http://ndevilla.free.fr/iniparser/ Welcome to iniParser -- version 3.1 released 08 Apr 2012 This modules offers parsing of ini files from the C level. See a complete documentation in HTML format, from this directory open the file html/index.html with any HTML-capable browser. Enjoy! N.Devillard Sun Apr 8 16:38:09 CEST 2012
iniparser.h
00001 00002 /*-------------------------------------------------------------------------*/ 00003 /** 00004 @file iniparser.h 00005 @author N. Devillard 00006 @brief Parser for ini files. 00007 */ 00008 /*--------------------------------------------------------------------------*/ 00009 00010 #ifndef _INIPARSER_H_ 00011 #define _INIPARSER_H_ 00012 00013 /*--------------------------------------------------------------------------- 00014 Includes 00015 ---------------------------------------------------------------------------*/ 00016 00017 #include <stdio.h> 00018 #include <stdlib.h> 00019 #include <string.h> 00020 00021 /* 00022 * The following #include is necessary on many Unixes but not Linux. 00023 * It is not needed for Windows platforms. 00024 * Uncomment it if needed. 00025 */ 00026 /* #include <unistd.h> */ 00027 00028 #include "dictionary.h" 00029 00030 /*-------------------------------------------------------------------------*/ 00031 /** 00032 @brief Get number of sections in a dictionary 00033 @param d Dictionary to examine 00034 @return int Number of sections found in dictionary 00035 00036 This function returns the number of sections found in a dictionary. 00037 The test to recognize sections is done on the string stored in the 00038 dictionary: a section name is given as "section" whereas a key is 00039 stored as "section:key", thus the test looks for entries that do not 00040 contain a colon. 00041 00042 This clearly fails in the case a section name contains a colon, but 00043 this should simply be avoided. 00044 00045 This function returns -1 in case of error. 00046 */ 00047 /*--------------------------------------------------------------------------*/ 00048 00049 int iniparser_getnsec(dictionary * d); 00050 00051 00052 /*-------------------------------------------------------------------------*/ 00053 /** 00054 @brief Get name for section n in a dictionary. 00055 @param d Dictionary to examine 00056 @param n Section number (from 0 to nsec-1). 00057 @return Pointer to char string 00058 00059 This function locates the n-th section in a dictionary and returns 00060 its name as a pointer to a string statically allocated inside the 00061 dictionary. Do not free or modify the returned string! 00062 00063 This function returns NULL in case of error. 00064 */ 00065 /*--------------------------------------------------------------------------*/ 00066 00067 char * iniparser_getsecname(dictionary * d, int n); 00068 00069 00070 /*-------------------------------------------------------------------------*/ 00071 /** 00072 @brief Save a dictionary to a loadable ini file 00073 @param d Dictionary to dump 00074 @param f Opened file pointer to dump to 00075 @return void 00076 00077 This function dumps a given dictionary into a loadable ini file. 00078 It is Ok to specify @c stderr or @c stdout as output files. 00079 */ 00080 /*--------------------------------------------------------------------------*/ 00081 00082 void iniparser_dump_ini(dictionary * d, FILE * f); 00083 00084 /*-------------------------------------------------------------------------*/ 00085 /** 00086 @brief Save a dictionary section to a loadable ini file 00087 @param d Dictionary to dump 00088 @param s Section name of dictionary to dump 00089 @param f Opened file pointer to dump to 00090 @return void 00091 00092 This function dumps a given section of a given dictionary into a loadable ini 00093 file. It is Ok to specify @c stderr or @c stdout as output files. 00094 */ 00095 /*--------------------------------------------------------------------------*/ 00096 00097 void iniparser_dumpsection_ini(dictionary * d, char * s, FILE * f); 00098 00099 /*-------------------------------------------------------------------------*/ 00100 /** 00101 @brief Dump a dictionary to an opened file pointer. 00102 @param d Dictionary to dump. 00103 @param f Opened file pointer to dump to. 00104 @return void 00105 00106 This function prints out the contents of a dictionary, one element by 00107 line, onto the provided file pointer. It is OK to specify @c stderr 00108 or @c stdout as output files. This function is meant for debugging 00109 purposes mostly. 