
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
speex_jitter.h
Go to the documentation of this file.
00001 /* Copyright (C) 2002 Jean-Marc Valin */ 00002 /** 00003 @file speex_jitter.h 00004 @brief Adaptive jitter buffer for Speex 00005 */ 00006 /* 00007 Redistribution and use in source and binary forms, with or without 00008 modification, are permitted provided that the following conditions 00009 are met: 00010 00011 - Redistributions of source code must retain the above copyright 00012 notice, this list of conditions and the following disclaimer. 00013 00014 - Redistributions in binary form must reproduce the above copyright 00015 notice, this list of conditions and the following disclaimer in the 00016 documentation and/or other materials provided with the distribution. 00017 00018 - Neither the name of the Xiph.org Foundation nor the names of its 00019 contributors may be used to endorse or promote products derived from 00020 this software without specific prior written permission. 00021 00022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE FOUNDATION OR 00026 CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00027 EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00028 PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00030 LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00031 NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00032 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 00034 */ 00035 00036 #ifndef SPEEX_JITTER_H 00037 #define SPEEX_JITTER_H 00038 /** @defgroup JitterBuffer JitterBuffer: Adaptive jitter buffer 00039 * This is the jitter buffer that reorders UDP/RTP packets and adjusts the buffer size 00040 * to maintain good quality and low latency. 00041 * @{ 00042 */ 00043 00044 #include "speex.h" 00045 #include "speex_bits.h" 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 /** Generic adaptive jitter buffer state */ 00052 struct JitterBuffer_; 00053 00054 /** Generic adaptive jitter buffer state */ 00055 typedef struct JitterBuffer_ JitterBuffer; 00056 00057 /** Definition of an incoming packet */ 00058 typedef struct _JitterBufferPacket JitterBufferPacket; 00059 00060 /** Definition of an incoming packet */ 00061 struct _JitterBufferPacket { 00062 char *data; /**< Data bytes contained in the packet */ 00063 spx_uint32_t len; /**< Length of the packet in bytes */ 00064 spx_uint32_t timestamp; /**< Timestamp for the packet */ 00065 spx_uint32_t span; /**< Time covered by the packet (same units as timestamp) */ 00066 }; 00067 00068 /** Packet has been retrieved */ 00069 #define JITTER_BUFFER_OK 0 00070 /** Packet is missing */ 00071 #define JITTER_BUFFER_MISSING 1 00072 /** Packet is incomplete (does not cover the entive tick */ 00073 #define JITTER_BUFFER_INCOMPLETE 2 00074 /** There was an error in the jitter buffer */ 00075 #define JITTER_BUFFER_INTERNAL_ERROR -1 00076 /** Invalid argument */ 00077 #define JITTER_BUFFER_BAD_ARGUMENT -2 00078 00079 00080 /** Set minimum amount of extra buffering required (margin) */ 00081 #define JITTER_BUFFER_SET_MARGIN 0 00082 /** Get minimum amount of extra buffering required (margin) */ 00083 #define JITTER_BUFFER_GET_MARGIN 1 00084 /* JITTER_BUFFER_SET_AVALIABLE_COUNT wouldn't make sense */ 00085 /** Get the amount of avaliable packets currently buffered */ 00086 #define JITTER_BUFFER_GET_AVALIABLE_COUNT 3 00087 00088 #define JITTER_BUFFER_ADJUST_INTERPOLATE -1 00089 #define JITTER_BUFFER_ADJUST_OK 0 00090 #define JITTER_BUFFER_ADJUST_DROP 1 00091 00092 /** Initialises jitter buffer 00093 * 00094 * @param tick Number of samples per "tick", i.e. the time period of the elements that