
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
verification.h
00001 00002 #ifndef _MBEDPACK_VERIFICATION_H 00003 #define _MBEDPACK_VERIFICATION_H 00004 00005 #include "mbedtls/rsa.h" 00006 #include "mbedtls/sha1.h" 00007 #include "pack_include.h" 00008 00009 typedef struct _verification_context_ { 00010 // internal footprint 00011 uint32_t stream_recieved_sz; // current size of recieved stream 00012 uint32_t stream_processed_sz; // current size of processed stream 00013 uint32_t stream_sig_stored_sz; // size of pck_header_sig_part 00014 unsigned char* pck_header_sig_part; // package header buffer to pck signature(include), for verify use 00015 00016 // sha1 context 00017 mbedtls_sha1_context* ctx; 00018 00019 } verification_context_t; 00020 00021 /** 00022 * init rsa context 00023 * 00024 * \param rsa_ctx point to an instance of mbedtls_rsa_context struct 00025 * \return 0 if success, or failed 00026 */ 00027 int mbed_rsa_ca_pkcs1_init(mbedtls_rsa_context* rsa_ctx); 00028 00029 /** 00030 * uinit rsa context 00031 * 00032 * \param rsa_ctx point to an instance of mbedtls_rsa_context struct 00033 */ 00034 void mbed_rsa_ca_pkcs1_uninit(mbedtls_rsa_context* rsa_ctx); 00035 00036 /////////////////////////////////////////////////////////////////////////////////////// 00037 00038 /** 00039 * verification init 00040 * 00041 * \return verification context if success, or NULL 00042 */ 00043 verification_context_t* mbed_hash_init(); 00044 00045 /** 00046 * verification update key 00047 * 00048 * \param ctx verification context 00049 * \param buffer data source to gen hash 00050 * \param buffer_size 00051 */ 00052 void mbed_hash_update_key(verification_context_t* ctx, unsigned char* buffer, uint32_t buffer_size); 00053 00054 /** 00055 * verify key 00056 * 00057 * \param ctx verification context 00058 * \param buffer data source to gen hash 00059 * \param buffer_size 00060 * 00061 * \return 0 if success, or failed 00062 */ 00063 int mbed_rsa_ca_pkcs1_verify(mbedtls_rsa_context* rsa, verification_context_t* ctx); 00064 00065 /** 00066 * verification uninit 00067 * 00068 * \param ctx verification context 00069 */ 00070 void mbed_hash_uninit(verification_context_t* ctx); 00071 00072 #endif 00073
Generated on Tue Jul 12 2022 16:28:54 by
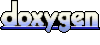