
inital draft
Dependencies: mbed LoRaWAN-lib SX1272Liby
Fork of LoRaWAN-demo-72_tjm by
SerialDisplay.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2015 Semtech 00008 00009 Description: VT100 serial display management 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #include "board.h" 00016 #include "vt100.h" 00017 #include "SerialDisplay.h" 00018 00019 VT100 vt( USBTX, USBRX ); 00020 00021 void SerialPrintCheckBox( bool activated, uint8_t color ) 00022 { 00023 if( activated == true ) 00024 { 00025 vt.SetAttribute( VT100::ATTR_OFF, color, color ); 00026 } 00027 else 00028 { 00029 vt.SetAttribute( VT100::ATTR_OFF ); 00030 } 00031 vt.printf( " " ); 00032 vt.SetAttribute( VT100::ATTR_OFF ); 00033 } 00034 00035 void SerialDisplayUpdateActivationMode( bool otaa ) 00036 { 00037 vt.SetCursorPos( 4, 17 ); 00038 SerialPrintCheckBox( otaa, VT100::WHITE ); 00039 vt.SetCursorPos( 9, 17 ); 00040 SerialPrintCheckBox( !otaa, VT100::WHITE ); 00041 } 00042 00043 void SerialDisplayUpdateEui( uint8_t line, uint8_t *eui ) 00044 { 00045 vt.SetCursorPos( line, 27 ); 00046 for( uint8_t i = 0; i < 8; i++ ) 00047 { 00048 vt.printf( "%02X ", eui[i] ); 00049 } 00050 vt.SetCursorPos( line, 50 ); 00051 vt.printf( "]" ); 00052 } 00053 00054 void SerialDisplayUpdateKey( uint8_t line, uint8_t *key ) 00055 { 00056 vt.SetCursorPos( line, 27 ); 00057 for( uint8_t i = 0; i < 16; i++ ) 00058 { 00059 vt.printf( "%02X ", key[i] ); 00060 } 00061 vt.SetCursorPos( line, 74 ); 00062 vt.printf( "]" ); 00063 } 00064 00065 void SerialDisplayUpdateNwkId( uint8_t id ) 00066 { 00067 vt.SetCursorPos( 10, 27 ); 00068 vt.printf( "%03d", id ); 00069 } 00070 00071 void SerialDisplayUpdateDevAddr( uint32_t addr ) 00072 { 00073 vt.SetCursorPos( 11, 27 ); 00074 vt.printf( "%02X %02X %02X %02X", ( addr >> 24 ) & 0xFF, ( addr >> 16 ) & 0xFF, ( addr >> 8 ) & 0xFF, addr & 0xFF ); 00075 } 00076 00077 void SerialDisplayUpdateFrameType( bool confirmed ) 00078 { 00079 vt.SetCursorPos( 15, 17 ); 00080 SerialPrintCheckBox( confirmed, VT100::WHITE ); 00081 vt.SetCursorPos( 15, 32 ); 00082 SerialPrintCheckBox( !confirmed, VT100::WHITE ); 00083 } 00084 00085 void SerialDisplayUpdateAdr( bool adr ) 00086 { 00087 vt.SetCursorPos( 16, 27 ); 00088 if( adr == true ) 00089 { 00090 vt.printf( " ON" ); 00091 } 00092 else 00093 { 00094 vt.printf( "OFF" ); 00095 } 00096 } 00097 00098 void SerialDisplayUpdateDutyCycle( bool dutyCycle ) 00099 { 00100 vt.SetCursorPos( 17, 27 ); 00101 if( dutyCycle == true ) 00102 { 00103 vt.printf( " ON" ); 00104 } 00105 else 00106 { 00107 vt.printf( "OFF" ); 00108 } 00109 } 00110 00111 void SerialDisplayUpdatePublicNetwork( bool network ) 00112 { 00113 vt.SetCursorPos( 19, 17 ); 00114 SerialPrintCheckBox( network, VT100::WHITE ); 00115 vt.SetCursorPos( 19, 30 ); 00116 SerialPrintCheckBox( !network, VT100::WHITE ); 00117 } 00118 00119 void SerialDisplayUpdateNetworkIsJoined( bool state ) 00120 { 00121 vt.SetCursorPos( 20, 17 ); 00122 SerialPrintCheckBox( !state, VT100::RED ); 00123 vt.SetCursorPos( 20, 30 ); 00124 SerialPrintCheckBox( state, VT100::GREEN ); 00125 } 00126 00127 void SerialDisplayUpdateLedState( uint8_t id, uint8_t state ) 00128 { 00129 switch( id ) 00130 { 00131 case 1: 00132 vt.SetCursorPos( 22, 17 ); 00133 SerialPrintCheckBox( state, VT100::RED ); 00134 break; 00135 case 2: 00136 vt.SetCursorPos( 22, 31 ); 00137 SerialPrintCheckBox( state, VT100::GREEN ); 00138 break; 00139 case 3: 00140 vt.SetCursorPos( 22, 45 ); 00141 SerialPrintCheckBox( state, VT100::BLUE ); 00142 break; 00143 } 00144 } 00145 