http://ndevilla.free.fr/iniparser/ Welcome to iniParser -- version 3.1 released 08 Apr 2012 This modules offers parsing of ini files from the C level. See a complete documentation in HTML format, from this directory open the file html/index.html with any HTML-capable browser. Enjoy! N.Devillard Sun Apr 8 16:38:09 CEST 2012
dictionary.h
00001 00002 /*-------------------------------------------------------------------------*/ 00003 /** 00004 @file dictionary.h 00005 @author N. Devillard 00006 @brief Implements a dictionary for string variables. 00007 00008 This module implements a simple dictionary object, i.e. a list 00009 of string/string associations. This object is useful to store e.g. 00010 informations retrieved from a configuration file (ini files). 00011 */ 00012 /*--------------------------------------------------------------------------*/ 00013 00014 #ifndef _DICTIONARY_H_ 00015 #define _DICTIONARY_H_ 00016 00017 /*--------------------------------------------------------------------------- 00018 Includes 00019 ---------------------------------------------------------------------------*/ 00020 00021 #include <stdio.h> 00022 #include <stdlib.h> 00023 #include <string.h> 00024 //#include <unistd.h> // Not required for MBED 00025 00026 /*--------------------------------------------------------------------------- 00027 New types 00028 ---------------------------------------------------------------------------*/ 00029 00030 00031 /*-------------------------------------------------------------------------*/ 00032 /** 00033 @brief Dictionary object 00034 00035 This object contains a list of string/string associations. Each 00036 association is identified by a unique string key. Looking up values 00037 in the dictionary is speeded up by the use of a (hopefully collision-free) 00038 hash function. 00039 */ 00040 /*-------------------------------------------------------------------------*/ 00041 typedef struct _dictionary_ { 00042 int n ; /** Number of entries in dictionary */ 00043 int size ; /** Storage size */ 00044 char ** val ; /** List of string values */ 00045 char ** key ; /** List of string keys */ 00046 unsigned * hash ; /** List of hash values for keys */ 00047 } dictionary ; 00048 00049 00050 /*--------------------------------------------------------------------------- 00051 Function prototypes 00052 ---------------------------------------------------------------------------*/ 00053 00054 /*-------------------------------------------------------------------------*/ 00055 /** 00056 @brief Compute the hash key for a string. 00057 @param key Character string to use for key. 00058 @return 1 unsigned int on at least 32 bits. 00059 00060 This hash function has been taken from an Article in Dr Dobbs Journal. 00061 This is normally a collision-free function, distributing keys evenly. 00062 The key is stored anyway in the struct so that collision can be avoided 00063 by comparing the key itself in last resort. 00064 */ 00065 /*--------------------------------------------------------------------------*/ 00066 unsigned dictionary_hash(const char * key); 00067 00068 /*-------------------------------------------------------------------------*/ 00069 /** 00070 @brief Create a new dictionary object. 00071 @param size Optional initial size of the dictionary. 00072 @return 1 newly allocated dictionary objet. 00073 00074 This function allocates a new dictionary object of given size and returns 00075 it. If you do not know in advance (roughly) the number of entries in the 00076 dictionary, give size=0. 00077 */ 00078 /*--------------------------------------------------------------------------*/ 00079 dictionary * dictionary_new(int size); 00080 00081 /*-------------------------------------------------------------------------*/ 00082 /** 00083 @brief Delete a dictionary object 00084 @param d dictionary object to deallocate. 00085 @return void 00086 00087 Deallocate a dictionary object and all memory associated to it. 00088 */ 00089 /*--------------------------------------------------------------------------*/ 00090 void dictionary_del(dictionary * vd); 00091 00092 /*-------------------------------------------------------------------------*/ 00093 /** 00094 @brief Get a value from a dictionary. 00095 @param d dictionary object to search. 00096 @param key Key to look for in the dictionary. 00097 @param def Default value to return if key not found. 00098 @return 1 pointer to internally allocated character string. 00099 00100 This function locates a key in a dictionary and returns a pointer to its 00101 value, or the passed 'def' pointer if no such key can be found in 00102 dictionary. The returned character pointer points to data internal to the 00103 dictionary object, you should not try to free it or modify it. 00104 */ 00105 /*--------------------------------------------------------------------------*/ 00106 char * dictionary_get(dictionary * d, const char * key, char * def); 00107 00108 00109 /*-------------------------------------------------------------------------*/ 00110 /** 00111 @brief Set a value in a dictionary. 00112 @param d dictionary object to modify. 00113 @param key Key to modify or add. 00114 @param val Value to add. 00115 @return int 0 if Ok, anything else otherwise 00116 00117 If the given key is found in the dictionary, the associated value is 00118 replaced by the provided one. If the key cannot be found in the 00119 dictionary, it is added to it. 00120 00121 It is Ok to provide a NULL value for val, but NULL values for the dictionary 00122 or the key are considered as errors: the function will return immediately 00123 in such a case. 00124 00125 Notice that if you dictionary_set a variable to NULL, a call to 00126 dictionary_get will return a NULL value: the variable will be found, and 00127 its value (NULL) is returned. In other words, setting the variable 00128 content to NULL is equivalent to deleting the variable from the 00129 dictionary. It is not possible (in this implementation) to have a key in 00130 the dictionary without value. 00131 00132 This function returns non-zero in case of failure. 00133 */ 00134 /*--------------------------------------------------------------------------*/ 00135 int dictionary_set(dictionary * vd, const char * key, const char * val); 00136 00137 /*-------------------------------------------------------------------------*/ 00138 /** 00139 @brief Delete a key in a dictionary 00140 @param d dictionary object to modify. 00141 @param key Key to remove. 00142 @return void 00143 00144 This function deletes a key in a dictionary. Nothing is done if the 00145 key cannot be found. 00146 */ 00147 /*--------------------------------------------------------------------------*/ 00148 void dictionary_unset(dictionary * d, const char * key); 00149 00150 00151 /*-------------------------------------------------------------------------*/ 00152 /** 00153 @brief Dump a dictionary to an opened file pointer. 00154 @param d Dictionary to dump 00155 @param f Opened file pointer. 00156 @return void 00157 00158 Dumps a dictionary onto an opened file pointer. Key pairs are printed out 00159 as @c [Key]=[Value], one per line. It is Ok to provide stdout or stderr as 00160 output file pointers. 00161 */ 00162 /*--------------------------------------------------------------------------*/ 00163 void dictionary_dump(dictionary * d, FILE * out); 00164 00165 #endif
Generated on Tue Jul 12 2022 19:10:58 by
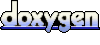