
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
wavfmt.h
00001 #ifndef WAVFMT_H 00002 #define WAVFMT_H 00003 00004 #ifdef __cplusplus 00005 extern "C" { 00006 #endif 00007 00008 00009 typedef struct { 00010 char chunkID[4]; 00011 long chunkSize; 00012 } ChunkHeader; 00013 00014 00015 #define RiffID "RIFF" /* chunk ID for RIFF Chunk */ 00016 #define RIFF_FORMAT "WAVE" 00017 00018 typedef struct { 00019 char chunkID[4]; //'RIFF' 00020 long chunkSize; 00021 char RiffFmt[4]; //'WAVE' 00022 } RiffChunk; 00023 00024 00025 00026 #define WAVE_FORMAT_PCM 0X01 00027 #define WAVE_CHANNEL_MONO 1 00028 #define WAVE_CHANNEL_STEREO 2 00029 00030 00031 00032 #define OFFSET_FMT_CHK sizeof(RiffChunk) 00033 #define FormatID "fmt " /* chunkID for Format Chunk. NOTE: There is a space at the end of this ID. */ 00034 00035 typedef struct { 00036 char chunkID[4]; 00037 long chunkSize; 00038 short wFormatTag; 00039 unsigned short wChannels; 00040 unsigned long dwSamplesPerSec; 00041 unsigned long dwAvgBytesPerSec; 00042 unsigned short wBlockAlign; 00043 unsigned short wBitsPerSample; 00044 /* Note: there may be additional fields here, depending upon wFormatTag. */ 00045 00046 } FormatChunk; 00047 00048 00049 #define OFFSET_DATA_CHK (OFFSET_FMT_CHK + sizeof(FormatChunk)) 00050 #define DataID "data" /* chunk ID for data Chunk */ 00051 00052 typedef struct { 00053 char chunkID[4]; 00054 long chunkSize; 00055 00056 unsigned char waveformData[]; 00057 } DataChunk; 00058 00059 00060 #define ListID "list" /* chunk ID for list Chunk */ 00061 00062 typedef struct { 00063 char chunkID[4]; /* 'list' */ 00064 long chunkSize; /* includes the Type ID below */ 00065 char typeID[]; /* 'adtl' */ 00066 } ListHeader; 00067 00068 00069 #define WAVE_HEADER_SIZE (OFFSET_DATA_CHK + sizeof(DataChunk)) 00070 00071 00072 int wavfmt_remove_header(FILE *pFile); 00073 int wavfmt_add_riff_chunk(FILE *pFile, long size); 00074 int wavfmt_add_fmt_chunk(FILE *pFile, unsigned short wChannels, unsigned long dwSamplesPerSec, unsigned long dwAvgBytesPerSec, unsigned short wBitsPerSample); 00075 int wavfmt_add_data_chunk_header(FILE *pFile, long size); 00076 00077 00078 #ifdef __cplusplus 00079 } 00080 #endif 00081 00082 00083 #endif
Generated on Tue Jul 12 2022 16:28:54 by
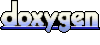