00146 void SerialDisplayUpdateData( uint8_t line, uint8_t *buffer, uint8_t size ) 00147 { 00148 if( size != 0 ) 00149 { 00150 vt.SetCursorPos( line, 27 ); 00151 for( uint8_t i = 0; i < size; i++ ) 00152 { 00153 vt.printf( "%02X ", buffer[i] ); 00154 if( ( ( i + 1 ) % 16 ) == 0 ) 00155 { 00156 line++; 00157 vt.SetCursorPos( line, 27 ); 00158 } 00159 } 00160 for( uint8_t i = size; i < 64; i++ ) 00161 { 00162 vt.printf( "__ " ); 00163 if( ( ( i + 1 ) % 16 ) == 0 ) 00164 { 00165 line++; 00166 vt.SetCursorPos( line, 27 ); 00167 } 00168 } 00169 vt.SetCursorPos( line - 1, 74 ); 00170 vt.printf( "]" ); 00171 } 00172 else 00173 { 00174 vt.SetCursorPos( line, 27 ); 00175 for( uint8_t i = 0; i < 64; i++ ) 00176 { 00177 vt.printf( "__ " ); 00178 if( ( ( i + 1 ) % 16 ) == 0 ) 00179 { 00180 line++; 00181 vt.SetCursorPos( line, 27 ); 00182 } 00183 } 00184 vt.SetCursorPos( line - 1, 74 ); 00185 vt.printf( "]" ); 00186 } 00187 } 00188 00189 void SerialDisplayUpdateUplinkAcked( bool state ) 00190 { 00191 vt.SetCursorPos( 24, 36 ); 00192 SerialPrintCheckBox( state, VT100::GREEN ); 00193 } 00194 00195 void SerialDisplayUpdateUplink( bool acked, uint8_t datarate, uint16_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize ) 00196 { 00197 // Acked 00198 SerialDisplayUpdateUplinkAcked( acked ); 00199 // Datarate 00200 vt.SetCursorPos( 25, 33 ); 00201 vt.printf( "DR%d", datarate ); 00202 // Counter 00203 vt.SetCursorPos( 26, 27 ); 00204 vt.printf( "%10d", counter ); 00205 // Port 00206 vt.SetCursorPos( 27, 34 ); 00207 vt.printf( "%3d", port ); 00208 // Data 00209 SerialDisplayUpdateData( 28, buffer, bufferSize ); 00210 // Help message 00211 vt.SetCursorPos( 42, 1 ); 00212 vt.printf( "To refresh screen please hit 'r' key." ); 00213 } 00214 00215 void SerialDisplayUpdateDonwlinkRxData( bool state ) 00216 { 00217 vt.SetCursorPos( 34, 4 ); 00218 SerialPrintCheckBox( state, VT100::GREEN ); 00219 } 00220 00221 void SerialDisplayUpdateDownlink( bool rxData, int16_t rssi, int8_t snr, uint16_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize ) 00222 { 00223 // Rx data 00224 SerialDisplayUpdateDonwlinkRxData( rxData ); 00225 // RSSI 00226 vt.SetCursorPos( 33, 32 ); 00227 vt.printf( "%5d", rssi ); 00228 // SNR 00229 vt.SetCursorPos( 34, 32 ); 00230 vt.printf( "%5d", snr ); 00231 // Counter 00232 vt.SetCursorPos( 35, 27 ); 00233 vt.printf( "%10d", counter ); 00234 if( rxData == true ) 00235 { 00236 // Port 00237 vt.SetCursorPos( 36, 34 ); 00238 vt.printf( "%3d", port ); 00239 // Data 00240 SerialDisplayUpdateData( 37, buffer, bufferSize ); 00241 } 00242 else 00243 { 00244 // Port 00245 vt.SetCursorPos( 36, 34 ); 00246 vt.printf( " " ); 00247 // Data 00248 SerialDisplayUpdateData( 37, NULL, 0 ); 00249 } 00250 } 00251 00252 void SerialDisplayDrawFirstLine( void ) 00253 { 00254 vt.PutBoxDrawingChar( 'l' ); 00255 for( int8_t i = 0; i <= 77; i++ ) 00256 { 00257 vt.PutBoxDrawingChar( 'q' ); 00258 } 00259 vt.PutBoxDrawingChar( 'k' ); 00260 vt.printf( "\r\n" ); 00261 } 00262 00263 void SerialDisplayDrawTitle( const char* title ) 00264 { 00265 vt.PutBoxDrawingChar( 'x' ); 00266 vt.printf( "%s", title ); 00267 vt.PutBoxDrawingChar( 'x' ); 00268 vt.printf( "\r\n" ); 00269 } 00270 void SerialDisplayDrawTopSeparator( void ) 00271 { 00272 vt.PutBoxDrawingChar( 't' ); 00273 for( int8_t i = 0; i <= 11; i++ ) 00274 { 00275 vt.PutBoxDrawingChar( 'q' ); 00276 } 00277 vt.PutBoxDrawingChar( 'w' ); 00278 for( int8_t i = 0; i <= 64; i++ ) 00279 { 00280 vt.PutBoxDrawingChar( 'q' ); 00281 } 00282 vt.PutBoxDrawingChar( 'u' ); 