
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
vorbis_psy.h
00001 /* Copyright (C) 2005 Jean-Marc Valin, CSIRO, Christopher Montgomery 00002 File: vorbis_psy.h 00003 00004 Redistribution and use in source and binary forms, with or without 00005 modification, are permitted provided that the following conditions 00006 are met: 00007 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 00011 - Redistributions in binary form must reproduce the above copyright 00012 notice, this list of conditions and the following disclaimer in the 00013 documentation and/or other materials provided with the distribution. 00014 00015 - Neither the name of the Xiph.org Foundation nor the names of its 00016 contributors may be used to endorse or promote products derived from 00017 this software without specific prior written permission. 00018 00019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00020 ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00021 LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00022 A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE FOUNDATION OR 00023 CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00024 EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00025 PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00027 LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00028 NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 */ 00031 00032 #ifndef VORBIS_PSY_H 00033 #define VORBIS_PSY_H 00034 00035 #ifdef VORBIS_PSYCHO 00036 00037 #include "smallft.h" 00038 #define P_BANDS 17 /* 62Hz to 16kHz */ 00039 #define NOISE_COMPAND_LEVELS 40 00040 00041 00042 #define todB(x) ((x)==0?-400.f:log((x)*(x))*4.34294480f) 00043 #define fromdB(x) (exp((x)*.11512925f)) 00044 00045 /* The bark scale equations are approximations, since the original 00046 table was somewhat hand rolled. The below are chosen to have the 00047 best possible fit to the rolled tables, thus their somewhat odd 00048 appearance (these are more accurate and over a longer range than 00049 the oft-quoted bark equations found in the texts I have). The 00050 approximations are valid from 0 - 30kHz (nyquist) or so. 00051 00052 all f in Hz, z in Bark */ 00053 00054 #define toBARK(n) (13.1f*atan(.00074f*(n))+2.24f*atan((n)*(n)*1.85e-8f)+1e-4f*(n)) 00055 #define fromBARK(z) (102.f*(z)-2.f*pow(z,2.f)+.4f*pow(z,3.f)+pow(1.46f,z)-1.f) 00056 00057 /* Frequency to octave. We arbitrarily declare 63.5 Hz to be octave 00058 0.0 */ 00059 00060 #define toOC(n) (log(n)*1.442695f-5.965784f) 00061 #define fromOC(o) (exp(((o)+5.965784f)*.693147f)) 00062 00063 00064 typedef struct { 00065 00066 float noisewindowlo; 00067 float noisewindowhi; 00068 int noisewindowlomin; 00069 int noisewindowhimin; 00070 int noisewindowfixed; 00071 float noiseoff[P_BANDS]; 00072 float noisecompand[NOISE_COMPAND_LEVELS]; 00073 00074 } VorbisPsyInfo; 00075 00076 00077 00078 typedef struct { 00079 int n; 00080 int rate; 00081 struct drft_lookup lookup; 00082 VorbisPsyInfo *vi; 00083 00084 float *window; 00085 float *noiseoffset; 00086 long *bark; 00087 00088 } VorbisPsy; 00089 00090 00091 VorbisPsy *vorbis_psy_init(int rate, int size); 00092 void vorbis_psy_destroy(VorbisPsy *psy); 00093 void compute_curve(VorbisPsy *psy, float *audio, float *curve); 00094 void curve_to_lpc(VorbisPsy *psy, float *curve, float *awk1, float *awk2, int ord); 00095 00096 #endif 00097 #endif
Generated on Tue Jul 12 2022 16:28:54 by
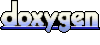