
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
mp4ff.h
00001 /* 00002 ** FAAD2 - Freeware Advanced Audio (AAC) Decoder including SBR decoding 00003 ** Copyright (C) 2003-2005 M. Bakker, Nero AG, http://www.nero.com 00004 ** 00005 ** This program is free software; you can redistribute it and/or modify 00006 ** it under the terms of the GNU General Public License as published by 00007 ** the Free Software Foundation; either version 2 of the License, or 00008 ** (at your option) any later version. 00009 ** 00010 ** This program is distributed in the hope that it will be useful, 00011 ** but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 ** MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 ** GNU General Public License for more details. 00014 ** 00015 ** You should have received a copy of the GNU General Public License 00016 ** along with this program; if not, write to the Free Software 00017 ** Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA. 00018 ** 00019 ** Any non-GPL usage of this software or parts of this software is strictly 00020 ** forbidden. 00021 ** 00022 ** The "appropriate copyright message" mentioned in section 2c of the GPLv2 00023 ** must read: "Code from FAAD2 is copyright (c) Nero AG, www.nero.com" 00024 ** 00025 ** Commercial non-GPL licensing of this software is possible. 00026 ** For more info contact Nero AG through Mpeg4AAClicense@nero.com. 00027 ** 00028 ** $Id: mp4ff.h,v 1.27 2009/01/29 00:41:08 menno Exp $ 00029 **/ 00030 00031 #ifndef MP4FF_H 00032 #define MP4FF_H 00033 00034 #include "mp4ffint.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif /* __cplusplus */ 00039 #define HAVE_STDINT_H 00040 #ifdef HAVE_STDINT_H 00041 #include <stdint.h> 00042 #else 00043 #include "mp4ff_int_types.h" 00044 #endif 00045 00046 #if 0 00047 /* file callback structure */ 00048 typedef struct 00049 { 00050 uint32_t (*read)(void *user_data, void *buffer, uint32_t length); 00051 uint32_t (*write)(void *udata, void *buffer, uint32_t length); 00052 uint32_t (*seek)(void *user_data, uint64_t position); 00053 uint32_t (*truncate)(void *user_data); 00054 void *user_data; 00055 } mp4ff_callback_t; 00056 00057 /* mp4 main file structure */ 00058 typedef void* mp4ff_t; 00059 #endif 00060 00061 /* API */ 00062 00063 mp4ff_t *mp4ff_open_read(mp4ff_callback_t *f); 00064 mp4ff_t *mp4ff_open_read_metaonly(mp4ff_callback_t *f); 00065 void mp4ff_close(mp4ff_t *f); 00066 int32_t mp4ff_get_sample_duration(const mp4ff_t *f, const int32_t track, const int32_t sample); 00067 int32_t mp4ff_get_sample_duration_use_offsets(const mp4ff_t *f, const int32_t track, const int32_t sample); 00068 int64_t mp4ff_get_sample_position(const mp4ff_t *f, const int32_t track, const int32_t sample); 00069 int32_t mp4ff_get_sample_offset(const mp4ff_t *f, const int32_t track, const int32_t sample); 00070 int32_t mp4ff_find_sample(const mp4ff_t *f, const int32_t track, const int64_t offset,int32_t * toskip); 00071 int32_t mp4ff_find_sample_use_offsets(const mp4ff_t *f, const int32_t track, const int64_t offset,int32_t * toskip); 00072 00073 int32_t mp4ff_read_sample(mp4ff_t *f, const int track, const int sample, 00074 unsigned char **audio_buffer, unsigned int *bytes); 00075 00076 int32_t mp4ff_set_position(mp4ff_t *f, const int64_t position); 00077 00078 int32_t rda_mp4ff_read_sample(mp4ff_t *f, const int32_t track, const int32_t sample, 00079 uint8_t **audio_buffer, uint32_t *bytes);//add by bolv 00080 int32_t rda_mp4ff_read_sample_adts(mp4ff_t *f, const int32_t track, const int32_t sample, 00081 uint8_t **audio_buffer, uint32_t *bytes);//add by bolv 00082 