
inital draft
Dependencies: mbed LoRaWAN-lib SX1272Liby
Fork of LoRaWAN-demo-72_tjm by
cmac.cpp
00001 /************************************************************************** 00002 Copyright (C) 2009 Lander Casado, Philippas Tsigas 00003 00004 All rights reserved. 00005 00006 Permission is hereby granted, free of charge, to any person obtaining 00007 a copy of this software and associated documentation files 00008 (the "Software"), to deal with the Software without restriction, including 00009 without limitation the rights to use, copy, modify, merge, publish, 00010 distribute, sublicense, and/or sell copies of the Software, and to 00011 permit persons to whom the Software is furnished to do so, subject to 00012 the following conditions: 00013 00014 Redistributions of source code must retain the above copyright notice, 00015 this list of conditions and the following disclaimers. Redistributions in 00016 binary form must reproduce the above copyright notice, this list of 00017 conditions and the following disclaimers in the documentation and/or 00018 other materials provided with the distribution. 00019 00020 In no event shall the authors or copyright holders be liable for any special, 00021 incidental, indirect or consequential damages of any kind, or any damages 00022 whatsoever resulting from loss of use, data or profits, whether or not 00023 advised of the possibility of damage, and on any theory of liability, 00024 arising out of or in connection with the use or performance of this software. 00025 00026 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00027 OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00028 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00029 CONTRIBUTORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00030 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00031 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00032 DEALINGS WITH THE SOFTWARE 00033 00034 *****************************************************************************/ 00035 //#include <sys/param.h> 00036 //#include <sys/systm.h> 00037 #include <stdint.h> 00038 #include "aes.h" 00039 #include "cmac.h" 00040 #include "utilities.h" 00041 00042 #define LSHIFT(v, r) do { \ 00043 int32_t i; \ 00044 for (i = 0; i < 15; i++) \ 00045 (r)[i] = (v)[i] << 1 | (v)[i + 1] >> 7; \ 00046 (r)[15] = (v)[15] << 1; \ 00047 } while (0) 00048 00049 #define XOR(v, r) do { \ 00050 int32_t i; \ 00051 for (i = 0; i < 16; i++) \ 00052 { \ 00053 (r)[i] = (r)[i] ^ (v)[i]; \ 00054 } \ 00055 } while (0) \ 00056 00057 00058 void AES_CMAC_Init(AES_CMAC_CTX *ctx) 00059 { 00060 memset1(ctx->X, 0, sizeof ctx->X); 00061 ctx->M_n = 0; 00062 memset1(ctx->rijndael.ksch, '\0', 240); 00063 } 00064 00065 void AES_CMAC_SetKey(AES_CMAC_CTX *ctx, const uint8_t key[AES_CMAC_KEY_LENGTH]) 00066 { 00067 //rijndael_set_key_enc_only(&ctx->rijndael, key, 128); 00068 aes_set_key( key, AES_CMAC_KEY_LENGTH, &ctx->rijndael); 00069 } 00070 00071 void AES_CMAC_Update(AES_CMAC_CTX *ctx, const uint8_t *data, uint32_t len) 00072 { 00073 uint32_t mlen; 00074 uint8_t in[16]; 00075 00076 if (ctx->M_n > 0) { 00077 mlen = MIN(16 - ctx->M_n, len); 00078 memcpy1(ctx->M_last + ctx->M_n, data, mlen); 00079 ctx->M_n += mlen; 00080 if (ctx->M_n < 16 || len == mlen) 00081 return; 00082 XOR(ctx->M_last, ctx->X); 00083 //rijndael_encrypt(&ctx->rijndael, ctx->X, ctx->X); 00084 aes_encrypt( ctx->X, ctx->X, &ctx->rijndael); 00085 data += mlen; 00086 len -= mlen; 00087 } 00088 while (len > 16) { /* not last block */ 00089 00090 XOR(data, ctx->X); 00091 //rijndael_encrypt(&ctx->rijndael, ctx->X, ctx->X); 00092 00093 memcpy1(in, &ctx->X[0], 16); //Bestela ez du ondo iten 00094 aes_encrypt( in, in, &ctx->rijndael); 00095 memcpy1(&ctx->X[0], in, 16); 00096 00097 data += 16; 00098 len -= 16; 00099 } 00100 /* potential last block, save it */ 00101 memcpy1(ctx->M_last, data, len); 00102 ctx->M_n = len; 00103 } 00104 00105 void AES_CMAC_Final(uint8_t digest[AES_CMAC_DIGEST_LENGTH], AES_CMAC_CTX *ctx) 00106 { 00107 uint8_t K[16]; 00108 uint8_t in[16]; 00109 /* generate subkey K1 */ 00110 memset1(K, '\0', 16); 00111 00112 //rijndael_encrypt(&ctx->rijndael, K, K); 00113 00114 aes_encrypt( K, K, &ctx->rijndael); 00115 00116 if (K[0] & 0x80) { 00117 LSHIFT(K, K); 00118 K[15] ^= 0x87; 00119 } else 00120 LSHIFT(K, K); 00121 00122 00123 if (ctx->M_n == 16) { 00124 /* last block was a complete block */ 00125 XOR(K, ctx->M_last); 00126 00127 } else { 00128 /* generate subkey K2 */ 00129 if (K[0] & 0x80) { 00130 LSHIFT(K, K); 00131 K[15] ^= 0x87; 00132 } else 00133 LSHIFT(K, K); 00134 00135 /* padding(M_last) */ 00136 ctx->M_last[ctx->M_n] = 0x80; 00137 while (++ctx->M_n < 16) 00138 ctx->M_last[ctx->M_n] = 0; 00139 00140 XOR(K, ctx->M_last); 00141 00142 00143 } 00144 XOR(ctx->M_last, ctx->X); 00145 00146 //rijndael_encrypt(&ctx->rijndael, ctx->X, digest); 00147 00148 memcpy1(in, &ctx->X[0], 16); //Bestela ez du ondo iten 00149 aes_encrypt(in, digest, &ctx->rijndael); 00150 memset1(K, 0, sizeof K); 00151 00152 } 00153
Generated on Wed Jul 13 2022 20:47:11 by
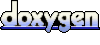