00110 */ 00111 /*--------------------------------------------------------------------------*/ 00112 void iniparser_dump(dictionary * d, FILE * f); 00113 00114 /*-------------------------------------------------------------------------*/ 00115 /** 00116 @brief Get the number of keys in a section of a dictionary. 00117 @param d Dictionary to examine 00118 @param s Section name of dictionary to examine 00119 @return Number of keys in section 00120 */ 00121 /*--------------------------------------------------------------------------*/ 00122 int iniparser_getsecnkeys(dictionary * d, char * s); 00123 00124 /*-------------------------------------------------------------------------*/ 00125 /** 00126 @brief Get the number of keys in a section of a dictionary. 00127 @param d Dictionary to examine 00128 @param s Section name of dictionary to examine 00129 @return pointer to statically allocated character strings 00130 00131 This function queries a dictionary and finds all keys in a given section. 00132 Each pointer in the returned char pointer-to-pointer is pointing to 00133 a string allocated in the dictionary; do not free or modify them. 00134 00135 This function returns NULL in case of error. 00136 */ 00137 /*--------------------------------------------------------------------------*/ 00138 char ** iniparser_getseckeys(dictionary * d, char * s); 00139 00140 /*-------------------------------------------------------------------------*/ 00141 /** 00142 @brief Get the string associated to a key 00143 @param d Dictionary to search 00144 @param key Key string to look for 00145 @param def Default value to return if key not found. 00146 @return pointer to statically allocated character string 00147 00148 This function queries a dictionary for a key. A key as read from an 00149 ini file is given as "section:key". If the key cannot be found, 00150 the pointer passed as 'def' is returned. 00151 The returned char pointer is pointing to a string allocated in 00152 the dictionary, do not free or modify it. 00153 */ 00154 /*--------------------------------------------------------------------------*/ 00155 char * iniparser_getstring(dictionary * d, const char * key, char * def); 00156 00157 /*-------------------------------------------------------------------------*/ 00158 /** 00159 @brief Get the string associated to a key, convert to an int 00160 @param d Dictionary to search 00161 @param key Key string to look for 00162 @param notfound Value to return in case of error 00163 @return integer 00164 00165 This function queries a dictionary for a key. A key as read from an 00166 ini file is given as "section:key". If the key cannot be found, 00167 the notfound value is returned. 00168 00169 Supported values for integers include the usual C notation 00170 so decimal, octal (starting with 0) and hexadecimal (starting with 0x) 00171 are supported. Examples: 00172 00173 - "42" -> 42 00174 - "042" -> 34 (octal -> decimal) 00175 - "0x42" -> 66 (hexa -> decimal) 00176 00177 Warning: the conversion may overflow in various ways. Conversion is 00178 totally outsourced to strtol(), see the associated man page for overflow 00179 handling. 00180 00181 Credits: Thanks to A. Becker for suggesting strtol() 00182 */ 00183 /*--------------------------------------------------------------------------*/ 00184 int iniparser_getint(dictionary * d, const char * key, int notfound); 00185 00186 /*-------------------------------------------------------------------------*/ 00187 /** 00188 @brief Get the string associated to a key, convert to a double 00189 @param d Dictionary to search 00190 @param key Key string to look for 00191 @param notfound Value to return in case of error 00192 @return double 00193 00194 This function queries a dictionary for a key. A key as read from an 00195 ini file is given as "section:key". If the key cannot be found, 00196 the notfound value is returned. 00197 */ 00198 /*--------------------------------------------------------------------------*/ 00199 double iniparser_getdouble(dictionary * d, const char * key, double notfound); 00200 00201 /*-------------------------------------------------------------------------*/ 00202 /** 00203 @brief Get the string associated to a key, convert to a boolean 00204 @param d Dictionary to search 00205 @param key Key string to look for 00206 @param notfound Value to return in case of error 00207 @return integer 00208 00209 This function queries a dictionary for a key. A key as read from an 00210 ini file is given as "section:key". If the key cannot be found, 00211 the notfound value is returned. 