 00029 rc = setConfiguration(1); 00030 DBG_ASSERT(rc == USBERR_OK); 00031 00032 uint8_t status[4]; 00033 rc = controlReceive( 00034 USB_DEVICE_TO_HOST | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_DEVICE, // 0xa0 00035 GET_STATUS, 00036 0, 0, status, sizeof(status)); 00037 DBG_ASSERT(rc == USBERR_OK); 00038 DBG_BYTES("HUB STATUS", status, sizeof(status)); 00039 00040 for(int i = 1; i <= m_hub_ports; i++) { 00041 rc = SetPortFeature(PORT_POWER, i); 00042 DBG("PORT_POWER port=%d rc=%d\n", i, rc); 00043 DBG_ASSERT(rc == USBERR_OK); 00044 if (rc != USBERR_OK) { 00045 return rc; 00046 } 00047 } 00048 wait_ms(PwrOn2PwrGood*2); 00049 00050 m_enumerated = true; 00051 return USBERR_OK; 00052 } 00053 00054 UsbErr UsbDevice::hub_poll() 00055 { 00056 DBG("%p m_hub=%d m_port=%d m_addr=%d\n", this, m_hub, m_port, m_addr); 00057 // check status 00058 for(int port = 1; port <= m_hub_ports; port++) { 00059 uint8_t status[4]; 00060 UsbErr rc = GetPortStatus(port, status, sizeof(status)); 00061 DBG_ASSERT(rc == USBERR_OK); 00062 DBG("port=%d\n", port); 00063 DBG_BYTES("STATUS", status, sizeof(status)); 00064 if (status[2] & 0x01) { // Connect Status Change, has changed 00065 DBG_ASSERT(status[0] & 0x01); 00066 ClearPortFeature(C_PORT_CONNECTION, port); 00067 DBG_ASSERT(m_pMgr); 00068 m_pMgr->onUsbDeviceConnected(m_addr, port); 00069 return USBERR_PROCESSING; 00070 } 00071 } 00072 return USBERR_OK; 00073 } 00074 00075 UsbErr UsbDevice::hub_PortReset(int port) 00076 { 00077 DBG("%p port=%d\n", this, port); 00078 DBG_ASSERT(port >= 1); 00079 SetPortReset(port); 00080 // wait reset 00081 for(int i = 0; i < 100; i++) { 00082 uint8_t status[4]; 00083 UsbErr rc = GetPortStatus(port, status, sizeof(status)); 00084 DBG_ASSERT(rc == USBERR_OK); 00085 DBG_BYTES("RESET", status, sizeof(status)); 00086 if (status[2] & 0x10) { // Reset change , Reset complete 00087 return USBERR_OK; 00088 } 00089 wait_ms(5); 00090 } 00091 return USBERR_ERROR; 00092 } 00093 00094 UsbErr UsbDevice::SetPortFeature(int feature, int index) 00095 { 00096 //DBG("feature=%d index=%d\n", feature, index); 00097 UsbErr rc; 00098 rc = controlSend( 00099 USB_HOST_TO_DEVICE | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_OTHER, 00100 SET_FEATURE, feature, index, 0, 0); 00101 return rc; 00102 } 00103 00104 UsbErr UsbDevice::ClearPortFeature(int feature, int index) 00105 { 00106 UsbErr rc; 00107 rc = controlSend( 00108 USB_HOST_TO_DEVICE | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_OTHER, 00109 CLEAR_FEATURE, feature, index, 0, 0); 00110 return rc; 00111 } 00112 00113 UsbErr UsbDevice::SetPortReset(int port) 00114 { 00115 //DBG("port=%d\n", port); 00116 UsbErr rc = SetPortFeature(PORT_RESET, port); 00117 DBG_ASSERT(rc == USBERR_OK); 00118 return rc; 00119 } 00120 00121 UsbErr UsbDevice::GetPortStatus(int port, uint8_t* buf, int size) 00122 { 00123 DBG_ASSERT(size == 4); 00124 UsbErr rc; 00125 //return USBControlTransfer(device, 00126 //DEVICE_TO_HOST | REQUEST_TYPE_CLASS | RECIPIENT_OTHER, 00127 //GET_STATUS,0,port,(u8*)status,4); 00128 00129 rc = controlReceive( 00130 USB_DEVICE_TO_HOST | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_OTHER, 00131 GET_STATUS, 0, port, buf, sizeof(buf)); 00132 return rc; 00133 }
Generated on Thu Jul 14 2022 15:03:49 by
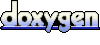