will be retrieved 00095 * @return Newly created jitter buffer state 00096 */ 00097 JitterBuffer *jitter_buffer_init(int tick); 00098 00099 /** Restores jitter buffer to its original state 00100 * 00101 * @param jitter Jitter buffer state 00102 */ 00103 void jitter_buffer_reset(JitterBuffer *jitter); 00104 00105 /** Destroys jitter buffer 00106 * 00107 * @param jitter Jitter buffer state 00108 */ 00109 void jitter_buffer_destroy(JitterBuffer *jitter); 00110 00111 /** Put one packet into the jitter buffer 00112 * 00113 * @param jitter Jitter buffer state 00114 * @param packet Incoming packet 00115 */ 00116 void jitter_buffer_put(JitterBuffer *jitter, const JitterBufferPacket *packet); 00117 00118 /** Get one packet from the jitter buffer 00119 * 00120 * @param jitter Jitter buffer state 00121 * @param packet Returned packet 00122 * @param current_timestamp Timestamp for the returned packet 00123 */ 00124 int jitter_buffer_get(JitterBuffer *jitter, JitterBufferPacket *packet, spx_int32_t *start_offset); 00125 00126 /** Get pointer timestamp of jitter buffer 00127 * 00128 * @param jitter Jitter buffer state 00129 */ 00130 int jitter_buffer_get_pointer_timestamp(JitterBuffer *jitter); 00131 00132 /** Advance by one tick 00133 * 00134 * @param jitter Jitter buffer state 00135 */ 00136 void jitter_buffer_tick(JitterBuffer *jitter); 00137 00138 /** Used like the ioctl function to control the jitter buffer parameters 00139 * 00140 * @param jitter Jitter buffer state 00141 * @param request ioctl-type request (one of the JITTER_BUFFER_* macros) 00142 * @param ptr Data exchanged to-from function 00143 * @return 0 if no error, -1 if request in unknown 00144 */ 00145 int jitter_buffer_ctl(JitterBuffer *jitter, int request, void *ptr); 00146 00147 int jitter_buffer_update_delay(JitterBuffer *jitter, JitterBufferPacket *packet, spx_int32_t *start_offset); 00148 00149 /* @} */ 00150 00151 /** @defgroup SpeexJitter SpeexJitter: Adaptive jitter buffer specifically for Speex 00152 * This is the jitter buffer that reorders UDP/RTP packets and adjusts the buffer size 00153 * to maintain good quality and low latency. This is a simplified version that works only 00154 * with Speex, but is much easier to use. 00155 * @{ 00156 */ 00157 00158 /** Speex jitter-buffer state. Never use it directly! */ 00159 typedef struct SpeexJitter { 00160 SpeexBits current_packet; /**< Current Speex packet */ 00161 int valid_bits; /**< True if Speex bits are valid */ 00162 JitterBuffer *packets; /**< Generic jitter buffer state */ 00163 void *dec; /**< Pointer to Speex decoder */ 00164 spx_int32_t frame_size; /**< Frame size of Speex decoder */ 00165 } SpeexJitter; 00166 00167 /** Initialise jitter buffer 00168 * 00169 * @param jitter State of the Speex jitter buffer 00170 * @param decoder Speex decoder to call 00171 * @param sampling_rate Sampling rate used by the decoder 00172 */ 00173 void speex_jitter_init(SpeexJitter *jitter, void *decoder, int sampling_rate); 00174 00175 /** Destroy jitter buffer */ 00176 void speex_jitter_destroy(SpeexJitter *jitter); 00177 00178 /** Put one packet into the jitter buffer */ 00179 void speex_jitter_put(SpeexJitter *jitter, char *packet, int len, int timestamp); 00180 00181 /** Get one packet from the jitter buffer */ 00182 void speex_jitter_get(SpeexJitter *jitter, spx_int16_t *out, int *start_offset); 00183 00184 /** Get pointer timestamp of jitter buffer */ 00185 int speex_jitter_get_pointer_timestamp(SpeexJitter *jitter); 00186 00187 #ifdef __cplusplus 00188 } 00189 #endif 00190 00191 /* @} */ 00192 #endif
Generated on Tue Jul 12 2022 16:28:54 by
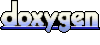