00212 00213 A true boolean is found if one of the following is matched: 00214 00215 - A string starting with 'y' 00216 - A string starting with 'Y' 00217 - A string starting with 't' 00218 - A string starting with 'T' 00219 - A string starting with '1' 00220 00221 A false boolean is found if one of the following is matched: 00222 00223 - A string starting with 'n' 00224 - A string starting with 'N' 00225 - A string starting with 'f' 00226 - A string starting with 'F' 00227 - A string starting with '0' 00228 00229 The notfound value returned if no boolean is identified, does not 00230 necessarily have to be 0 or 1. 00231 */ 00232 /*--------------------------------------------------------------------------*/ 00233 int iniparser_getboolean(dictionary * d, const char * key, int notfound); 00234 00235 00236 /*-------------------------------------------------------------------------*/ 00237 /** 00238 @brief Set an entry in a dictionary. 00239 @param ini Dictionary to modify. 00240 @param entry Entry to modify (entry name) 00241 @param val New value to associate to the entry. 00242 @return int 0 if Ok, -1 otherwise. 00243 00244 If the given entry can be found in the dictionary, it is modified to 00245 contain the provided value. If it cannot be found, -1 is returned. 00246 It is Ok to set val to NULL. 00247 */ 00248 /*--------------------------------------------------------------------------*/ 00249 int iniparser_set(dictionary * ini, const char * entry, const char * val); 00250 00251 00252 /*-------------------------------------------------------------------------*/ 00253 /** 00254 @brief Delete an entry in a dictionary 00255 @param ini Dictionary to modify 00256 @param entry Entry to delete (entry name) 00257 @return void 00258 00259 If the given entry can be found, it is deleted from the dictionary. 00260 */ 00261 /*--------------------------------------------------------------------------*/ 00262 void iniparser_unset(dictionary * ini, const char * entry); 00263 00264 /*-------------------------------------------------------------------------*/ 00265 /** 00266 @brief Finds out if a given entry exists in a dictionary 00267 @param ini Dictionary to search 00268 @param entry Name of the entry to look for 00269 @return integer 1 if entry exists, 0 otherwise 00270 00271 Finds out if a given entry exists in the dictionary. Since sections 00272 are stored as keys with NULL associated values, this is the only way 00273 of querying for the presence of sections in a dictionary. 00274 */ 00275 /*--------------------------------------------------------------------------*/ 00276 int iniparser_find_entry(dictionary * ini, const char * entry) ; 00277 00278 /*-------------------------------------------------------------------------*/ 00279 /** 00280 @brief Parse an ini file and return an allocated dictionary object 00281 @param ininame Name of the ini file to read. 00282 @return Pointer to newly allocated dictionary 00283 00284 This is the parser for ini files. This function is called, providing 00285 the name of the file to be read. It returns a dictionary object that 00286 should not be accessed directly, but through accessor functions 00287 instead. 00288 00289 The returned dictionary must be freed using iniparser_freedict(). 00290 */ 00291 /*--------------------------------------------------------------------------*/ 00292 dictionary * iniparser_load(const char * ininame); 00293 00294 /*-------------------------------------------------------------------------*/ 00295 /** 00296 @brief Free all memory associated to an ini dictionary 00297 @param d Dictionary to free 00298 @return void 00299 00300 Free all memory associated to an ini dictionary. 00301 It is mandatory to call this function before the dictionary object 00302 gets out of the current context. 00303 */ 00304 /*--------------------------------------------------------------------------*/ 00305 void iniparser_freedict(dictionary * d); 00306 00307 #endif
Generated on Tue Jul 12 2022 19:10:58 by
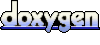