int32_t mp4ff_read_sample_v2(mp4ff_t *f, const int track, const int sample,unsigned char *buffer);//returns 0 on error, number of bytes read on success, use mp4ff_read_sample_getsize() to check buffer size needed 00083 int32_t mp4ff_read_sample_getsize(mp4ff_t *f, const int track, const int sample);//returns 0 on error, buffer size needed for mp4ff_read_sample_v2() on success 00084 int32_t rda_mp4ff_fill_stsz_table(mp4ff_t *f, const int32_t track, const int32_t sample);//add by bolv 00085 int32_t rda_mp4ff_refill_stsz_table(mp4ff_t *f, const int32_t track, const int32_t sample, const int32_t numSamples);//add by bolv 00086 int32_t rda_mp4ff_get_sample_position_and_size(mp4ff_t *f, const int32_t track, const int32_t sample, int32_t *offset, int32_t *size);//add by bolv 00087 00088 int32_t mp4ff_get_decoder_config(const mp4ff_t *f, const int track, 00089 unsigned char** ppBuf, unsigned int* pBufSize); 00090 int32_t mp4ff_get_track_type(const mp4ff_t *f, const int track); 00091 int32_t mp4ff_total_tracks(const mp4ff_t *f); 00092 int32_t rda_mp4ff_get_aac_track(const mp4ff_t *f);//add by bolv 00093 int32_t mp4ff_num_samples(const mp4ff_t *f, const int track); 00094 int32_t mp4ff_time_scale(const mp4ff_t *f, const int track); 00095 00096 uint32_t mp4ff_get_avg_bitrate(const mp4ff_t *f, const int32_t track); 00097 uint32_t mp4ff_get_max_bitrate(const mp4ff_t *f, const int32_t track); 00098 int64_t mp4ff_get_track_duration(const mp4ff_t *f, const int32_t track); //returns (-1) if unknown 00099 int64_t mp4ff_get_track_duration_use_offsets(const mp4ff_t *f, const int32_t track); //returns (-1) if unknown 00100 uint32_t mp4ff_get_sample_rate(const mp4ff_t *f, const int32_t track); 00101 uint32_t mp4ff_get_channel_count(const mp4ff_t * f,const int32_t track); 00102 uint32_t mp4ff_get_audio_type(const mp4ff_t * f,const int32_t track); 00103 00104 00105 /* metadata */ 00106 int mp4ff_meta_get_num_items(const mp4ff_t *f); 00107 int mp4ff_meta_get_by_index(const mp4ff_t *f, unsigned int index, 00108 char **item, char **value); 00109 int mp4ff_meta_get_title(const mp4ff_t *f, char **value); 00110 int mp4ff_meta_get_artist(const mp4ff_t *f, char **value); 00111 int mp4ff_meta_get_writer(const mp4ff_t *f, char **value); 00112 int mp4ff_meta_get_album(const mp4ff_t *f, char **value); 00113 int mp4ff_meta_get_date(const mp4ff_t *f, char **value); 00114 int mp4ff_meta_get_tool(const mp4ff_t *f, char **value); 00115 int mp4ff_meta_get_comment(const mp4ff_t *f, char **value); 00116 int mp4ff_meta_get_genre(const mp4ff_t *f, char **value); 00117 int mp4ff_meta_get_track(const mp4ff_t *f, char **value); 00118 int mp4ff_meta_get_disc(const mp4ff_t *f, char **value); 00119 int mp4ff_meta_get_totaltracks(const mp4ff_t *f, char **value); 00120 int mp4ff_meta_get_totaldiscs(const mp4ff_t *f, char **value); 00121 int mp4ff_meta_get_compilation(const mp4ff_t *f, char **value); 00122 int mp4ff_meta_get_tempo(const mp4ff_t *f, char **value); 00123 int32_t mp4ff_meta_get_coverart(const mp4ff_t *f, char **value); 00124 #ifdef USE_TAGGING 00125 00126 /* metadata tag structure */ 00127 typedef struct 00128 { 00129 char *item; 00130 char *value; 00131 } mp4ff_tag_t; 00132 00133 /* metadata list structure */ 00134 typedef struct 00135 { 00136 mp4ff_tag_t *tags; 00137 uint32_t count; 00138 } mp4ff_metadata_t; 00139 00140 int32_t mp4ff_meta_update(mp4ff_callback_t *f,const mp4ff_metadata_t * data); 00141 00142 #endif 00143 00144 00145 #ifdef __cplusplus 00146 } 00147 #endif /* __cplusplus */ 00148 00149 #endif
Generated on Tue Jul 12 2022 16:28:53 by
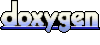