00283 vt.printf( "\r\n" ); 00284 } 00285 00286 void SerialDisplayDrawColSeparator( void ) 00287 { 00288 vt.PutBoxDrawingChar( 'x' ); 00289 for( int8_t i = 0; i <= 11; i++ ) 00290 { 00291 vt.PutBoxDrawingChar( ' ' ); 00292 } 00293 vt.PutBoxDrawingChar( 't' ); 00294 for( int8_t i = 0; i <= 64; i++ ) 00295 { 00296 vt.PutBoxDrawingChar( 'q' ); 00297 } 00298 vt.PutBoxDrawingChar( 'u' ); 00299 vt.printf( "\r\n" ); 00300 } 00301 00302 void SerialDisplayDrawSeparator( void ) 00303 { 00304 vt.PutBoxDrawingChar( 't' ); 00305 for( int8_t i = 0; i <= 11; i++ ) 00306 { 00307 vt.PutBoxDrawingChar( 'q' ); 00308 } 00309 vt.PutBoxDrawingChar( 'n' ); 00310 for( int8_t i = 0; i <= 64; i++ ) 00311 { 00312 vt.PutBoxDrawingChar( 'q' ); 00313 } 00314 vt.PutBoxDrawingChar( 'u' ); 00315 vt.printf( "\r\n" ); 00316 } 00317 00318 void SerialDisplayDrawLine( const char* firstCol, const char* secondCol ) 00319 { 00320 vt.PutBoxDrawingChar( 'x' ); 00321 vt.printf( "%s", firstCol ); 00322 vt.PutBoxDrawingChar( 'x' ); 00323 vt.printf( "%s", secondCol ); 00324 vt.PutBoxDrawingChar( 'x' ); 00325 vt.printf( "\r\n" ); 00326 } 00327 00328 void SerialDisplayDrawBottomLine( void ) 00329 { 00330 vt.PutBoxDrawingChar( 'm' ); 00331 for( int8_t i = 0; i <= 11; i++ ) 00332 { 00333 vt.PutBoxDrawingChar( 'q' ); 00334 } 00335 vt.PutBoxDrawingChar( 'v' ); 00336 for( int8_t i = 0; i <= 64; i++ ) 00337 { 00338 vt.PutBoxDrawingChar( 'q' ); 00339 } 00340 vt.PutBoxDrawingChar( 'j' ); 00341 vt.printf( "\r\n" ); 00342 } 00343 00344 void SerialDisplayInit( void ) 00345 { 00346 vt.ClearScreen( 2 ); 00347 vt.SetCursorMode( false ); 00348 vt.SetCursorPos( 0, 0 ); 00349 00350 // "+-----------------------------------------------------------------------------+" ); 00351 SerialDisplayDrawFirstLine( ); 00352 // "¦ LoRaWAN Demonstration Application ¦" ); 00353 SerialDisplayDrawTitle( " LoRaWAN Production Demonstration Application " ); 00354 // "+------------+----------------------------------------------------------------¦" ); 00355 SerialDisplayDrawTopSeparator( ); 00356 // "¦ Activation ¦ [ ]Over The Air ¦" ); 00357 SerialDisplayDrawLine( " Activation ", " [ ]Over The Air " ); 00358 // "¦ ¦ DevEui [__ __ __ __ __ __ __ __] ¦" ); 00359 SerialDisplayDrawLine( " ", " DevEui [__ __ __ __ __ __ __ __] " ); 00360 // "¦ ¦ AppEui [__ __ __ __ __ __ __ __] ¦" ); 00361 SerialDisplayDrawLine( " ", " AppEui [__ __ __ __ __ __ __ __] " ); 00362 // "¦ ¦ AppKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00363 SerialDisplayDrawLine( " ", " AppKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00364 // "¦ +----------------------------------------------------------------¦" ); 00365 SerialDisplayDrawColSeparator( ); 00366 // "¦ ¦ [x]Personalisation ¦" ); 00367 SerialDisplayDrawLine( " ", " [ ]Personalisation " ); 00368 // "¦ ¦ NwkId [___] ¦" ); 00369 SerialDisplayDrawLine( " ", " NwkId [___] " ); 00370 // "¦ ¦ DevAddr [__ __ __ __] ¦" ); 00371 SerialDisplayDrawLine( " ", " DevAddr [__ __ __ __] " ); 00372 // "¦ ¦ NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00373 SerialDisplayDrawLine( " ", " NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00374 // "¦ ¦ AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00375 SerialDisplayDrawLine( " ", " AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00376 // "+------------+----------------------------------------------------------------¦" ); 00377 SerialDisplayDrawSeparator( ); 00378 // "¦ MAC params ¦ [ ]Confirmed / [ ]Unconfirmed ¦" ); 00379 SerialDisplayDrawLine( " MAC params ", " [ ]Confirmed / [ ]Unconfirmed " ); 00380 // "¦ ¦ ADR [ ] ¦" ); 00381 SerialDisplayDrawLine( " ", " ADR [ ] " ); 00382 // "¦ ¦ Duty cycle[ ] ¦" ); 00383 SerialDisplayDrawLine( " ", " Duty cycle[ ] " ); 00384 // "+------------+----------------------------------------------------------------¦" ); 00385 SerialDisplayDrawSeparator( ); 00386 // "¦ Network ¦ [ ]Public / [ ]Private ¦" ); 00387 SerialDisplayDrawLine( " Network ", " [ ]Public / [ ]Private " ); 00388 // "¦ ¦ [ ]Joining / [ ]Joined ¦" ); 00389 SerialDisplayDrawLine( " ", " [ ]Joining / [ ]Joined " ); 00390 // "+------------+----------------------------------------------------------------¦" ); 00391 SerialDisplayDrawSeparator( ); 00392 // "¦ LED status ¦ [ ]LED1(Tx) / [ ]LED2(Rx) / [ ]LED3(App) ¦" ); 00393 SerialDisplayDrawLine( " LED status ", " [ ]LED1(Tx) / [ ]LED2(Rx) / [ ]LED3(App) " ); 00394 // "+------------+----------------------------------------------------------------¦" ); 00395 SerialDisplayDrawSeparator( ); 00396 // "¦ Uplink ¦ Acked [ ] ¦" ); 00397 SerialDisplayDrawLine( " Uplink ", " Acked [ ] " ); 00398 // "¦ ¦ Datarate [ ] ¦" ); 00399 SerialDisplayDrawLine( " ", " Datarate [ ] " ); 00400 // "¦ ¦ Counter [ ] ¦" ); 00401 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00402 // "¦ ¦ Port [ ] ¦" ); 00403 SerialDisplayDrawLine( " ", " Port [ ] " ); 00404 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00405 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00406 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00407 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00408 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00409 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00410 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00411 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00412 // "+------------+----------------------------------------------------------------¦" ); 00413 SerialDisplayDrawSeparator( ); 00414 // "¦ Downlink ¦ RSSI [ ] dBm ¦" ); 00415 SerialDisplayDrawLine( " Downlink ", " RSSI [ ] dBm " ); 00416 // "¦ [ ]Data ¦ SNR [ ] dB ¦" ); 00417 SerialDisplayDrawLine( " [ ]Data ", " SNR [ ] dB " ); 00418 // "¦ ¦ Counter [ ] ¦" ); 00419 // "¦ ¦ Counter [ ] ¦" ); 00420 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00421 // "¦ ¦ Port [ ] ¦" ); 00422 SerialDisplayDrawLine( " ", " Port [ ] " ); 00423 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00424 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00425 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00426 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00427 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00428 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00429 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00430 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00431 // "+------------+----------------------------------------------------------------+" ); 00432 SerialDisplayDrawBottomLine( ); 00433 vt.printf( "To refresh screen please hit 'r' key.\r\n" ); 00434 } 00435 00436 bool SerialDisplayReadable( void ) 00437 { 00438 return vt.Readable( ); 00439 } 00440 00441 uint8_t SerialDisplayGetChar( void ) 00442 { 00443 return vt.GetChar( ); 00444 } 00445 00446 void SerialDisplayMessage1(char *str) 00447 { 00448 vt.SetCursorPos( 45, 0 ); 00449 vt.printf("%s",str); 00450 } 00451 00452 void SerialDisplayMessage2(char *str) 00453 { 00454 vt.SetCursorPos( 46, 0 ); 00455 vt.printf("%s",str); 00456 }
Generated on Wed Jul 13 2022 20:47:11 by
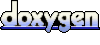